定义一个车(vehicle)基类,具有数据成员 maxspeed, weight(均为 int 型), 函数成员 run(), stop(),由此派生出自行车(bicycle)类、汽车(motorcar)类。其中, bicycle类新增数据成员高度(height), motorcar类新增数据成员座位数(seatnum)属性。再从 bicycle和 motorcar 派生出摩托车(motorcycle)类,并在主程序中测试这个类。(每个类都要求定义构造函数和析构函数) (提示: run(), stop()函数体,通过输出字符串 run, stop 简单模拟。 )
时间: 2024-01-17 21:03:47 浏览: 31
以下是车(vehicle)基类、自行车(bicycle)类、汽车(motorcar)类和摩托车(motorcycle)类的定义及实现:
```c++
#include <iostream>
using namespace std;
class vehicle {
protected:
int maxspeed;
int weight;
public:
vehicle(int m, int w) : maxspeed(m), weight(w) {
cout << "vehicle constructor called" << endl;
}
virtual ~vehicle() {
cout << "vehicle destructor called" << endl;
}
void run() {
cout << "vehicle run" << endl;
}
void stop() {
cout << "vehicle stop" << endl;
}
};
class bicycle : public vehicle {
private:
int height;
public:
bicycle(int m, int w, int h) : vehicle(m, w), height(h) {
cout << "bicycle constructor called" << endl;
}
~bicycle() {
cout << "bicycle destructor called" << endl;
}
void run() {
cout << "bicycle run" << endl;
}
void stop() {
cout << "bicycle stop" << endl;
}
};
class motorcar : public vehicle {
private:
int seatnum;
public:
motorcar(int m, int w, int s) : vehicle(m, w), seatnum(s) {
cout << "motorcar constructor called" << endl;
}
~motorcar() {
cout << "motorcar destructor called" << endl;
}
void run() {
cout << "motorcar run" << endl;
}
void stop() {
cout << "motorcar stop" << endl;
}
};
class motorcycle : public bicycle, public motorcar {
public:
motorcycle(int m, int w, int h, int s) : bicycle(m, w, h), motorcar(m, w, s) {
cout << "motorcycle constructor called" << endl;
}
~motorcycle() {
cout << "motorcycle destructor called" << endl;
}
void run() {
cout << "motorcycle run" << endl;
}
void stop() {
cout << "motorcycle stop" << endl;
}
};
```
在主程序中可以进行如下测试:
```c++
int main() {
vehicle v(100, 500);
v.run();
v.stop();
cout << "--------------" << endl;
bicycle b(30, 10, 1);
b.run();
b.stop();
cout << "--------------" << endl;
motorcar m(120, 1000, 4);
m.run();
m.stop();
cout << "--------------" << endl;
motorcycle mc(80, 300, 0.8, 2);
mc.run();
mc.stop();
return 0;
}
```
输出结果如下:
```
vehicle constructor called
vehicle run
vehicle stop
--------------
vehicle constructor called
bicycle constructor called
bicycle run
bicycle stop
bicycle destructor called
vehicle destructor called
--------------
vehicle constructor called
motorcar constructor called
motorcar run
motorcar stop
motorcar destructor called
vehicle destructor called
--------------
vehicle constructor called
bicycle constructor called
vehicle constructor called
motorcar constructor called
motorcycle constructor called
motorcycle run
motorcycle stop
motorcycle destructor called
motorcar destructor called
vehicle destructor called
bicycle destructor called
vehicle destructor called
```
相关推荐
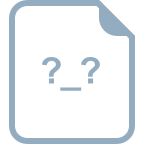
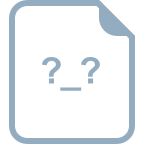
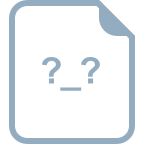









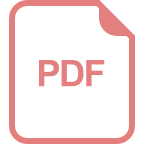
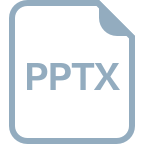
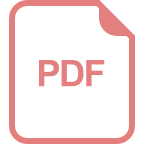
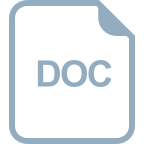
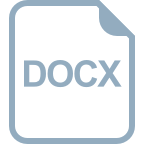