class balanced loss
时间: 2023-05-02 13:00:41 浏览: 55
b'class平衡损失'指的是一种用于解决训练样本类别不平衡问题的损失函数。通过对不同类别的样本进行加权,使得模型的训练更加平衡。在分类问题中,存在某些类别样本数量较少,而某些类别样本数量较多的情况下,使用b'class平衡损失'可以提高分类算法的准确性和鲁棒性。
相关问题
Class-Balanced focal loss代码实现
以下一个简单的代码实现,用于计算类别平衡的focal loss:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class ClassBalancedFocalLoss(nn.Module):
def __init__(self, gamma=2, alpha=None, size_average=True):
super(ClassBalancedFocalLoss, self).__init__()
self.gamma = gamma
self.alpha = alpha
if self.alpha is None:
self.alpha = torch.ones(1, requires_grad=True)
self.size_average = size_average
def forward(self, inputs, targets):
# 计算分类损失
ce_loss = F.cross_entropy(inputs, targets, reduction='none')
pt = torch.exp(-ce_loss)
# 计算类别平衡权重
class_weights = self.alpha / (torch.sum(self.alpha) + 1e-10)
weights = torch.pow(1 - pt, self.gamma) * class_weights
# 计算最终的focal loss
balanced_focal_loss = ce_loss * weights
if self.size_average:
balanced_focal_loss = torch.mean(balanced_focal_loss)
return balanced_focal_loss
```
这里的`gamma`是focal loss中的调节因子,`alpha`是每个类别的权重,如果不提供`alpha`,则默认所有类别的权重相等。`inputs`是模型的输出,`targets`是真实的类别标签。
你可以根据需要将其用于你的训练过程中,例如:
```python
loss_criterion = ClassBalancedFocalLoss(gamma=2, alpha=None)
outputs = model(inputs)
loss = loss_criterion(outputs, targets)
```
请注意,这只是一个简单的实现,实际应用中可能需要根据具体情况进行修改和调整。
batch-balanced contrastive loss的pytorch代码
以下是一个batch-balanced contrastive loss的PyTorch代码示例:
```python
import torch
import torch.nn.functional as F
class BatchBalancedContrastiveLoss(torch.nn.Module):
def __init__(self, margin=1.0, batch_size=256, num_classes=10):
super(BatchBalancedContrastiveLoss, self).__init__()
self.margin = margin
self.batch_size = batch_size
self.num_classes = num_classes
def forward(self, features, labels):
# Compute pairwise cosine similarity
similarity_matrix = F.cosine_similarity(features.unsqueeze(1), features.unsqueeze(0), dim=2)
# Create mask for positive pairs (same class)
mask_pos = torch.zeros_like(similarity_matrix)
for i in range(self.batch_size):
mask_pos[i*self.num_classes:(i+1)*self.num_classes, i*self.num_classes:(i+1)*self.num_classes] = 1
mask_pos = mask_pos.to(features.device)
# Create mask for negative pairs (different class)
mask_neg = 1 - mask_pos
# Compute losses for positive and negative pairs
loss_pos = torch.sum(mask_pos * (1 - similarity_matrix)**2)
loss_neg = torch.sum(mask_neg * torch.relu(similarity_matrix - self.margin)**2)
# Compute the final loss by balancing the positive and negative losses
num_pos = torch.sum(mask_pos).item()
num_neg = torch.sum(mask_neg).item()
beta = num_neg / (num_pos + num_neg)
loss = beta * loss_pos + (1 - beta) * loss_neg
return loss
```
在这个例子中,我们创建了一个`BatchBalancedContrastiveLoss`类,它继承自`torch.nn.Module`。`margin`参数是对比损失函数中的间隔参数,`batch_size`参数是每个batch中的样本数量,`num_classes`参数是每个类别的样本数。
在`forward`方法中,我们首先使用`F.cosine_similarity`函数计算特征向量之间的余弦相似度矩阵。我们然后创建了一个`mask_pos`变量,它是一个大小为(batch_size*num_classes, batch_size*num_classes)的零矩阵,其中对角线上的元素为1,代表同类别样本之间的相似度。我们还创建了一个`mask_neg`变量,它是`mask_pos`的补集,代表不同类别样本之间的相似度。
接下来,我们计算正样本对和负样本对的损失。对于正样本对,我们使用(1-相似度)^2计算损失。对于负样本对,我们使用max(0, 相似度-间隔)^2计算损失。
最后,我们计算平衡的对比损失,通过计算正样本对和负样本对的损失之间的权衡。我们使用beta=num_neg/(num_pos+num_neg)来计算负样本在损失函数中所占的比例,其中num_pos和num_neg分别是正样本对和负样本对的数量。
这个代码示例可以用于训练具有类别标签的对比学习模型,例如具有训练集中的类别标签的图像数据集。
相关推荐
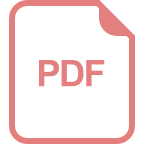
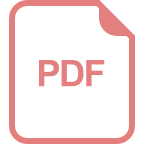
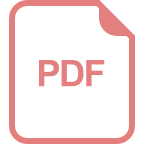












