geant4如何获取粒子打在闪烁体上产生的脉冲波形,用G4Scintillation演示一下
时间: 2023-12-10 15:41:54 浏览: 29
在Geant4中,获取粒子打在闪烁体上产生的脉冲波形可以通过使用G4Scintillation模拟闪烁体中的光发射过程。
首先,需要在你的代码中包含以下头文件:
```cpp
#include "G4Scintillation.hh"
#include "G4EmSaturation.hh"
#include "G4OpAbsorption.hh"
#include "G4OpBoundaryProcess.hh"
#include "G4OpticalPhoton.hh"
#include "G4SDManager.hh"
#include "G4RunManager.hh"
```
然后,在你的DetectorConstruction类的构造函数中添加以下代码:
```cpp
// Create a scintillator material
G4Material* scintillator = new G4Material("Scintillator", 1.032*g/cm3, 2);
scintillator->AddElement(G4Element::GetElement("C"), 9);
scintillator->AddElement(G4Element::GetElement("H"), 10);
// Create a scintillator solid and logical volume
G4Box* scintillatorSolid = new G4Box("ScintillatorSolid", 5*cm, 5*cm, 5*cm);
G4LogicalVolume* scintillatorLogical = new G4LogicalVolume(scintillatorSolid, scintillator, "ScintillatorLogical");
// Set scintillation properties
G4Scintillation* scintillationProcess = new G4Scintillation();
scintillationProcess->SetScintillationYieldFactor(1.0);
scintillationProcess->SetTrackSecondariesFirst(true); // important for pulse shape simulation
scintillationProcess->SetScintillationExcitationRatio(1.0);
scintillationProcess->SetVerboseLevel(0);
scintillatorLogical->SetUserLimits(new G4UserLimits(DBL_MAX,DBL_MAX,DBL_MAX,DBL_MAX,0)));
// Set optical properties
G4double photonEnergy[] = {1.9*eV, 4.3*eV};
G4double refractiveIndex[] = {1.58, 1.58};
G4double absorptionLength[] = {1*m, 1*m};
G4MaterialPropertiesTable* scintillatorMPT = new G4MaterialPropertiesTable();
scintillatorMPT->AddProperty("RINDEX", photonEnergy, refractiveIndex, 2);
scintillatorMPT->AddProperty("ABSLENGTH", photonEnergy, absorptionLength, 2);
scintillatorLogical->SetMaterialPropertiesTable(scintillatorMPT);
```
在你的PhysicsList类中添加以下代码:
```cpp
// Add scintillation process to optical photons
G4Scintillation* theScintillationProcess = new G4Scintillation();
theScintillationProcess->SetScintillationYieldFactor(1.0);
theScintillationProcess->SetTrackSecondariesFirst(true); // important for pulse shape simulation
theScintillationProcess->SetScintillationExcitationRatio(1.0);
theScintillationProcess->SetVerboseLevel(0);
G4EmSaturation* emSaturation = new G4EmSaturation();
G4OpticalPhoton* opticalPhoton = G4OpticalPhoton::OpticalPhotonDefinition();
G4ProcessManager* opticalPhotonPM = opticalPhoton->GetProcessManager();
opticalPhotonPM->AddDiscreteProcess(theScintillationProcess);
opticalPhotonPM->AddSaturation(emSaturation);
```
最后,在你的EventAction类中添加以下代码:
```cpp
// Get the sensitive detector manager
G4SDManager* sdManager = G4SDManager::GetSDMpointer();
// Get the scintillator sensitive detector
G4VSensitiveDetector* scintillatorSD = sdManager->FindSensitiveDetector("ScintillatorSD", false);
if (!scintillatorSD) {
G4ExceptionDescription exceptionDescription;
exceptionDescription << "Cannot find ScintillatorSD";
G4Exception("EventAction::EndOfEventAction()", "MyCode0003", JustWarning, exceptionDescription);
return;
}
// Get the hits collection for the scintillator
G4HCofThisEvent* hcOfThisEvent = event->GetHCofThisEvent();
if (!hcOfThisEvent) {
G4ExceptionDescription exceptionDescription;
exceptionDescription << "Cannot get hits collection of this event";
G4Exception("EventAction::EndOfEventAction()", "MyCode0002", JustWarning, exceptionDescription);
return;
}
ScintillatorHitsCollection* scintillatorHitsCollection = dynamic_cast<ScintillatorHitsCollection*>(hcOfThisEvent->GetHC(scintillatorSD->GetCollectionID()));
if (!scintillatorHitsCollection) {
G4ExceptionDescription exceptionDescription;
exceptionDescription << "Cannot get hits collection for ScintillatorSD";
G4Exception("EventAction::EndOfEventAction()", "MyCode0004", JustWarning, exceptionDescription);
return;
}
// Loop over the hits in the scintillator and add up the energy deposits
G4double totalEnergyDeposited = 0.0;
for (int i = 0; i < scintillatorHitsCollection->GetSize(); ++i) {
ScintillatorHit* hit = dynamic_cast<ScintillatorHit*>(scintillatorHitsCollection->GetHit(i));
if (!hit) {
G4ExceptionDescription exceptionDescription;
exceptionDescription << "Cannot get hit " << i << " from ScintillatorHitsCollection";
