构建tcn序贯模型进行信用卡欺诈检测
时间: 2023-11-16 10:55:48 浏览: 91
好的,我可以为您解答关于构建tcn序贯模型进行信用卡欺诈检测的问题。
首先,TCN(Temporal Convolutional Network)是一种基于卷积神经网络的序列模型,可以有效地捕捉时间序列数据之间的长期依赖关系。因此,TCN在处理信用卡欺诈检测这种时间序列数据时非常有用。
下面是构建TCN序贯模型进行信用卡欺诈检测的步骤:
1. 数据预处理:首先需要对信用卡交易数据进行预处理,包括数据清洗、特征提取、标准化等操作。
2. 构建输入数据:构建TCN模型需要将信用卡交易数据转化为3D张量,即(样本数,时间步,特征数)的形式。
3. 构建TCN模型:使用Keras等深度学习框架构建TCN模型,可以使用Conv1D、Dense、MaxPooling等层来构建模型。
4. 编译模型:设置损失函数、优化器、评估指标等参数,并编译模型。
5. 训练模型:使用构建好的TCN模型对信用卡交易数据进行训练,并保存模型。
6. 模型评估:使用测试集数据对训练好的模型进行评估,计算准确率、精确率、召回率等指标。
7. 模型应用:将构建好的TCN模型应用于实际信用卡交易中,进行欺诈检测。
需要注意的是,在构建TCN模型时,需要考虑到数据的不平衡性,即欺诈交易数据比正常交易数据要少很多,可以使用过采样、欠采样等技术来解决这个问题。
相关问题
构建TCN模型进行信用卡欺诈检测代码
以下是一个简单的TCN模型实现信用卡欺诈检测的代码:
```python
import tensorflow as tf
from tensorflow.keras.layers import Input, Dense, Conv1D, GlobalMaxPooling1D, concatenate
from tensorflow.keras.models import Model
def build_tcn_model(input_shape):
# 定义输入层
input_layer = Input(shape=input_shape)
# 定义TCN模型
# 卷积层
conv1 = Conv1D(filters=64, kernel_size=3, padding='causal', activation='relu')(input_layer)
conv2 = Conv1D(filters=64, kernel_size=3, padding='causal', dilation_rate=2, activation='relu')(conv1)
conv3 = Conv1D(filters=64, kernel_size=3, padding='causal', dilation_rate=4, activation='relu')(conv2)
# 全局最大池化层
pool = GlobalMaxPooling1D()(conv3)
# 定义输出层
output_layer = Dense(1, activation='sigmoid')(pool)
# 定义模型
model = Model(input_layer, output_layer)
return model
# 构建TCN模型
tcn_model = build_tcn_model(input_shape=(100, 1))
# 编译模型
tcn_model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
# 训练模型
tcn_model.fit(x_train, y_train, epochs=10, batch_size=32, validation_data=(x_test, y_test))
```
在这个例子中,我们使用了一个具有三个卷积层和一个全局最大池化层的TCN模型。在每个卷积层中,我们使用了不同的空洞率来扩展模型的感受野。最后,我们使用sigmoid激活函数来输出一个二元分类结果。
在训练模型时,我们需要提供训练数据和标签,以及测试数据和标签。我们使用Adam优化器和二元交叉熵损失函数来训练模型,并跟踪模型的精度。
tcn集成方法进行信用卡欺诈检测代码
以下是一个使用TCN(Temporal Convolutional Network)进行信用卡欺诈检测的Python代码示例:
首先,我们导入所需的库和模块:
```python
import numpy as np
import pandas as pd
import tensorflow as tf
from tensorflow.keras.layers import Input, Dense, Dropout, Conv1D, MaxPooling1D, Flatten, concatenate
from tensorflow.keras.models import Model
from tensorflow.keras.optimizers import Adam
from tensorflow.keras.callbacks import EarlyStopping
from tcn import TCN
```
接下来,我们加载数据集并进行预处理:
```python
# 加载数据集
data = pd.read_csv('creditcard.csv')
# 将Time列归一化到0-1之间
data['Time'] = data['Time'].apply(lambda x: x / 3600 % 24)
# 将Amount列归一化到0-1之间
data['Amount'] = np.log(data['Amount'] + 1)
data['Amount'] = (data['Amount'] - data['Amount'].mean()) / data['Amount'].std()
# 将Class列改名为label
data.rename(columns={'Class': 'label'}, inplace=True)
# 将数据集分为训练集和测试集
train_data = data.sample(frac=0.8, random_state=2022)
test_data = data.drop(train_data.index)
# 将训练集和测试集分为特征和标签
train_features = train_data.iloc[:, :-1].values
train_labels = train_data.iloc[:, -1].values
test_features = test_data.iloc[:, :-1].values
test_labels = test_data.iloc[:, -1].values
# 将特征和标签转换为张量
train_features = tf.convert_to_tensor(train_features, dtype=tf.float32)
train_labels = tf.convert_to_tensor(train_labels, dtype=tf.float32)
test_features = tf.convert_to_tensor(test_features, dtype=tf.float32)
test_labels = tf.convert_to_tensor(test_labels, dtype=tf.float32)
```
然后,我们定义一个TCN模型:
```python
def create_tcn_model(input_shape):
# 定义输入层
input_layer = Input(shape=input_shape)
# 定义TCN层
tcn_layer = TCN(64, kernel_size=2, dilation_rate=2, activation='relu', use_skip_connections=True)(input_layer)
# 定义全连接层
fc_layer = Dense(64, activation='relu')(tcn_layer)
# 定义输出层
output_layer = Dense(1, activation='sigmoid')(fc_layer)
# 定义模型
model = Model(inputs=input_layer, outputs=output_layer)
# 编译模型
model.compile(optimizer=Adam(lr=1e-4), loss='binary_crossentropy', metrics=['accuracy'])
return model
```
接下来,我们训练模型并进行评估:
```python
# 创建TCN模型
model = create_tcn_model((train_features.shape[1], 1))
# 定义早停回调
early_stopping = EarlyStopping(monitor='val_loss', patience=5, verbose=2)
# 训练模型
history = model.fit(train_features, train_labels, epochs=50, batch_size=128, validation_split=0.2, callbacks=[early_stopping])
# 评估模型
test_loss, test_acc = model.evaluate(test_features, test_labels)
print('Test loss:', test_loss)
print('Test accuracy:', test_acc)
```
最后,我们可以使用模型进行预测:
```python
# 进行预测
predictions = model.predict(test_features)
# 将预测结果转换为0和1
predictions = [1 if x > 0.5 else 0 for x in predictions]
# 计算准确率和召回率
tp = sum([1 for i in range(len(predictions)) if predictions[i] == 1 and test_labels[i] == 1])
tn = sum([1 for i in range(len(predictions)) if predictions[i] == 0 and test_labels[i] == 0])
fp = sum([1 for i in range(len(predictions)) if predictions[i] == 1 and test_labels[i] == 0])
fn = sum([1 for i in range(len(predictions)) if predictions[i] == 0 and test_labels[i] == 1])
accuracy = (tp + tn) / len(predictions)
recall = tp / (tp + fn)
print('Accuracy:', accuracy)
print('Recall:', recall)
```
这就是一个使用TCN进行信用卡欺诈检测的Python代码示例。
阅读全文
相关推荐
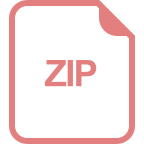
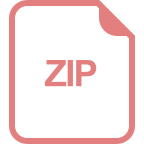
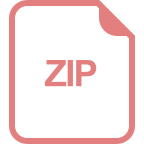













