2.实现睡觉的理发师问题(同步互斥方式采用信号量或mutex方式均可) 理发师问题的描述:一个理发店接待室有n张椅子,工作室有1张椅子;没有顾客时,理发师睡觉;第一个顾客来到时,必须将理发师唤醒;顾客来时如果还有空座的话,他就坐在一个座位上等待;如果顾客来时没有空座位了,他就离开,不理发了;当理发师处理完所有顾客,而又没有新顾客来时,他又开始睡觉。这题使用Java完成代码
时间: 2024-03-14 07:46:45 浏览: 60
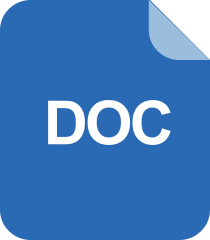
理发师问题的实现 课程设计
以下是使用Java实现的睡觉的理发师问题:
```java
import java.util.concurrent.Semaphore;
public class BarberShop {
private int numWaitingSeats;
private Semaphore barberSleeping;
private Semaphore barberReady;
private Semaphore customerReady;
private Semaphore accessSeats;
private Semaphore leaveBarberChair;
public BarberShop(int numWaitingSeats) {
this.numWaitingSeats = numWaitingSeats;
barberSleeping = new Semaphore(0);
barberReady = new Semaphore(0);
customerReady = new Semaphore(0);
accessSeats = new Semaphore(1);
leaveBarberChair = new Semaphore(0);
}
public void start() {
Thread barberThread = new Thread(new Barber());
barberThread.start();
for (int i = 1; i <= 10; i++) {
Thread customerThread = new Thread(new Customer(i));
customerThread.start();
}
}
class Barber implements Runnable {
public void run() {
while (true) {
try {
System.out.println("Barber is sleeping...");
barberSleeping.acquire();
System.out.println("Barber is ready to cut hair.");
barberReady.release();
Thread.sleep(500);
System.out.println("Barber finished cutting hair.");
leaveBarberChair.release();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
class Customer implements Runnable {
private int id;
public Customer(int id) {
this.id = id;
}
public void run() {
try {
accessSeats.acquire();
if (numWaitingSeats > 0) {
numWaitingSeats--;
System.out.println("Customer " + id + " is waiting.");
accessSeats.release();
customerReady.release();
barberSleeping.release();
barberReady.acquire();
System.out.println("Customer " + id + " is getting a haircut.");
leaveBarberChair.acquire();
System.out.println("Customer " + id + " left the barbershop.");
accessSeats.acquire();
numWaitingSeats++;
} else {
accessSeats.release();
System.out.println("Customer " + id + " left the barbershop.");
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public static void main(String[] args) {
BarberShop barberShop = new BarberShop(5);
barberShop.start();
}
}
```
这个程序使用了五个信号量来实现同步和互斥:`barberSleeping`用于唤醒理发师,`barberReady`用于指示理发师已准备好理发,`customerReady`用于通知理发师有顾客等待,`accessSeats`用于控制对等待座位的访问,`leaveBarberChair`用于通知客户理发已完成。
在`start()`方法中,我们启动一个理发师线程和十个顾客线程。
在`Barber`类中,理发师线程将一直循环执行,如果没有顾客,它将等待。如果有顾客,他将准备好理发并开始工作。工作时间为500ms。最后,他会释放`leaveBarberChair`信号量,通知顾客理发已完成。
在`Customer`类中,顾客线程将首先等待访问`accessSeats`信号量,以便访问等待座位。如果有空座,他会占用一个座位并释放`accessSeats`信号量,然后通知理发师有人等待。然后他会等待理发师准备好,并接受理发。最后,他会释放`leaveBarberChair`信号量,以通知理发师理发完成。如果没有空座位,他将释放`accessSeats`信号量并离开理发店。
在`main`方法中,我们创建了一个`BarberShop`对象,并传递了等待座位的数量。然后我们启动程序。
阅读全文
相关推荐
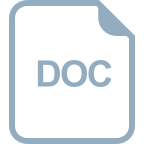
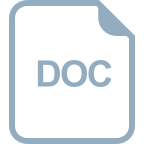




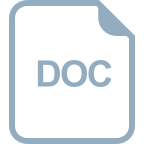
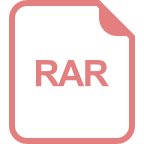
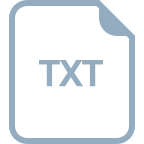
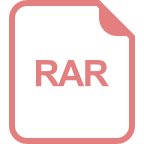
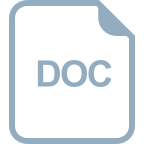
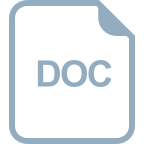
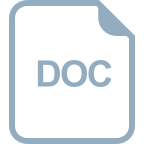
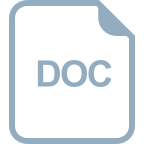
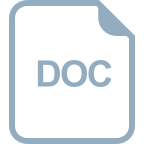


