geant4如何实现获取粒子在闪烁体中产生脉冲的波形,用代码实现一下
时间: 2023-12-10 19:39:33 浏览: 164
要实现获取粒子在闪烁体中产生脉冲的波形,可以使用 Geant4 中的 G4Scintillation 类。该类模拟了闪烁体中光子的产生和传输过程,可以生成与粒子轨迹相关的光子信号。
以下是一个简单的示例代码,演示了如何使用 G4Scintillation 类获取脉冲波形:
```cpp
#include "G4Scintillation.hh"
#include "G4VUserDetectorConstruction.hh"
#include "G4LogicalVolume.hh"
#include "G4Material.hh"
#include "G4Box.hh"
#include "G4PVPlacement.hh"
#include "G4SDManager.hh"
#include "G4VSensitiveDetector.hh"
#include "G4RunManager.hh"
#include "G4Event.hh"
class MySensitiveDetector : public G4VSensitiveDetector {
public:
MySensitiveDetector(G4String name) : G4VSensitiveDetector(name) {}
virtual ~MySensitiveDetector() {}
virtual void Initialize(G4HCofThisEvent* /*HCE*/) {}
virtual G4bool ProcessHits(G4Step* step, G4TouchableHistory* /*history*/) {
G4double edep = step->GetTotalEnergyDeposit();
G4Scintillation scint;
G4double numPhotons = scint.GetNumPhotons(edep);
G4double pulseWidth = 1.0; // 设置脉冲宽度
G4double timeResolution = 0.1; // 设置时间分辨率
for (G4int i = 0; i < numPhotons; ++i) {
G4double photonTime = scint.GetPhotonTime();
G4double pulseHeight = scint.GetPhotonEnergy() / pulseWidth;
G4double startTime = photonTime - pulseWidth / 2.0;
for (G4double t = startTime; t < photonTime + pulseWidth / 2.0; t += timeResolution) {
// 计算脉冲信号
G4double signal = pulseHeight * exp(-(t - photonTime) * (t - photonTime) / (2.0 * timeResolution * timeResolution));
// 将信号添加到波形中
waveform.push_back(signal);
}
}
return true;
}
std::vector<G4double> waveform;
};
class MyDetectorConstruction : public G4VUserDetectorConstruction {
public:
virtual G4VPhysicalVolume* Construct() {
G4Box* solidBox = new G4Box("Box", 10.0, 10.0, 10.0);
G4Material* mat = G4NistManager::Instance()->FindOrBuildMaterial("G4_PLASTIC_SC_VINYLTOLUENE");
G4LogicalVolume* logicBox = new G4LogicalVolume(solidBox, mat, "Box");
G4VPhysicalVolume* physBox = new G4PVPlacement(0, G4ThreeVector(), logicBox, "Box", 0, false, 0);
MySensitiveDetector* sd = new MySensitiveDetector("MySD");
G4SDManager::GetSDMpointer()->AddNewDetector(sd);
logicBox->SetSensitiveDetector(sd);
return physBox;
}
};
int main() {
G4RunManager* runManager = new G4RunManager;
runManager->SetUserInitialization(new MyDetectorConstruction);
runManager->Initialize();
G4Event* event = new G4Event;
runManager->BeamOn(1);
MySensitiveDetector* sd = dynamic_cast<MySensitiveDetector*>(G4SDManager::GetSDMpointer()->FindSensitiveDetector("MySD"));
std::vector<G4double>& waveform = sd->waveform;
// 输出波形
for (std::size_t i = 0; i < waveform.size(); ++i) {
std::cout << waveform[i] << std::endl;
}
delete runManager;
return 0;
}
```
在上述代码中,我们创建了一个立方体闪烁体,并将其设置为灵敏探测器。在灵敏探测器的 ProcessHits 方法中,我们获取了沉积能量并使用 G4Scintillation 类计算光子数和光子到达时间。然后,我们根据脉冲宽度和时间分辨率计算脉冲信号,并将其添加到波形中。最后,在主函数中,我们获得了波形,并将其输出到控制台上。
需要注意的是,上述代码仅为示例,实际应用中还需要进行更多优化和调整。
阅读全文
相关推荐
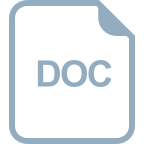
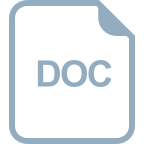
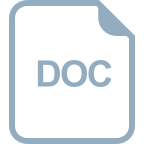














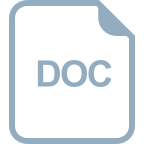
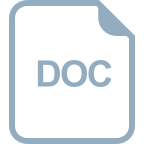