计算平均绩点c语言第一行一个整数 t(1 <= t <= 100) 代表测试用例组数。每组测试用例包含三行: 每组测试样例第一行一个整数 n (1 <= n <= 30)表示该学年课程总数, 第二行有 n 个小数 a1, a2, ... , an (0.1 <= ai <= 10.0)表示第 i 门课程的学分数。 第三行有 n 个整数 b1, b2, ... , bn (0 <= bi <= 100)表示第 i 门课程的成绩。
时间: 2023-12-25 07:04:43 浏览: 27
好的,你的问题是如何计算平均绩点,对吗?
平均绩点可以按照以下公式计算:
GPA = Σ(学分×绩点) / Σ学分
其中,Σ表示求和,学分是指每门课程的学分数,绩点是指每门课程的绩点,可以按照以下表格进行对应:
| 分数 | 绩点 |
| ---- | ---- |
| 90~100 | 4.0 |
| 85~89 | 3.7 |
| 82~84 | 3.3 |
| 78~81 | 3.0 |
| 75~77 | 2.7 |
| 72~74 | 2.3 |
| 68~71 | 2.0 |
| 64~67 | 1.5 |
| 60~63 | 1.0 |
| 0~59 | 0.0 |
根据上述公式和表格,我们可以编写如下的 C 语言代码实现平均绩点的计算:
```c
#include <stdio.h>
int main() {
int t;
scanf("%d", &t);
while (t--) {
int n, credit[30], score[30];
double gpa = 0, total_credit = 0;
scanf("%d", &n);
for (int i = 0; i < n; i++) {
scanf("%d", &credit[i]);
total_credit += credit[i];
}
for (int i = 0; i < n; i++) {
scanf("%d", &score[i]);
if (score[i] >= 90) {
gpa += 4.0 * credit[i];
} else if (score[i] >= 85) {
gpa += 3.7 * credit[i];
} else if (score[i] >= 82) {
gpa += 3.3 * credit[i];
} else if (score[i] >= 78) {
gpa += 3.0 * credit[i];
} else if (score[i] >= 75) {
gpa += 2.7 * credit[i];
} else if (score[i] >= 72) {
gpa += 2.3 * credit[i];
} else if (score[i] >= 68) {
gpa += 2.0 * credit[i];
} else if (score[i] >= 64) {
gpa += 1.5 * credit[i];
} else if (score[i] >= 60) {
gpa += 1.0 * credit[i];
}
}
printf("%.2lf\n", gpa / total_credit);
}
return 0;
}
```
其中,t 表示测试用例组数,n 表示每组测试用例中课程的总数,credit 数组用于存储每门课程的学分数,score 数组用于存储每门课程的成绩,gpa 表示总绩点,total_credit 表示总学分。程序通过循环读入每组测试用例的数据,并按照上述公式和表格进行计算,最终输出平均绩点。
相关推荐
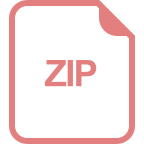
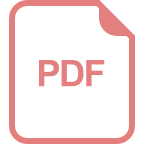
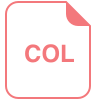
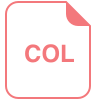
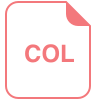
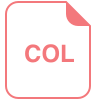










