To rotate a letter (lowercase) means to shift it through the alphabet, wrapping around to the beginning if necessary, so ‘a’ rotated by 3 is ‘d’ and ‘a’ rotated by -1 is ‘z’. To rotate a word (all letters are lowercase), rotate each letter by the same amount. For example, “cheer” rotated by 7 is “jolly” and “melon” rotated by -10 is “cubed”. You might want to use the built-in function ord, which converts a character to a integer code, and chr, which converts integer codes to characters. Letters of the alphabet are encoded in alphabetical order, so for example: ord('c') - ord('a') == 2. Because 'c' is the two-eth letter of the alphabet. Input A string and an integer. Output A new string. Example Input Copy Output1 Copy cheer 7 jolly Input Copy Output2 Copy melon -10 cubed Input Copy Output3 Copy peace 10 zokmo
时间: 2024-01-12 17:02:35 浏览: 32
Sure, here's the updated code for the program to rotate a lowercase string by a certain amount:
```python
def rotate_word(word, rotation):
new_word = ""
for letter in word:
new_letter = chr(((ord(letter) - ord('a') + rotation) % 26) + ord('a'))
new_word += new_letter
return new_word
# Example usage
print(rotate_word("cheer", 7)) # Output: "jolly"
print(rotate_word("melon", -10)) # Output: "cubed"
print(rotate_word("peace", 10)) # Output: "zokmo"
```
In this code, we define a `rotate_word` function that takes two arguments: a lowercase string `word` and an integer `rotation`. We use a `for` loop to iterate over each letter in the word and calculate its new position in the alphabet by adding the rotation to its current position in the alphabet and wrapping around to the beginning if necessary using the `%` operator. We then convert the new position back to a letter using the `chr` function and add it to a new string `new_word`. Finally, we return the new string.
In the example usage, we call the `rotate_word` function with the input strings and rotations and print out the resulting rotated strings.
相关推荐
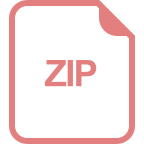
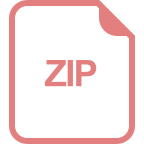














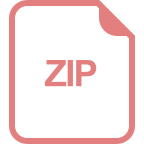