二叉树的创建、遍历、统计叶子结点、求深度等操作(递归算法)部分 编程实现二叉树的二叉链表存储表示的基本操作,这些基本操作至少包括:二叉树的创建、二叉树的先序遍历、中序遍历、后序遍历等的递归算法实现。要求能够进行简单的输入输出验证。
时间: 2024-10-24 13:10:36 浏览: 72
在二叉树的数据结构中,每个节点包含两个指针,分别指向左孩子和右孩子。以下是二叉树的一些基础操作的递归算法实现:
1. **创建二叉树** (递归插入法)
- 定义一个Node类,包含值val、左child和右child属性。
```python
class Node:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
```
- 创建函数`build_tree`接收一个列表,按照元素值构建二叉树。
```python
def build_tree(in_list):
if not in_list: return None
mid = len(in_list) // 2
root = Node(in_list[mid])
root.left = build_tree(in_list[:mid])
root.right = build_tree(in_list[mid+1:])
return root
```
2. **遍历二叉树**
- 先序遍历(根-左-右):
```python
def pre_order(root):
if root is not None:
print(root.val, end=" ")
pre_order(root.left)
pre_order(root.right)
```
-右):
```python
def in_order(root):
if root is not None:
in_order(root.left)
print(root.val, end=" ")
in_order(root.right)
```
- 后序遍历(左-右-根):
```python
def post_order(root):
if root is not None:
post_order(root.left)
post_order(root.right)
print(root.val, end=" ")
```
3. **统计叶子结点** (无子节点)
```python
def count_leaves(root):
if root is None:
return 0
else:
return int(root.left is None and root.right is None)
```
4. **求树的深度** (从根开始递归计算最长路径)
```python
def depth(root):
if root is None:
return 0
else:
left_depth = depth(root.left)
right_depth = depth(root.right)
return max(left_depth, right_depth) + 1
```
为了验证输入输出,你可以构造一个测试用例,例如给定一个已知的二叉树结构的序列,然后创建并遍历树,检查结果是否符合预期。例如:
```python
in_list = [1, 2, 3, 4, 5, 6, 7]
root = build_tree(in_list)
print("Pre-order:", pre_order(root)) # 根据实际树结构打印结果
print("In-order:", in_order(root))
print("Post-order:", post_order(root))
print("Number of leaves:", count_leaves(root))
print("Tree depth:", depth(root))
```
阅读全文
相关推荐
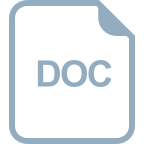
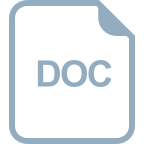
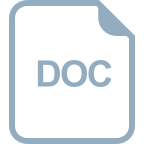















