集成tcn模型进行欺诈检测
时间: 2023-11-16 09:26:08 浏览: 39
集成TCN(Temporal Convolutional Network)模型进行欺诈检测是一种有效的方法。
TCN是一种用于时间序列数据处理的卷积神经网络,它可以学习时间序列数据中的长期依赖关系。在欺诈检测中,我们可以将交易数据看作时间序列数据,并使用TCN模型来学习交易数据中的模式和规律。
具体来说,我们可以将每个交易看作一个时间步,并将交易金额、交易时间、交易地点等作为输入特征。然后,使用多个TCN模型对交易数据进行预测,将它们的输出结果进行集成,得到最终的欺诈检测结果。
集成多个TCN模型可以提高模型的准确性和鲁棒性。我们可以使用不同的超参数、不同的初始化方法、不同的输入特征等来构建不同的TCN模型,然后将它们的输出结果进行加权平均或投票,得到最终的预测结果。
总之,集成TCN模型是一种有效的欺诈检测方法,可以在实际场景中得到广泛应用。
相关问题
集成tcn模型进行欺诈检测代码
以下是一个基于TCN模型进行欺诈检测的代码示例:
首先,我们需要导入必要的库:
```
import numpy as np
import pandas as pd
import tensorflow as tf
from tensorflow.keras.layers import Input, Conv1D, Dense, Dropout, GlobalMaxPooling1D
from tensorflow.keras.models import Model
from tensorflow.keras.optimizers import Adam
from tensorflow.keras.regularizers import l2
from sklearn.model_selection import train_test_split
```
接下来,我们可以加载数据集。假设我们的数据集包含以下特征:
- 时间戳(timestamp)
- 用户ID(user_id)
- 交易金额(transaction_amount)
- 是否欺诈(fraudulent)
```
data = pd.read_csv('fraud_data.csv')
```
我们可以根据需要对数据进行预处理,例如将时间戳转换为数字或进行缩放。然后,我们可以将数据集分成训练集和测试集:
```
X = data[['timestamp', 'user_id', 'transaction_amount']].values
y = data['fraudulent'].values
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
接下来,我们可以构建TCN模型:
```
def build_model(input_shape, num_classes):
input_layer = Input(shape=input_shape)
x = Conv1D(filters=64, kernel_size=3, padding='same', activation='relu', kernel_regularizer=l2(0.001))(input_layer)
x = Dropout(0.2)(x)
x = Conv1D(filters=64, kernel_size=3, padding='same', activation='relu', kernel_regularizer=l2(0.001))(x)
x = Dropout(0.2)(x)
x = Conv1D(filters=64, kernel_size=3, padding='same', activation='relu', kernel_regularizer=l2(0.001))(x)
x = Dropout(0.2)(x)
x = GlobalMaxPooling1D()(x)
x = Dense(128, activation='relu', kernel_regularizer=l2(0.001))(x)
x = Dropout(0.2)(x)
output_layer = Dense(num_classes, activation='sigmoid')(x)
model = Model(inputs=input_layer, outputs=output_layer)
return model
model = build_model(input_shape=(X_train.shape[1], 1), num_classes=1)
model.summary()
```
在这个模型中,我们使用了三个卷积层和一个全局最大池化层,以及一些dropout层和正则化层。最后,我们添加了一个密集层和一个输出层。模型的输出是二进制分类,因此我们使用Sigmoid激活函数。
然后,我们可以编译模型并训练它:
```
model.compile(loss='binary_crossentropy', optimizer=Adam(lr=0.001), metrics=['accuracy'])
history = model.fit(X_train.reshape(-1, X_train.shape[1], 1), y_train,
validation_data=(X_test.reshape(-1, X_test.shape[1], 1), y_test),
epochs=10, batch_size=64)
```
最后,我们可以使用测试集评估模型的性能:
```
score = model.evaluate(X_test.reshape(-1, X_test.shape[1], 1), y_test, verbose=0)
print('Test loss:', score[0])
print('Test accuracy:', score[1])
```
这就是一个基于TCN模型进行欺诈检测的示例代码。
构建TCN模型进行信用卡欺诈检测代码
以下是一个简单的TCN模型实现信用卡欺诈检测的代码:
```python
import tensorflow as tf
from tensorflow.keras.layers import Input, Dense, Conv1D, GlobalMaxPooling1D, concatenate
from tensorflow.keras.models import Model
def build_tcn_model(input_shape):
# 定义输入层
input_layer = Input(shape=input_shape)
# 定义TCN模型
# 卷积层
conv1 = Conv1D(filters=64, kernel_size=3, padding='causal', activation='relu')(input_layer)
conv2 = Conv1D(filters=64, kernel_size=3, padding='causal', dilation_rate=2, activation='relu')(conv1)
conv3 = Conv1D(filters=64, kernel_size=3, padding='causal', dilation_rate=4, activation='relu')(conv2)
# 全局最大池化层
pool = GlobalMaxPooling1D()(conv3)
# 定义输出层
output_layer = Dense(1, activation='sigmoid')(pool)
# 定义模型
model = Model(input_layer, output_layer)
return model
# 构建TCN模型
tcn_model = build_tcn_model(input_shape=(100, 1))
# 编译模型
tcn_model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
# 训练模型
tcn_model.fit(x_train, y_train, epochs=10, batch_size=32, validation_data=(x_test, y_test))
```
在这个例子中,我们使用了一个具有三个卷积层和一个全局最大池化层的TCN模型。在每个卷积层中,我们使用了不同的空洞率来扩展模型的感受野。最后,我们使用sigmoid激活函数来输出一个二元分类结果。
在训练模型时,我们需要提供训练数据和标签,以及测试数据和标签。我们使用Adam优化器和二元交叉熵损失函数来训练模型,并跟踪模型的精度。
相关推荐
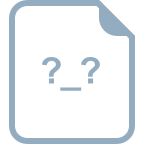












