python五子棋游戏代码
时间: 2023-04-06 07:00:29 浏览: 199
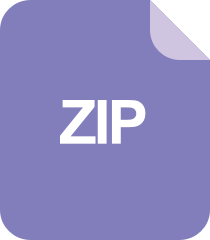
python五子棋代码
你好!以下是 Python 五子棋游戏的代码:
```python
import numpy as np
# 定义棋盘大小
BOARD_SIZE = 15
# 定义棋子类型
EMPTY = 0
BLACK = 1
WHITE = 2
# 定义胜利条件
WIN_COUNT = 5
# 初始化棋盘
board = np.zeros((BOARD_SIZE, BOARD_SIZE), dtype=int)
# 定义落子函数
def move(color, x, y):
if board[x][y] == EMPTY:
board[x][y] = color
return True
else:
return False
# 定义判断胜利函数
def is_win(color, x, y):
count = 1
# 横向判断
for i in range(1, WIN_COUNT):
if y-i >= 0 and board[x][y-i] == color:
count += 1
else:
break
for i in range(1, WIN_COUNT):
if y+i < BOARD_SIZE and board[x][y+i] == color:
count += 1
else:
break
if count >= WIN_COUNT:
return True
# 纵向判断
count = 1
for i in range(1, WIN_COUNT):
if x-i >= 0 and board[x-i][y] == color:
count += 1
else:
break
for i in range(1, WIN_COUNT):
if x+i < BOARD_SIZE and board[x+i][y] == color:
count += 1
else:
break
if count >= WIN_COUNT:
return True
# 左上到右下判断
count = 1
for i in range(1, WIN_COUNT):
if x-i >= 0 and y-i >= 0 and board[x-i][y-i] == color:
count += 1
else:
break
for i in range(1, WIN_COUNT):
if x+i < BOARD_SIZE and y+i < BOARD_SIZE and board[x+i][y+i] == color:
count += 1
else:
break
if count >= WIN_COUNT:
return True
# 右上到左下判断
count = 1
for i in range(1, WIN_COUNT):
if x-i >= 0 and y+i < BOARD_SIZE and board[x-i][y+i] == color:
count += 1
else:
break
for i in range(1, WIN_COUNT):
if x+i < BOARD_SIZE and y-i >= 0 and board[x+i][y-i] == color:
count += 1
else:
break
if count >= WIN_COUNT:
return True
return False
# 定义主函数
def main():
# 初始化游戏
color = BLACK
while True:
print(board)
x = int(input("请输入落子的横坐标:"))
y = int(input("请输入落子的纵坐标:"))
if move(color, x, y):
if is_win(color, x, y):
print("恭喜,{}方获胜!".format("黑" if color == BLACK else "白"))
break
color = WHITE if color == BLACK else BLACK
else:
print("该位置已有棋子,请重新输入!")
if __name__ == '__main__':
main()
```
希望对你有帮助!
阅读全文
相关推荐




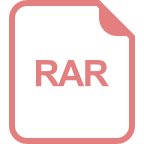








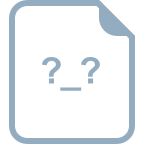