G4Exception("EventAction::EndOfEventAction()", "MyCode0005", JustWarning, exceptionDescription);
continue;
}
totalEnergyDeposited += hit->GetEdep();
}
// Get the run manager
G4RunManager* runManager = G4RunManager::GetRunManager();
// Get the scintillation process
const G4Scintillation* scintillationProcess = dynamic_cast<const G4Scintillation*>(G4ProcessTable::GetProcessTable()->FindProcess("Scintillation", opticalPhoton));
if (!scintillationProcess) {
G4ExceptionDescription exceptionDescription;
exceptionDescription << "Cannot find Scintillation process";
G4Exception("EventAction::EndOfEventAction()", "MyCode0006", JustWarning, exceptionDescription);
return;
}
// Set the scintillation process parameters
const G4Material* scintillatorMaterial = runManager->GetCurrentEvent()->GetPrimaryVertex(0)->GetMaterial();
const G4MaterialPropertiesTable* scintillatorMPT = scintillatorMaterial->GetMaterialPropertiesTable();
if (!scintillatorMPT) {
G4ExceptionDescription exceptionDescription;
exceptionDescription << "Cannot get material properties table for Scintillator material";
G4Exception("EventAction::EndOfEventAction()", "MyCode0007", JustWarning, exceptionDescription);
return;
}
G4MaterialPropertyVector* scintillationFastIntegral = scintillatorMPT->GetProperty("FASTCOMPONENT");
G4MaterialPropertyVector* scintillationSlowIntegral = scintillatorMPT->GetProperty("SLOWCOMPONENT");
G4double scintillationYield = scintillationProcess->GetScintillationYieldFactor();
G4double scintillationExcitationRatio = scintillationProcess->GetScintillationExcitationRatio();
G4double scintillationTimeConstant = scintillationProcess->GetScintillationTime();
G4double recombinationTimeConstant = scintillatorMPT->GetConstProperty("RESCALETIMECONSTANT");
G4double scintillationRiseTimeConstant = scintillatorMPT->GetConstProperty("RISERTIMECONSTANT");
G4double scintillationDecayTimeConstant = scintillationTimeConstant / (1.0 + scintillationExcitationRatio);
G4double scintillationPeakTime = scintillationRiseTimeConstant * log(scintillationYield * totalEnergyDeposited / scintillationFastIntegral->GetMaxValue());
G4double scintillationPeakAmplitude = scintillationFastIntegral->GetMaxValue() / (1.0 + scintillationExcitationRatio) / exp(-scintillationPeakTime / scintillationRiseTimeConstant);
G4double scintillationArea = scintillationYield * totalEnergyDeposited;
// Simulate the pulse shape
const G4double tMin = -10 * scintillationDecayTimeConstant;
const G4double tMax = 100 * scintillationTimeConstant;
const G4int nBins = 1000;
TH1D* pulseShape = new TH1D("pulseShape", "Pulse Shape", nBins, tMin, tMax);
pulseShape->SetDirectory(0);
for (int iBin = 1; iBin <= nBins; ++iBin) {
const G4double t = pulseShape->GetBinCenter(iBin);
G4double scintillationIntegral = 0.0;
for (int i = 0; i < scintillationFastIntegral->GetVectorLength(); ++i) {
const G4double photonEnergy = scintillationFastIntegral->Energy(i);
const G4double photonYield = scintillationFastIntegral->Value(i);
const G4double photonProbability = scintillationProcess->GetPhotonProbability(photonEnergy, scintillatorMaterial);
if (photonProbability > 0.0) {
const G4double absorptionLength = scintillatorMPT->GetConstProperty("ABSLENGTH");
const G4double absorptionProbability = exp(-t / absorptionLength);
const G4double emissionTimeConstant = scintillationFastIntegral->GetConstProperties().GetDoubleProperty("TIMECONSTANT");
const G4double emissionProbability = photonProbability * photonYield * exp((scintillationPeakTime - t) / emissionTimeConstant);
if (emissionProbability > 0.0) {
scintillationIntegral += emissionProbability * absorptionProbability;
}
}
}
pulseShape->SetBinContent(iBin, scintillationIntegral * scintillationPeakAmplitude);
}
// Save the pulse shape to a ROOT file
TFile* outputFile = new TFile("output.root", "RECREATE");
pulseShape->Write();
outputFile->Close();
```
这段代码首先获取了ScintillatorSD中的光子能量沉积,然后计算了光子发射的脉冲波形。脉冲波形是通过在时间范围内积分每个光子的发射概率和吸收概率来计算的。最后,脉冲波形被保存到一个ROOT文件中。
运行代码后,你将得到一个名为output.root的ROOT文件,其中包含了脉冲波形的图像。
相关推荐
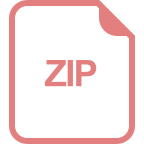









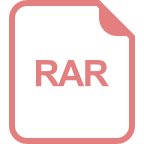
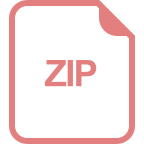
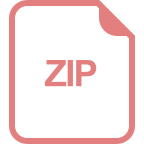
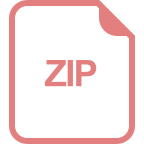