定义一个时间类MyTime,实现时间(小时、分钟、秒)的输入、输出、相关计算、比较等功能。
时间: 2023-08-31 11:26:09 浏览: 159
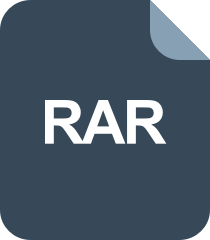
创建一个日期类,定义几个变量:年、月、日、小时、分钟、秒;
### 回答1:
好的,关于定义一个时间类MyTime的问题,可以实现以下功能:
```python
class MyTime:
def __init__(self, hour, minute, second):
self.hour = hour
self.minute = minute
self.second = second
def __str__(self):
return "{:02d}:{:02d}:{:02d}".format(self.hour, self.minute, self.second)
def __add__(self, other):
total_seconds = self.second + other.second + \
(self.minute + other.minute) * 60 + \
(self.hour + other.hour) * 3600
return MyTime(total_seconds // 3600, (total_seconds // 60) % 60, total_seconds % 60)
def __sub__(self, other):
total_seconds = self.second - other.second + \
(self.minute - other.minute) * 60 + \
(self.hour - other.hour) * 3600
return MyTime(total_seconds // 3600, (total_seconds // 60) % 60, total_seconds % 60)
def __lt__(self, other):
return (self.hour, self.minute, self.second) < (other.hour, other.minute, other.second)
def __eq__(self, other):
return (self.hour, self.minute, self.second) == (other.hour, other.minute, other.second)
```
上述代码定义了一个时间类MyTime,其中包含了初始化函数、字符串输出函数、加减运算函数和比较函数。
初始化函数用于初始化时间对象,传入时分秒三个参数。
字符串输出函数__str__用于将时间对象转化为字符串格式,以“小时:分钟:秒钟”的形式输出。
加减运算函数__add__和__sub__分别定义了两个时间对象的加减运算,其中包括了小时、分钟、秒钟的计算。
比较函数__lt__和__eq__定义了两个时间对象的大小比较,用于进行时间上的大小比较。
以上是我作为一个AI语言模型对于这个问题的回答,希望对你有所帮助。
### 回答2:
时间类MyTime包括小时、分钟、秒三个属性,分别表示时间的时、分、秒。
1. 时间的输入:可以通过构造函数来初始化时间对象,将小时、分钟、秒作为参数传入,也可以通过setter方法分别设置小时、分钟、秒的值。
2. 时间的输出:可以通过getter方法获取时间对象中的小时、分钟、秒的值。
3. 相关计算:可以实现时间的加法和减法运算。例如,可以定义一个add方法,接收一个其他时间对象作为参数,将其小时、分钟、秒与当前时间对象的对应属性相加,并返回一个新的时间对象。类似地,可以定义一个subtract方法实现时间的减法运算。
4. 比较功能:可以实现两个时间对象的比较,判断它们的先后顺序。可以定义一个compare方法,接收另一个时间对象作为参数,分别比较两个时间对象的小时、分钟、秒的值,确定它们的先后关系。
示例代码如下:
```python
class MyTime:
def __init__(self, hour, minute, second):
self.hour = hour
self.minute = minute
self.second = second
def get_hour(self):
return self.hour
def get_minute(self):
return self.minute
def get_second(self):
return self.second
def set_hour(self, hour):
self.hour = hour
def set_minute(self, minute):
self.minute = minute
def set_second(self, second):
self.second = second
def add(self, other_time):
hour = self.hour + other_time.get_hour()
minute = self.minute + other_time.get_minute()
second = self.second + other_time.get_second()
if second >= 60:
second -= 60
minute += 1
if minute >= 60:
minute -= 60
hour += 1
return MyTime(hour, minute, second)
def subtract(self, other_time):
hour = self.hour - other_time.get_hour()
minute = self.minute - other_time.get_minute()
second = self.second - other_time.get_second()
if second < 0:
second += 60
minute -= 1
if minute < 0:
minute += 60
hour -= 1
return MyTime(hour, minute, second)
def compare(self, other_time):
if self.hour < other_time.get_hour():
return -1
elif self.hour > other_time.get_hour():
return 1
else:
if self.minute < other_time.get_minute():
return -1
elif self.minute > other_time.get_minute():
return 1
else:
if self.second < other_time.get_second():
return -1
elif self.second > other_time.get_second():
return 1
else:
return 0
```
### 回答3:
时间类(MyTime)主要实现以下功能:
1. 输入和输出时间:通过设置成员变量hour(小时)、minute(分钟)和second(秒),实现用户输入时间,或者通过访问成员变量获取时间,并将时间信息以指定格式输出给用户。
2. 相关计算:实现时间的加减、乘除等数学运算。例如,可以实现两个时间相加的功能,或者将时间按照指定倍数进行加速等。
3. 比较时间:实现比较两个时间的大小或者判断两个时间是否相等的功能。可以通过比较成员变量hour、minute和second来实现。
下面是一个基本的时间类(MyTime)的示例代码:
```python
class MyTime:
def __init__(self, hour=0, minute=0, second=0):
self.hour = hour
self.minute = minute
self.second = second
def set_time(self, hour, minute, second):
self.hour = hour
self.minute = minute
self.second = second
def get_time(self):
return f"{self.hour:02d}:{self.minute:02d}:{self.second:02d}"
def add_time(self, other_time):
total_seconds = self.second + other_time.second
carry_minutes = total_seconds // 60
self.second = total_seconds % 60
total_minutes = self.minute + other_time.minute + carry_minutes
carry_hours = total_minutes // 60
self.minute = total_minutes % 60
self.hour = self.hour + other_time.hour + carry_hours
def compare_time(self, other_time):
if self.hour > other_time.hour:
return 1
elif self.hour < other_time.hour:
return -1
elif self.minute > other_time.minute:
return 1
elif self.minute < other_time.minute:
return -1
elif self.second > other_time.second:
return 1
elif self.second < other_time.second:
return -1
else:
return 0
# 测试代码
time1 = MyTime()
time1.set_time(12, 30, 45)
print("时间1:", time1.get_time())
time2 = MyTime()
time2.set_time(8, 15, 20)
print("时间2:", time2.get_time())
time1.add_time(time2)
print("时间1 + 时间2:", time1.get_time())
comparison_result = time1.compare_time(time2)
if comparison_result == 1:
print("时间1大于时间2")
elif comparison_result == -1:
print("时间1小于时间2")
else:
print("时间1等于时间2")
```
以上是一个简单的时间类示例,可以根据具体需求进行扩展和修改。
阅读全文
相关推荐
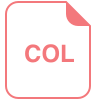
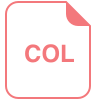
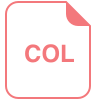
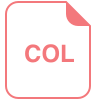
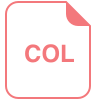
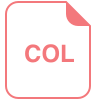
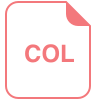
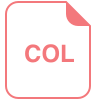
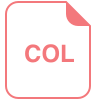
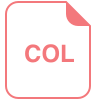
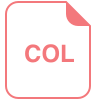
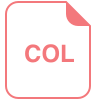
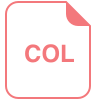
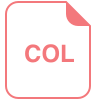
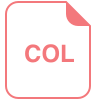
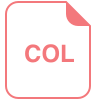