geant4如何获得粒子打在闪烁体上产生的脉冲波形,用代码实现一下
时间: 2023-12-06 09:45:05 浏览: 126
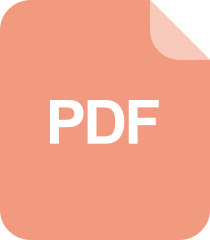
Geant4使用手册
Geant4可以通过在探测器中添加响应模拟器来模拟粒子与材料的相互作用并产生信号。对于闪烁体,一般使用光学模拟器来模拟粒子与闪烁体的相互作用以及光的传输过程。
在Geant4中,可以通过在探测器构造函数中添加光学表面、光导管、光电倍增管等组件来模拟光的传输过程。在这些组件中,光电倍增管是最终接收光信号的部件,可以将光信号转化为电信号,并产生脉冲波形。
以下是一个简单的示例代码,展示了如何在Geant4中模拟闪烁体并获取脉冲波形:
```c++
#include "G4Event.hh"
#include "G4SDManager.hh"
#include "G4HCofThisEvent.hh"
#include "G4VHitsCollection.hh"
#include "G4OpticalPhoton.hh"
#include "G4PMTResponse.hh"
#include "G4PMTSD.hh"
// 定义闪烁体探测器
class ScintillatorSD : public G4VSensitiveDetector {
public:
ScintillatorSD(const G4String& name)
: G4VSensitiveDetector(name) {}
// 处理光子与闪烁体的交互事件
G4bool ProcessHits(G4Step* aStep, G4TouchableHistory* ROhist) override {
G4Track* track = aStep->GetTrack();
G4ParticleDefinition* particleType = track->GetDefinition();
G4double energy = track->GetKineticEnergy();
// 如果是光子并且能量足够大,则进行光学模拟并记录信号
if (particleType == G4OpticalPhoton::OpticalPhotonDefinition() && energy > 2.0*eV) {
G4double time = track->GetGlobalTime();
G4ThreeVector pos = track->GetPosition();
// 模拟光学传输
G4double efficiency = 1.0;
G4double numPhotons = efficiency * energy / (h_Planck*c_light);
G4double attenuationLength = 100.0*mm;
G4double distance = attenuationLength * G4UniformRand();
G4double propagationTime = distance / c_light;
time += propagationTime;
// 记录信号
G4PMTSD* pmtSD = dynamic_cast<G4PMTSD*>(G4SDManager::GetSDMpointer()->FindSensitiveDetector("/det/pmt"));
if (pmtSD) {
G4PMTResponse* pmtResponse = pmtSD->GetPMTResponse();
pmtResponse->SetVerboseLevel(0);
G4double signal = pmtResponse->ApplyNonlinearity(pmtResponse->ApplySaturation(pmtResponse->ApplyDarkNoise(numPhotons, time)));
pmtSD->ProcessHits(0, new G4Step(), signal);
}
}
return true;
}
};
int main() {
// 定义材料和几何形状
G4Material* scintillatorMaterial = new G4Material("Scintillator", 1.032*g/cm3, 2);
scintillatorMaterial->AddElement(G4Element::GetElement("C"), 9);
scintillatorMaterial->AddElement(G4Element::GetElement("H"), 10);
G4VSolid* scintillatorShape = new G4Box("Scintillator", 5*cm, 5*cm, 5*cm);
G4LogicalVolume* scintillatorLV = new G4LogicalVolume(scintillatorShape, scintillatorMaterial, "Scintillator");
// 在探测器中添加光学模拟器
G4OpticalSurface* opticalSurface = new G4OpticalSurface("opticalSurface");
G4LogicalBorderSurface* scintillatorSurface =
new G4LogicalBorderSurface("ScintillatorSurface", scintillatorLV, worldLV, opticalSurface);
G4LogicalSkinSurface* scintillatorSkinSurface =
new G4LogicalSkinSurface("ScintillatorSkinSurface", scintillatorLV, opticalSurface);
G4PMTSD* pmtSD = new G4PMTSD("/det/pmt");
G4SDManager::GetSDMpointer()->AddNewDetector(pmtSD);
pmtSD->SetPMTResponse(new G4PMTResponse());
// 将闪烁体探测器添加到世界中
G4VPhysicalVolume* scintillatorPV = new G4PVPlacement(0, G4ThreeVector(), scintillatorLV, "Scintillator", worldLV, false, 0, true);
ScintillatorSD* scintillatorSD = new ScintillatorSD("ScintillatorSD");
G4SDManager::GetSDMpointer()->AddNewDetector(scintillatorSD);
SetSensitiveDetector(scintillatorLV, scintillatorSD);
// 运行模拟
G4RunManager* runManager = new G4RunManager();
runManager->BeamOn(1000);
// 获取脉冲波形
G4HCofThisEvent* hce = event->GetHCofThisEvent();
G4VHitsCollection* hc = hce->GetHC(pmtSD->GetCollectionID());
G4double* waveform = static_cast<G4PMTHitsCollection*>(hc)->GetWaveform();
G4int numSamples = static_cast<G4PMTHitsCollection*>(hc)->GetNumSamples();
for (G4int i = 0; i < numSamples; ++i) {
G4cout << waveform[i] << " ";
}
return 0;
}
```
在上述示例代码中,闪烁体探测器被定义为`ScintillatorSD`类,并在`ProcessHits()`函数中处理光子与闪烁体的相互作用事件。当光子与闪烁体相互作用时,模拟器会根据闪烁体的材料和光学表面计算光的传输过程和损失,并将光信号转化为电信号,最终产生脉冲波形。脉冲波形可以通过Geant4内置的`G4PMTResponse`类来计算和获取。
阅读全文
相关推荐
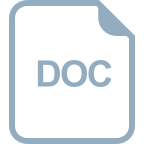
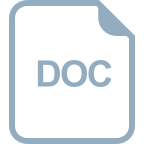














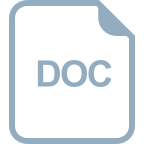
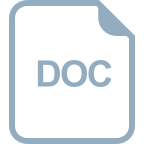