结构体中的指针结构体调用
时间: 2023-12-14 22:33:03 浏览: 28
结构体中的指针结构体调用可以通过C#调用C++的DLL实现。具体步骤如下:
1.在C++中定义结构体和指针结构体,例如:
```c++
typedef struct _tagPerson
{
char name[20];
int age;
}Person;
typedef struct _tagPersonList
{
Person* pPerson;
int count;
}PersonList;
```
2.在C++中编写导出函数,例如:
```c++
extern "C" __declspec(dllexport) PersonList* GetPersonList()
{
PersonList* pList = new PersonList;
pList->count = 2;
pList->pPerson = new Person[2];
strcpy_s(pList->pPerson[0].name, "Tom");
pList->pPerson[0].age = 20;
strcpy_s(pList->pPerson[1].name, "Jerry");
pList->pPerson[1].age = 22;
return pList;
}
```
3.在C#中定义结构体和指针结构体,例如:
```c#
[StructLayout(LayoutKind.Sequential)]
public struct Person
{
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 20)]
public string name;
public int age;
}
[StructLayout(LayoutKind.Sequential)]
public struct PersonList
{
public IntPtr pPerson;
public int count;
}
```
4.在C#中声明导出函数,例如:
```c#
[DllImport("TestDll.dll", CallingConvention = CallingConvention.Cdecl)]
public static extern IntPtr GetPersonList();
```
5.在C#中调用导出函数,例如:
```c#
IntPtr pList = GetPersonList();
PersonList list = (PersonList)Marshal.PtrToStructure(pList, typeof(PersonList));
Person[] persons = new Person[list.count];IntPtr ptr = list.pPerson;
int size = Marshal.SizeOf(typeof(Person));
for (int i = 0; i < list.count; i++)
{
persons[i] = (Person)Marshal.PtrToStructure(ptr, typeof(Person));
ptr = new IntPtr(ptr.ToInt64() + size);
}
```
以上代码演示了如何在C#中调用C++ DLL中的结构体和指针结构体。需要注意的是,在C#中使用指针时需要使用IntPtr类型,并且需要进行内存管理,避免内存泄漏。
相关推荐
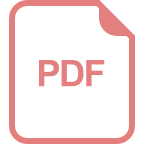














