图像隐写系统中用python对输入框数据实现rsa加密
时间: 2023-06-19 22:10:54 浏览: 119
实现RSA加密需要用到Python中的`rsa`库,可以通过pip进行安装。以下是一个简单的图像隐写系统中对输入框数据进行RSA加密的例子:
```python
import rsa
# 生成RSA公私钥对
(public_key, private_key) = rsa.newkeys(512)
# 将公钥保存到文件
with open('public_key.pem', mode='wb') as f:
f.write(public_key.save_pkcs1())
# 读取公钥文件
with open('public_key.pem', mode='rb') as f:
key_data = f.read()
public_key = rsa.PublicKey.load_pkcs1(key_data)
# 对输入框数据进行加密
message = 'Hello, world!'
message = message.encode('utf8')
ciphertext = rsa.encrypt(message, public_key)
print(ciphertext)
```
首先,通过`rsa.newkeys()`函数生成RSA公私钥对。然后,将公钥保存到文件中,以备后续使用。接着,读取公钥文件,并解析出公钥对象。最后,对输入框数据进行加密,得到密文,并输出到控制台。
相关问题
python编程实现DES与RSA加密算法
在Python中,你可以使用`pycryptodome`库来实现DES(Data Encryption Standard)和RSA(Rivest-Shamir-Adleman)这两种常见的加密算法。
**DES(数据加密标准)**:
DES是一种块加密算法,它将明文分成64位的数据块,然后使用56位密钥进行加密。Python通过`Crypto.Cipher.DES`模块来操作DES。首先,你需要生成一个DES密码对象,然后设置模式(如ECB、CBC等),接着对输入数据进行加密或解密:
```python
from Crypto.Cipher import DES
key = b'your_des_key' # 8字节长度的密钥
cipher = DES.new(key, DES.MODE_ECB) # 使用ECB模式
ciphertext = cipher.encrypt(b'plaintext') # 加密
decrypted_text = cipher.decrypt(ciphertext) # 解密
```
**RSA(公钥加密算法)**:
RSA是一种非对称加密算法,涉及到两个密钥:公钥和私钥。公钥用于加密,私钥用于解密。Python中的`Crypto.PublicKey.RSA`模块提供了RSA的支持:
```python
from Crypto.PublicKey import RSA
from Crypto.Cipher import PKCS1_OAEP
# 生成RSA密钥对
key = RSA.generate(2048)
# 公钥用于加密,私钥用于解密
public_key = key.publickey()
private_key = key
# 对数据进行RSA加密
cipher_rsa = PKCS1_OAEP.new(public_key)
encrypted_data = cipher_rsa.encrypt(b'plaintext')
# 对数据进行RSA解密
cipher_rsa_dec = PKCS1_OAEP.new(private_key)
decrypted_data = cipher_rsa_dec.decrypt(encrypted_data)
```
使用python中RSA对明文数据加密
在Python中,可以使用`cryptography`库来进行RSA加密,这是一个强大的密码学工具包。以下是使用RSA进行加密的基本步骤:
1. **安装库**:
首先需要安装`cryptography`库,如果还没有安装,可以通过pip进行安装:
```
pip install cryptography
```
2. **生成密钥对**:
```python
from cryptography.hazmat.primitives import serialization
from cryptography.hazmat.primitives.asymmetric import rsa
# 密钥长度通常选择2048位或更长
key = rsa.generate_private_key(public_exponent=65537, key_size=2048)
private_key = key.private_bytes(
encoding=serialization.Encoding.PEM,
format=serialization.PrivateFormat.PKCS8,
encryption_algorithm=serialization.NoEncryption()
)
public_key = key.public_key().public_bytes(
encoding=serialization.Encoding.PEM,
format=serialization.PublicFormat.SubjectPublicKeyInfo
)
```
3. **加载公钥进行加密**:
对明文数据使用接收者的公钥进行加密:
```python
plaintext = b"Hello, world!"
encrypted_text = public_key.encrypt(plaintext, padding.OAEP(mgf=padding.MGF1(algorithm=hashes.SHA256()), algorithm=hashes.SHA256(), label=None))
```
4. **解密密文**:
使用私钥才能解密密文:
```python
decrypted_text = private_key.decrypt(encrypted_text, padding.OAEP(mgf=padding.MGF1(algorithm=hashes.SHA256()), algorithm=hashes.SHA256(), label=None)).decode()
print(decrypted_text) # 输出原始明文
```
阅读全文
相关推荐
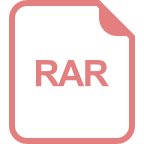
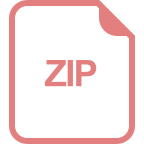
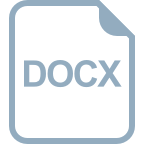













