C# MyCaffe 调用mean.binaryproto train.caffemodel prototxt
时间: 2024-02-24 13:56:15 浏览: 21
在C#中使用MyCaffe调用train.caffemodel和prototxt文件的步骤与使用mean.binaryproto文件类似。您可以使用MyCaffe提供的BlobProto和NetParameter类来加载这些文件,并将它们转换为C#中的对象。
以下是一个示例代码片段,展示了如何在C#中使用MyCaffe加载train.caffemodel和prototxt文件:
```csharp
using MyCaffe;
using MyCaffe.basecode;
using MyCaffe.common;
using MyCaffe.param;
using MyCaffe.proto;
using System.Collections.Generic;
namespace MyCaffeTest
{
class Program
{
static void Main(string[] args)
{
// Load the prototxt file
NetParameter net_param = new NetParameter();
net_param.CopyFrom(LayerParameter.ParseFrom(File.ReadAllBytes("train.prototxt")));
// Load the caffemodel file
BlobProto blob_proto = new BlobProto();
blob_proto.Load("train.caffemodel");
// Convert the BlobProto object to a Blob object
BlobCollection<float> colBlobs = new BlobCollection<float>();
Blob<float> blob = new Blob<float>(new List<int> { 1, 3, 256, 256 });
blob.FromProto(blob_proto);
colBlobs.Add(blob);
// Create the solver
SolverParameter solver_param = new SolverParameter();
solver_param.net_param = net_param;
solver_param.test_iter.Add(0);
solver_param.test_interval = 1;
solver_param.base_lr = 0.01;
solver_param.momentum = 0.9;
solver_param.weight_decay = 5e-4;
solver_param.lr_policy = "inv";
solver_param.gamma = 0.1;
solver_param.power = 0.75;
solver_param.display = 1;
solver_param.max_iter = 1;
solver_param.snapshot = 1;
solver_param.snapshot_prefix = "snapshot";
solver_param.type = SolverParameter.SolverType.SGD;
// Create the solver and solve the net
MyCaffeControl<float> mycaffe = new MyCaffeControl<float>(null, null, null, colBlobs, null, solver_param, null, null, null, null);
mycaffe.Solve();
System.Console.ReadKey();
}
}
}
```
在上面的代码中,我们使用了MyCaffe提供的NetParameter和BlobProto类,分别加载了train.prototxt和train.caffemodel文件。我们还创建了一个SolverParameter对象,并使用NetParameter对象初始化它。最后,我们使用这些对象创建了一个MyCaffeControl对象,并使用Solve函数来训练网络。
希望这可以帮助您解决问题。
相关推荐
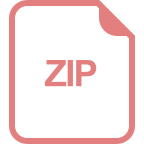














