python游戏代码五子棋
时间: 2023-10-05 09:02:44 浏览: 97
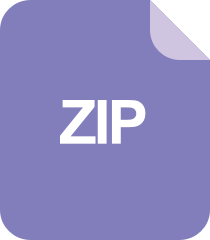
python五子棋代码
五子棋是一种非常经典的棋类游戏,它是基于策略和判断的。下面是一个用Python编写的简单五子棋游戏代码:
```python
# 导入必要的模块
import numpy as np
# 创建一个15x15的棋盘
board = np.zeros((15, 15), dtype=int)
# 定义两个玩家的棋子表示
player1 = 1
player2 = 2
# 开始游戏
def play_game():
current_player = player1
while True:
move = get_move(current_player)
if is_valid_move(move):
place_move(move, current_player)
print_board()
if is_winner(move, current_player):
print(f"玩家 {current_player} 胜利!")
break
elif np.count_nonzero(board) == 15*15:
print("平局!")
break
else:
current_player = player2 if current_player == player1 else player1
else:
print("无效的移动,请重试。")
# 获取玩家输入的移动坐标
def get_move(player):
x = int(input(f"玩家 {player},请输入要下棋的 x 坐标(0-14):"))
y = int(input(f"玩家 {player},请输入要下棋的 y 坐标(0-14):"))
return (x, y)
# 判断移动是否有效
def is_valid_move(move):
if board[move] == 0:
return True
return False
# 落子
def place_move(move, player):
board[move] = player
# 判断是否有玩家胜利
def is_winner(move, player):
x, y = move
# 横向
if x + 4 < 15 and np.all(board[x:x+5, y] == player):
return True
# 纵向
if y + 4 < 15 and np.all(board[x, y:y+5] == player):
return True
# 正斜向
if x + 4 < 15 and y + 4 < 15 and np.all(np.diag(board[x:x+5, y:y+5]) == player):
return True
# 反斜向
if x - 4 >= 0 and y + 4 < 15 and np.all(np.diag(np.fliplr(board[x-4:x+1, y:y+5])) == player):
return True
return False
# 打印当前棋盘状态
def print_board():
row_numbers = [str(i).zfill(2) for i in range(15)]
col_numbers = [str(i).zfill(2) for i in range(15)]
print(" " + " ".join(col_numbers))
for i, row in enumerate(board):
print(row_numbers[i] + " " + " ".join([str(cell).zfill(2) for cell in row]))
# 运行游戏
play_game()
```
以上是一个简单的五子棋游戏代码,通过逐步判断落子的位置是否满足胜利条件来判断游戏胜负。玩家可以通过输入坐标的方式下棋,棋盘上1表示玩家1的棋子,2表示玩家2的棋子。每次落子后都会更新棋盘状态并打印出来,直到有玩家胜利或棋盘满了为止。
阅读全文
相关推荐

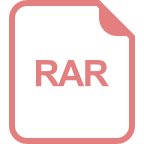
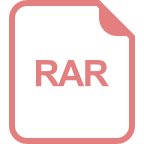
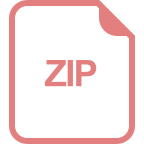
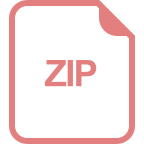








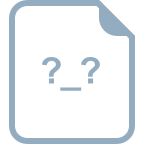