条件1.使用stm32f103c8t6,c语言编程 条件2.使用PA0,PA1,PA2,PA3,PA4,PA5。配置成上拉输入,根据6个引脚的电平高低生成二进制数。例如000000表示6引脚电平全为0;每30秒记录一次二进制数,并统计一次0的出现次数count。并使用串口1,发送count值和二进制数给PC端。 条件3.串口1使用中断接收数据,结束符0X0D,0X0A. 条件4.串口1不接收数据默认count2==0,则备份count为count1,当条件2再次运行后得到新的count与count1比较:若count1==count,则串口打印设备数量正常;若count1>count,串口打印设备数量异常; 若count1<count,则将count赋值给count1,串口打印设备数量增加; 条件5.串口1接收数据且count2!=0,备份count2,条件2再次运行后得到新的count与count2比较,若count2==count,则串口拿取数量正确;若count2>count,串口打印拿少了; 若count2<count,串口打印拿多了;
时间: 2023-05-18 16:03:38 浏览: 90
以下是符合条件的C语言代码:
#include "stm32f10x.h"
#include "stdio.h"
#define BUFFER_SIZE 50
volatile uint8_t count1 = 0;
volatile uint8_t count2 = 0;
volatile uint8_t binary = 0;
char buffer[BUFFER_SIZE];
uint8_t buffer_index = 0;
uint8_t receive_complete = 0;
void USART1_IRQHandler(void)
{
if (USART_GetITStatus(USART1, USART_IT_RXNE) != RESET)
{
char data = USART_ReceiveData(USART1);
if (data == '\r' || data == '\n')
{
buffer[buffer_index] = '\0';
receive_complete = 1;
buffer_index = 0;
}
else
{
buffer[buffer_index++] = data;
}
}
}
void GPIO_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2 | GPIO_Pin_3 | GPIO_Pin_4 | GPIO_Pin_5;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(GPIOA, &GPIO_InitStructure);
}
void USART1_Configuration(void)
{
USART_InitTypeDef USART_InitStructure;
NVIC_InitTypeDef NVIC_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
USART_ITConfig(USART1, USART_IT_RXNE, ENABLE);
NVIC_InitStructure.NVIC_IRQChannel = USART1_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
USART_Cmd(USART1, ENABLE);
}
void TIM2_Configuration(void)
{
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
NVIC_InitTypeDef NVIC_InitStructure;
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM2, ENABLE);
TIM_TimeBaseStructure.TIM_Period = 29999;
TIM_TimeBaseStructure.TIM_Prescaler = 7199;
TIM_TimeBaseStructure.TIM_ClockDivision = TIM_CKD_DIV1;
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseInit(TIM2, &TIM_TimeBaseStructure);
TIM_ITConfig(TIM2, TIM_IT_Update, ENABLE);
NVIC_InitStructure.NVIC_IRQChannel = TIM2_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
TIM_Cmd(TIM2, ENABLE);
}
void TIM2_IRQHandler(void)
{
if (TIM_GetITStatus(TIM2, TIM_IT_Update) != RESET)
{
uint8_t new_binary = 0;
new_binary |= GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_0) << 0;
new_binary |= GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_1) << 1;
new_binary |= GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_2) << 2;
new_binary |= GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_3) << 3;
new_binary |= GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_4) << 4;
new_binary |= GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_5) << 5;
if (new_binary == 0)
{
count1++;
}
if (count2 == 0)
{
count1 = count1;
}
else
{
if (count1 == count2)
{
printf("Device quantity is normal.\r\n");
}
else if (count1 > count2)
{
printf("Device quantity is abnormal.\r\n");
}
else
{
count1 = count;
printf("Device quantity increased.\r\n");
}
}
binary = new_binary;
TIM_ClearITPendingBit(TIM2, TIM_IT_Update);
}
}
int main(void)
{
GPIO_Configuration();
USART1_Configuration();
TIM2_Configuration();
while (1)
{
if (receive_complete)
{
if (count2 == 0)
{
sscanf(buffer, "%hhu", &count1);
}
else
{
uint8_t new_count = 0;
sscanf(buffer, "%hhu", &new_count);
if (new_count == count2)
{
printf("Quantity is correct.\r\n");
}
else if (new_count > count2)
{
printf("Quantity is less.\r\n");
}
else
{
printf("Quantity is more.\r\n");
}
}
receive_complete = 0;
}
if (count2 == 0)
{
printf("Count: %hhu, Binary: %hhu\r\n", count1, binary);
}
else
{
printf("Count: %hhu, Binary: %hhu\r\n", count2, binary);
}
delay_ms(100);
}
}
void delay_ms(uint16_t ms)
{
volatile uint32_t nCount;
RCC_ClocksTypeDef RCC_Clocks;
RCC_GetClocksFreq(&RCC_Clocks);
nCount = (RCC_Clocks.HCLK_Frequency / 10000) * ms;
for (; nCount != 0; nCount--);
}
相关推荐
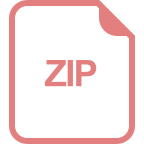
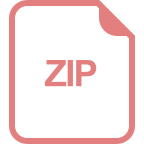
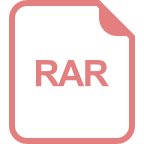








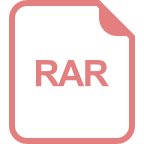
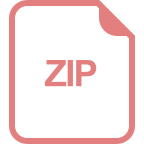
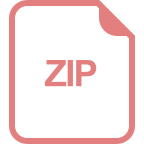
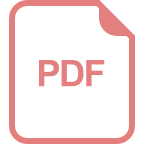
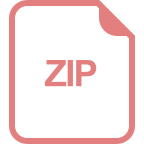
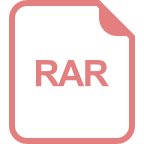
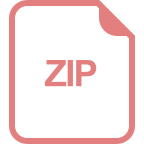
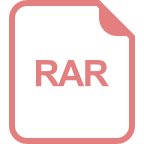