/ 定义四个方向 int X[4]={0,0,-1,1}; int Y[4]={-1,1,0,0}; void dfs(int nowx,int nowy){ //方法为深度优先搜索,输入当前结点 if(nowx==ex&&nowy==ey){ //若结点为终点,则计数并输出 mycount++; printf("\n第%d条路:\n",mycount); printf("(%d,%d)->",fx,fy); for(int i=0;i<temp.size()-1;i++){ printf("(%d,%d)->",temp[i].x,temp[i].y); } printf("(%d,%d)\n",ex,ey); printf("当前迷宫路径为:\n"); // 定义一个数组记录需要标记的点的位置 bool flag[maxn+1][maxn+1] = {0}; // 对每个邻点进行遍历 for(int i=0;i<temp.size()-1;i++){ int x = temp[i].x; int y = temp[i].y; // 判断下一个坐标的方向,并标记 for(int j=0; j<4; j++){ int newx = x + X[j]; int newy = y + Y[j]; if(newx == temp[i+1].x && newy == temp[i+1].y){ flag[x][y] = true; break; } } } // 遍历整个迷宫,根据需要标记的点的位置进行标记 for(int a = 1; a <= m; a++) { for(int b = 1; b <= n; b++) { if(route[a][b] == 1 || flag[a][b] == 1) { printf("1 "); } else { printf("0 "); } } printf("\n"); } return; } for(int i=0;i<4;i++){ //遍历邻点 int newx=nowx+X[i]; int newy=nowy+Y[i]; if(judge(newx,newy)){ // 访问邻点 temp.push_back(node(newx,newy)); //将邻点设为下一路径结点 ismark[nowx][nowy]=true; //并标记 dfs(newx,newy); temp.pop_back(); //到这一步说明该邻点方案以实现完(或成功或失败) ismark[newx][newy]=false; //取消标记 } } }输出坐标方向与下一个坐标位置并展示代码
时间: 2023-11-24 12:05:12 浏览: 29
这里给出一个可能的实现,其中node为一个简单的结构体,用于存储二维坐标信息:
```c++
#include <iostream>
#include <vector>
using namespace std;
const int maxn = 105;
int m, n, sx, sy, ex, ey;
int route[maxn][maxn], ismark[maxn][maxn];
int X[4] = {0, 0, -1, 1};
int Y[4] = {-1, 1, 0, 0};
int mycount = 0;
struct node {
int x;
int y;
node(int _x, int _y) : x(_x), y(_y) {}
};
vector<node> temp;
bool judge(int x, int y) {
if (x < 1 || x > m || y < 1 || y > n || route[x][y] == 1 || ismark[x][y] == 1) {
return false;
}
return true;
}
void dfs(int nowx, int nowy) {
if (nowx == ex && nowy == ey) {
mycount++;
cout << "\n第" << mycount << "条路:\n";
cout << "(" << sx << "," << sy << ")->";
for (int i = 0; i < temp.size() - 1; i++) {
cout << "(" << temp[i].x << "," << temp[i].y << ")->";
}
cout << "(" << ex << "," << ey << ")\n";
cout << "当前迷宫路径为:\n";
bool flag[maxn][maxn] = {0};
for (int i = 0; i < temp.size() - 1; i++) {
int x = temp[i].x;
int y = temp[i].y;
for (int j = 0; j < 4; j++) {
int newx = x + X[j];
int newy = y + Y[j];
if (newx == temp[i + 1].x && newy == temp[i + 1].y) {
flag[x][y] = true;
break;
}
}
}
for (int a = 1; a <= m; a++) {
for (int b = 1; b <= n; b++) {
if (route[a][b] == 1 || flag[a][b] == 1) {
cout << "1 ";
} else {
cout << "0 ";
}
}
cout << endl;
}
return;
}
for (int i = 0; i < 4; i++) {
int newx = nowx + X[i];
int newy = nowy + Y[i];
if (judge(newx, newy)) {
temp.push_back(node(newx, newy));
ismark[nowx][nowy] = true;
dfs(newx, newy);
temp.pop_back();
ismark[newx][newy] = false;
}
}
}
int main() {
cin >> m >> n;
for (int i = 1; i <= m; i++) {
for (int j = 1; j <= n; j++) {
cin >> route[i][j];
}
}
cin >> sx >> sy >> ex >> ey;
temp.push_back(node(sx, sy));
dfs(sx, sy);
if (mycount == 0) {
cout << "不存在路径" << endl;
}
return 0;
}
```
相关推荐
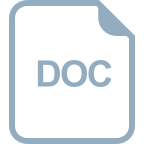
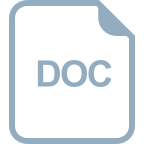
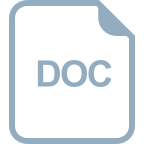















