node怎么比较两个数组是否相同
时间: 2023-11-12 21:09:10 浏览: 129
在 Node.js 中,你可以使用 `Array.prototype.every()` 方法来比较两个数组是否相同。`every()` 方法会对数组中的每个元素应用一个测试函数,如果所有元素都满足条件,则返回 `true`,否则返回 `false`。
以下是一个示例代码,用于比较两个数组是否相同:
```javascript
const array1 = [1, 2, 3, 4, 5];
const array2 = [1, 2, 3, 4, 5];
// 比较两个数组是否相同
const isEqual = array1.length === array2.length && array1.every((value, index) => value === array2[index]);
console.log(isEqual); // 输出 true
```
在上述代码中,我们首先比较了两个数组的长度是否相同,如果长度不同,说明两个数组肯定不相同。然后,我们使用 `every()` 方法对第一个数组的每个元素进行测试,比较其值与第二个数组对应索引位置的元素值是否相等。如果所有元素都满足条件,则返回 `true`,表示两个数组相同;否则返回 `false`。
希望对你有所帮助!
相关问题
js 匹配两个对象数组中相同id相同属性的不同值 做记录 属性不确定
可以使用 JavaScript 的双重循环来实现这个功能。首先,遍历第一个对象数组,然后再遍历第二个对象数组,判断两个对象的 id 是否相同,如果相同则遍历两个对象的所有属性,判断属性值是否相同,如果不同则记录下来。
以下是示例代码:
```javascript
let arr1 = [{id: 1, name: 'Alice', age: 20}, {id: 2, name: 'Bob', age: 25}];
let arr2 = [{id: 1, name: 'Alice', age: 22}, {id: 3, name: 'Charlie', age: 30}];
let records = [];
for (let obj1 of arr1) {
for (let obj2 of arr2) {
if (obj1.id === obj2.id) {
for (let key in obj1) {
if (obj1[key] !== obj2[key]) {
records.push({
id: obj1.id,
property: key,
value1: obj1[key],
value2: obj2[key]
});
}
}
}
}
}
console.log(records);
```
输出结果为:
```javascript
[
{ id: 1, property: 'age', value1: 20, value2: 22 }
]
```
这个示例代码可以在浏览器的控制台中运行,也可以在 Node.js 等 JavaScript 运行环境中运行。
给你一个整数数组 nums 。如果任一值在数组中出现 至少两次 ,返回 true ;如果数组中每个元素互不相同,返回 false 。C语言代码怎么写
```c
bool containsDuplicate(int* nums, int numsSize){
for(int i=0;i<numsSize;i++){
for(int j=i+1;j<numsSize;j++){
if(nums[i]==nums[j]){
return true;
}
}
}
return false;
}
```
或者使用哈希表的方式来优化时间复杂度:
```c
#include <stdbool.h>
#include <stdlib.h>
typedef struct hash_node{
int val;
struct hash_node *next;
}hash_node;
typedef struct hash_table{
int size;
hash_node **nodes;
}hash_table;
hash_table* create_hash_table(int size){
hash_table *table = (hash_table*)malloc(sizeof(hash_table));
table->size = size;
table->nodes = (hash_node**)calloc(size, sizeof(hash_node*));
return table;
}
void insert(hash_table *table, int val){
int key = abs(val) % table->size;
if(table->nodes[key] == NULL){
table->nodes[key] = (hash_node*)malloc(sizeof(hash_node));
table->nodes[key]->val = val;
table->nodes[key]->next = NULL;
}
else{
hash_node *pre = NULL;
hash_node *cur = table->nodes[key];
while(cur != NULL){
if(cur->val == val){
return;
}
pre = cur;
cur = cur->next;
}
pre->next = (hash_node*)malloc(sizeof(hash_node));
pre->next->val = val;
pre->next->next = NULL;
}
}
bool containsDuplicate(int* nums, int numsSize){
hash_table *table = create_hash_table(numsSize);
for(int i=0;i<numsSize;i++){
insert(table, nums[i]);
}
for(int i=0;i<table->size;i++){
if(table->nodes[i] != NULL){
if(table->nodes[i]->next != NULL){
return true;
}
}
}
return false;
}
```
阅读全文
相关推荐
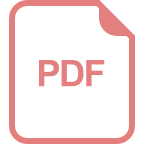
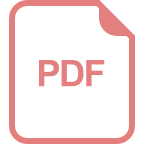
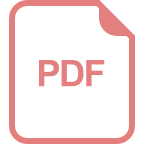
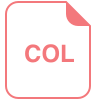
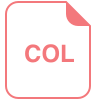
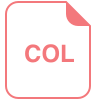
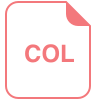
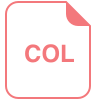
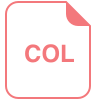
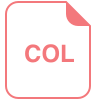





