基于tensorflow2.0在VGG16中添加SENet进行人脸表情识别代码
时间: 2024-05-13 09:16:53 浏览: 11
下面是基于TensorFlow 2.0和VGG16模型添加SENet进行人脸表情识别的示例代码:
```python
import tensorflow as tf
from tensorflow.keras.layers import Input, Conv2D, MaxPooling2D, Flatten, Dense, Dropout, GlobalAveragePooling2D, Reshape, Multiply, Add
from tensorflow.keras.models import Model
from tensorflow.keras.applications.vgg16 import VGG16
# 定义SE模块
def se_module(input_feature, ratio=8):
channel = input_feature.shape[-1]
gap = GlobalAveragePooling2D()(input_feature)
fc1 = Dense(channel // ratio, activation='relu')(gap)
fc2 = Dense(channel, activation='sigmoid')(fc1)
fc2 = Reshape((1, 1, channel))(fc2)
return Multiply()([input_feature, fc2])
# 加载VGG16模型
base_model = VGG16(weights='imagenet', include_top=False, input_shape=(48, 48, 3))
for layer in base_model.layers:
layer.trainable = False
# 添加SE模块
x = base_model.output
x = se_module(x)
x = Flatten()(x)
x = Dense(128, activation='relu')(x)
x = Dropout(0.5)(x)
predictions = Dense(7, activation='softmax')(x)
model = Model(inputs=base_model.input, outputs=predictions)
# 编译模型
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# 训练模型
model.fit(train_generator, epochs=10, validation_data=val_generator)
```
其中,`se_module`函数定义了SE模块,`ratio`参数用于控制FC层输出通道数与输入通道数的比例,默认为8。
在加载VGG16模型后,通过调用`base_model.output`获取模型输出,然后将输出作为SE模块的输入,并将SE模块输出后的结果通过Flatten层压平,接着添加一个全连接层、一个Dropout层和一个Softmax层,最终构建出完整的SENet-VGG16模型,并使用`model.compile`编译模型,使用`model.fit`训练模型。
需要注意的是,本示例代码中的`train_generator`和`val_generator`需要根据具体情况进行替换,以适配训练数据的输入形式和标签形式。
相关推荐
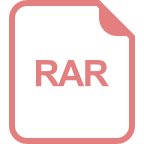
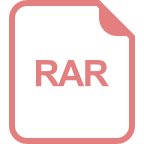
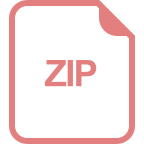














