stm32怎么用一段c代码简单实现按键一控制正向流水灯(按一下动一下) 按键二控制反向流水灯(按一下动一下) 按键三控制流水灯的数量(1-3个)
时间: 2023-05-28 22:07:11 浏览: 386
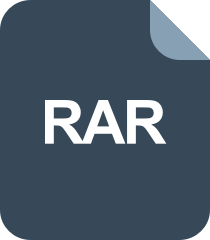
HAL库编写 STM32F103C8 带按键控制流水灯

以下是一个简单的实现,使用了STM32的HAL库:
```c
#include "stm32f4xx_hal.h"
// 定义LED灯的GPIO端口和引脚
#define LED_GPIO_PORT GPIOD
#define LED_PIN_1 GPIO_PIN_12
#define LED_PIN_2 GPIO_PIN_13
#define LED_PIN_3 GPIO_PIN_14
// 定义按键的GPIO端口和引脚
#define BUTTON_GPIO_PORT GPIOA
#define BUTTON_PIN_1 GPIO_PIN_0
#define BUTTON_PIN_2 GPIO_PIN_1
#define BUTTON_PIN_3 GPIO_PIN_2
// 定义流水灯的数量
int num_lights = 1;
// 定义流水灯的方向
int direction = 1; // 1为正向,-1为反向
// 定义按键状态
int button_1_pressed = 0;
int button_2_pressed = 0;
int button_3_pressed = 0;
// 初始化GPIO
void init_gpio()
{
// 使能GPIO时钟
__HAL_RCC_GPIOA_CLK_ENABLE();
__HAL_RCC_GPIOD_CLK_ENABLE();
// 初始化LED灯的GPIO
GPIO_InitTypeDef GPIO_InitStruct;
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP;
GPIO_InitStruct.Pull = GPIO_NOPULL;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH;
GPIO_InitStruct.Pin = LED_PIN_1 | LED_PIN_2 | LED_PIN_3;
HAL_GPIO_Init(LED_GPIO_PORT, &GPIO_InitStruct);
// 初始化按键的GPIO
GPIO_InitStruct.Mode = GPIO_MODE_INPUT;
GPIO_InitStruct.Pull = GPIO_PULLUP;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH;
GPIO_InitStruct.Pin = BUTTON_PIN_1 | BUTTON_PIN_2 | BUTTON_PIN_3;
HAL_GPIO_Init(BUTTON_GPIO_PORT, &GPIO_InitStruct);
}
int main(void)
{
// 初始化HAL库
HAL_Init();
// 初始化GPIO
init_gpio();
while (1)
{
// 检测按键1是否按下
if (HAL_GPIO_ReadPin(BUTTON_GPIO_PORT, BUTTON_PIN_1) == GPIO_PIN_SET && !button_1_pressed)
{
button_1_pressed = 1;
// 切换流水灯方向
direction = -direction;
}
else if (HAL_GPIO_ReadPin(BUTTON_GPIO_PORT, BUTTON_PIN_1) == GPIO_PIN_RESET)
{
button_1_pressed = 0;
}
// 检测按键2是否按下
if (HAL_GPIO_ReadPin(BUTTON_GPIO_PORT, BUTTON_PIN_2) == GPIO_PIN_SET && !button_2_pressed)
{
button_2_pressed = 1;
// 切换流水灯数量
num_lights++;
if (num_lights > 3)
{
num_lights = 1;
}
}
else if (HAL_GPIO_ReadPin(BUTTON_GPIO_PORT, BUTTON_PIN_2) == GPIO_PIN_RESET)
{
button_2_pressed = 0;
}
// 检测按键3是否按下
if (HAL_GPIO_ReadPin(BUTTON_GPIO_PORT, BUTTON_PIN_3) == GPIO_PIN_SET && !button_3_pressed)
{
button_3_pressed = 1;
}
else if (HAL_GPIO_ReadPin(BUTTON_GPIO_PORT, BUTTON_PIN_3) == GPIO_PIN_RESET)
{
button_3_pressed = 0;
}
// 控制LED灯的流水效果
if (direction == 1)
{
for (int i = 0; i < num_lights; i++)
{
HAL_GPIO_WritePin(LED_GPIO_PORT, LED_PIN_1 << i, GPIO_PIN_SET);
HAL_Delay(100);
HAL_GPIO_WritePin(LED_GPIO_PORT, LED_PIN_1 << i, GPIO_PIN_RESET);
}
}
else if (direction == -1)
{
for (int i = num_lights - 1; i >= 0; i--)
{
HAL_GPIO_WritePin(LED_GPIO_PORT, LED_PIN_1 << i, GPIO_PIN_SET);
HAL_Delay(100);
HAL_GPIO_WritePin(LED_GPIO_PORT, LED_PIN_1 << i, GPIO_PIN_RESET);
}
}
}
}
```
在这个代码中,我们使用了一个`init_gpio()`函数来初始化GPIO端口和引脚。在`main()`函数中,我们使用了一个无限循环来检测按键状态和控制LED灯的流水效果。
在每个按键被按下的时候,我们会更新相应的状态变量,然后根据状态变量来改变流水灯的方向和数量。在控制LED灯的流水效果时,我们根据流水灯的方向和数量来控制LED灯的亮灭。
阅读全文
相关推荐
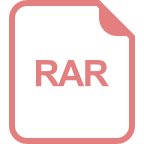





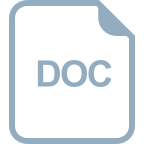





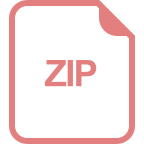
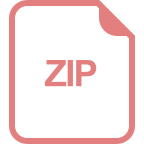
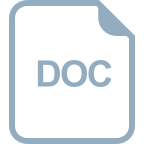
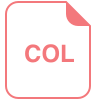
