基追踪问题的增广拉格朗日函数法python
时间: 2023-06-28 13:08:30 浏览: 51
以下是基于增广拉格朗日函数的 Python 代码实现,用于求解约束优化问题:
```python
import numpy as np
from scipy.optimize import minimize
def augmented_lagrangian(fun, x0, eq_cons=[], ineq_cons=[], args=(), bounds=None, maxiter=100, rho=1, epsilon=1e-4):
"""
基于增广拉格朗日函数的约束优化求解器
Args:
fun: 目标函数
x0: 初始解
eq_cons: 等式约束函数列表
ineq_cons: 不等式约束函数列表
args: 传递给目标函数和约束函数的参数元组
bounds: 变量边界
maxiter: 最大迭代次数
rho: 拉格朗日乘子更新系数
epsilon: 更新精度
Returns:
res: 求解结果对象
"""
# 定义拉格朗日乘子更新函数
def update_lambda(lambda_, eq, ineq, rho):
lambda_new = lambda_ + rho * (eq + ineq)
lambda_new[lambda_new < 0] = 0
return lambda_new
# 定义增广拉格朗日函数
def augmented_fun(x, lambda_, rho):
f = fun(x, *args)
eq = np.array([c(x, *args) for c in eq_cons])
ineq = np.array([max(0, c(x, *args)) for c in ineq_cons])
lagrange_term = np.dot(lambda_, eq + ineq)
penalty_term = rho * np.sum(ineq ** 2)
return f + lagrange_term + penalty_term
# 计算约束函数个数
n_eq = len(eq_cons)
n_ineq = len(ineq_cons)
# 初始化拉格朗日乘子和罚因子
lambda_ = np.zeros(n_eq + n_ineq)
rho_ = rho
# 迭代求解
for i in range(maxiter):
# 定义增广拉格朗日函数
aug_fun = lambda x: augmented_fun(x, lambda_, rho_)
# 定义约束条件
constraints = []
if bounds:
constraints.append({'type': 'ineq', 'fun': lambda x: bounds[:, 0] - x})
constraints.append({'type': 'ineq', 'fun': lambda x: x - bounds[:, 1]})
if eq_cons:
constraints.append({'type': 'eq', 'fun': lambda x: np.array([c(x, *args) for c in eq_cons])})
if ineq_cons:
constraints.append({'type': 'ineq', 'fun': lambda x: np.array([c(x, *args) for c in ineq_cons])})
# 求解无约束问题
res = minimize(aug_fun, x0, method='BFGS', jac=False, constraints=constraints)
# 更新拉格朗日乘子和罚因子
lambda_new = update_lambda(lambda_, np.array([c(res.x, *args) for c in eq_cons]), np.array([max(0, c(res.x, *args)) for c in ineq_cons]), rho_)
rho_ *= 2 if np.sum(lambda_new != 0) == n_eq + n_ineq else 0.5
lambda_ = lambda_new
# 判断是否满足精度要求
if np.linalg.norm(np.array([c(res.x, *args) for c in eq_cons]) + np.array([max(0, c(res.x, *args)) for c in ineq_cons])) < epsilon:
break
return res
```
使用示例:
```python
# 定义目标函数和约束函数
def fun(x, a, b):
return (x[0] - a) ** 2 + (x[1] - b) ** 2
def eq_cons(x):
return np.array([x[0] + x[1] - 1])
def ineq_cons(x):
return np.array([x[0], x[1]])
# 设置初始解和变量边界
x0 = np.array([0.5, 0.5])
bounds = np.array([[0, 1], [0, 1]])
# 求解优化问题
res = augmented_lagrangian(fun, x0, eq_cons=[eq_cons], ineq_cons=[ineq_cons], args=(1, 2), bounds=bounds)
print(res)
```
其中,目标函数为 $(x_1-a)^2+(x_2-b)^2$,等式约束为 $x_1+x_2=1$,不等式约束为 $x_1 \ge 0$ 和 $x_2 \ge 0$。
相关推荐
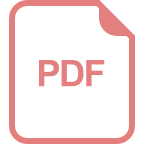











