用c编写2.实现睡觉的理发师问题(同步互斥方式采用信号量或mutex方式均可)理发师问题的描述:一个理发店接待室有n张椅子,工作室有1张椅子;没有顾客时,理发师睡觉;第一个顾客来到时,必须将理发师唤醒;顾客来时如果还有空座的话,他就坐在一个座位上等待;如果顾客来时没有空座位了,他就离开,不理发了;当理发师处理完所有顾客,而又没有新顾客来时,他又开始睡觉
时间: 2024-03-01 11:50:35 浏览: 179
好的,这是一个经典的同步互斥问题,可以使用信号量或者互斥锁来解决。以下是一个使用信号量解决的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <semaphore.h>
#define N 5 // 等待室椅子数量
sem_t customers; // 顾客信号量
sem_t barber; // 理发师信号量
sem_t mutex; // 互斥锁
int waiting = 0; // 等待的顾客数目
void *barber_func(void *arg)
{
while (1) {
sem_wait(&customers); // 等待顾客
sem_wait(&mutex);
waiting--;
sem_post(&mutex);
sem_post(&barber); // 叫理发师理发
printf("Barber is cutting hair\n");
sleep(1);
sem_wait(&mutex);
if (waiting == 0) { // 没有顾客了
sem_post(&mutex);
printf("Barber is sleeping\n");
sleep(1);
} else {
sem_post(&mutex);
}
}
}
void *customer_func(void *arg)
{
while (1) {
sem_wait(&mutex);
if (waiting < N) { // 还有座位,进入等待室等待
waiting++;
sem_post(&customers);
sem_post(&mutex);
sem_wait(&barber);
printf("Customer is getting a haircut\n");
sleep(1);
} else { // 没有座位,离开
sem_post(&mutex);
printf("Customer left\n");
sleep(1);
}
}
}
int main()
{
pthread_t barber_thread, customer_thread[N];
sem_init(&customers, 0, 0);
sem_init(&barber, 0, 0);
sem_init(&mutex, 0, 1);
pthread_create(&barber_thread, NULL, barber_func, NULL);
for (int i = 0; i < N; i++) {
pthread_create(&customer_thread[i], NULL, customer_func, NULL);
}
pthread_join(barber_thread, NULL);
for (int i = 0; i < N; i++) {
pthread_join(customer_thread[i], NULL);
}
sem_destroy(&customers);
sem_destroy(&barber);
sem_destroy(&mutex);
return 0;
}
```
在这个示例代码中,我们使用了三个信号量 `customers`, `barber` 和 `mutex`,分别用来表示等待室中的顾客数目、理发师的状态和互斥锁。在主函数中,我们创建了一个理发师线程和 `N` 个顾客线程,并且对信号量进行了初始化。在理发师线程中,理发师会不断地等待顾客来到,如果有顾客来了,他会先将等待室中的顾客数目减一,然后对 `barber` 信号量进行操作,叫醒理发师理发。理发师理发的时间是 1 秒钟,然后他会检查等待室中是否还有顾客,如果没有则继续睡觉,否则继续等待。在顾客线程中,顾客会不断地尝试进入等待室,如果等待室还有座位,则等待室中的顾客数目加一,然后对 `customers` 信号量进行操作,叫醒理发师理发。理发师理发的时间是 1 秒钟,然后顾客离开等待室。如果等待室没有座位,则直接离开。
阅读全文
相关推荐






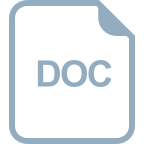

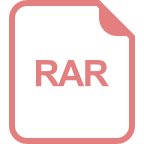







