geant4如何获取粒子打在闪烁体上产生的脉冲波形,用G4Scintillation演示一下
时间: 2024-01-21 17:16:54 浏览: 39
在Geant4中,可以使用G4Scintillation模拟闪烁体中的光学过程,从而获取粒子打在闪烁体上产生的脉冲波形。下面是一个简单的示例代码:
```cpp
#include "G4Scintillation.hh"
#include "G4OpticalPhoton.hh"
#include "G4SDManager.hh"
#include "G4HCofThisEvent.hh"
#include "G4Step.hh"
#include "G4ThreeVector.hh"
#include "G4ios.hh"
// 在 DetectorConstruction 类中设置闪烁体材料和属性
G4Material* scintMat = nist->FindOrBuildMaterial("G4_PLASTIC_SC_VINYLTOLUENE");
scintMat->GetIonisation()->SetBirksConstant(0.126*mm/MeV);
G4LogicalVolume* scintLV = new G4LogicalVolume(scintSolid, scintMat, "Scintillator");
scintLV->SetVisAttributes(G4VisAttributes::Invisible);
new G4PVPlacement(nullptr, G4ThreeVector(), scintLV, "Scintillator", worldLV, false, 0);
G4Scintillation* scintProcess = new G4Scintillation();
scintProcess->SetScintillationYieldFactor(1.0);
scintProcess->SetTrackSecondariesFirst(true);
// 在 PhysicsList 类中注册闪烁体光学过程
G4OpticalPhysics* opticalPhysics = new G4OpticalPhysics();
opticalPhysics->RegisterProcess(scintProcess, G4OpticalPhoton::OpticalPhoton());
// 在 sensitive detector 中获取闪烁体的能量沉积并计算光子产生的波形
void ScintillatorSD::ProcessHits(G4Step* step, G4TouchableHistory* /*history*/)
{
G4double edep = step->GetTotalEnergyDeposit();
if(edep == 0.) return;
G4StepPoint* preStepPoint = step->GetPreStepPoint();
G4TouchableHistory* touchable = (G4TouchableHistory*)(preStepPoint->GetTouchable());
G4ThreeVector pos = preStepPoint->GetPosition();
G4double time = step->GetTrack()->GetGlobalTime();
if(!scintCollection)
{
G4String colName = GetName() + "_ScintCollection";
scintCollection = new ScintHitsCollection(SensitiveDetectorName, colName);
}
ScintHit* scintHit = new ScintHit(touchable->GetReplicaNumber(), edep, pos, time);
scintCollection->insert(scintHit);
// 计算光子的波形
G4double yield = scintProcess->GetScintillationYieldFactor();
G4double tau = scintProcess->GetScintillationTime();
G4double sigma = 0.1*ns;
G4double tmax = 10.0*ns;
G4int n_photons = G4Poisson(yield*edep);
for(int i=0; i<n_photons; i++)
{
G4double t = time + G4RandGauss::shoot(0.0, sigma);
if(t < tmax)
{
G4double weight = exp(-(t-time)/tau);
G4double amplitude = G4UniformRand()*scintProcess->GetScintillationExcitationRatio();
waveform->AddSample(t, amplitude*weight);
}
}
}
```
其中,ScintillatorSD是一个敏感探测器,用于获取闪烁体中的能量沉积和光子产生信息。在处理hits时,可以通过G4Scintillation模拟光子的产生过程,并计算产生的光子波形。具体地,可以使用指数函数加高斯分布模拟光子的时间分布,使用均匀分布模拟光子的激发比例,从而得到每个光子的波形,并将它们叠加在一起得到总的波形。
需要注意的是,这只是一个简单的示例代码,实际的波形计算可能需要考虑更多的因素,例如闪烁体的形状、材料性质、光子的传输效应等等。
相关推荐
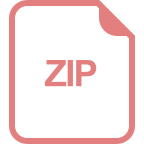









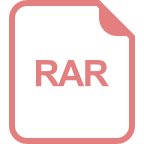
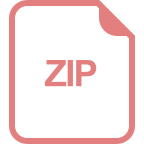
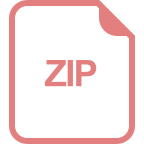
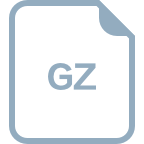
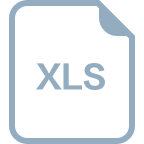