python小游戏五子棋
时间: 2023-06-23 19:59:05 浏览: 52
以下是一个简单的 Python 五子棋游戏的代码:
```python
import numpy as np
# 定义棋盘大小
BOARD_SIZE = 15
# 定义棋子类型
EMPTY = 0
BLACK = 1
WHITE = 2
# 定义棋盘
board = np.zeros((BOARD_SIZE, BOARD_SIZE))
# 判断是否胜利
def check_win(player):
# 判断横向是否有五子连珠
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE - 4):
if board[i][j] == player and board[i][j + 1] == player and board[i][j + 2] == player and board[i][j + 3] == player and board[i][j + 4] == player:
return True
# 判断纵向是否有五子连珠
for i in range(BOARD_SIZE - 4):
for j in range(BOARD_SIZE):
if board[i][j] == player and board[i + 1][j] == player and board[i + 2][j] == player and board[i + 3][j] == player and board[i + 4][j] == player:
return True
# 判断左上到右下是否有五子连珠
for i in range(BOARD_SIZE - 4):
for j in range(BOARD_SIZE - 4):
if board[i][j] == player and board[i + 1][j + 1] == player and board[i + 2][j + 2] == player and board[i + 3][j + 3] == player and board[i + 4][j + 4] == player:
return True
# 判断右上到左下是否有五子连珠
for i in range(BOARD_SIZE - 4):
for j in range(4, BOARD_SIZE):
if board[i][j] == player and board[i + 1][j - 1] == player and board[i + 2][j - 2] == player and board[i + 3][j - 3] == player and board[i + 4][j - 4] == player:
return True
return False
# 打印棋盘
def print_board():
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if board[i][j] == EMPTY:
print(".", end=" ")
elif board[i][j] == BLACK:
print("X", end=" ")
elif board[i][j] == WHITE:
print("O", end=" ")
print()
print()
# 下棋
def play(player):
print("Player", player, "turn")
x = int(input("Enter x coordinate:"))
y = int(input("Enter y coordinate:"))
if board[x][y] != EMPTY:
print("Invalid move, try again")
play(player)
else:
board[x][y] = player
print_board()
if check_win(player):
print("Player", player, "wins!")
elif np.count_nonzero(board == EMPTY) == 0:
print("Game over, it's a tie!")
else:
play(BLACK if player == WHITE else WHITE)
# 开始游戏
print_board()
play(BLACK)
```
在这个游戏中,黑色棋子为 X,白色棋子为 O。玩家每次输入棋子的坐标,然后程序判断该位置是否可用。如果可用,程序就在该位置放置棋子,并检查是否有五子连珠。如果有五子连珠,程序就宣布该玩家胜利。如果棋盘已满,程序就宣布平局。否则,程序就切换到下一个玩家的回合。
相关推荐
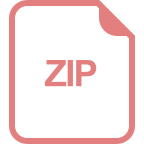
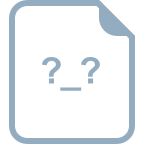













