写一个DDA直线算法,参数有 int x0,int y0,int x1,int y1,TGAImage &image,TGAColor color
时间: 2023-09-18 20:05:35 浏览: 216
### 回答1:
DDA 直线算法是一种基于增量的直线绘制算法,可以通过以下步骤实现:
1. 计算直线的斜率 k = (y1 - y0) / (x1 - x0)。
2. 根据起始点和斜率选择 x 或 y 作为增量变量 delta,并计算步长 step = abs(delta)。
3. 从起始点开始往终点绘制直线,每次增加 delta 并根据斜率计算相应的另一坐标值。
4. 在每个坐标点处绘制像素。
具体实现可以参考下面的代码:
```c++
void drawLine(int x0, int y0, int x1, int y1, TGAImage &image) {
bool steep = false;
if (abs(x1-x0) < abs(y1-y0)) {
std::swap(x0, y0);
std::swap(x1, y1);
steep = true;
}
if (x0 > x1) {
std::swap(x0, x1);
std::swap(y0, y1);
}
float dx = x1 - x0;
float dy = y1 - y0;
float k = dy / dx;
float y = y0;
for (int x = x0; x <= x1; x++) {
if (steep) {
image.set(y, x, red);
} else {
image.set(x, y, red);
}
y += k;
}
}
```
其中,`red` 是用来表示颜色的变量。这个函数接受四个整型参数 `x0`、`y0`、`x1`、`y1` 表示起点和终点的坐标,还有一个 `TGAImage` 类型的引用参数 `image` 表示图像对象。在函数中,首先判断直线是更接近于水平还是竖直方向,并根据需要交换坐标。然后计算直线的斜率和步长,从起点开始按照增量绘制直线,并在每个坐标点处设置颜色。
### 回答2:
DDA直线算法是一种用于在计算机图形学中绘制直线的算法。下面是一个使用DDA算法绘制直线的示例代码:
```c++
#include <iostream>
#include <cmath>
#include "tgaimage.h" // 请根据实际情况引入TGAImage头文件
void drawLineDDA(int x0, int y0, int x1, int y1, TGAImage& image) {
float dx = x1 - x0;
float dy = y1 - y0;
float steps = std::max(std::abs(dx), std::abs(dy)); // 确定步长
float xIncrement = dx / steps; // 计算X轴增量
float yIncrement = dy / steps; // 计算Y轴增量
float x = x0;
float y = y0;
for (int i = 0; i <= steps; i++) {
image.set((int)x, (int)y, TGAColor(255, 255, 255));
x += xIncrement; // 沿X轴方向增量叠加
y += yIncrement; // 沿Y轴方向增量叠加
}
}
int main() {
TGAImage image(800, 600, TGAImage::RGB); // 创建一个800x600的图片
int x0 = 100;
int y0 = 100;
int x1 = 500;
int y1 = 300;
drawLineDDA(x0, y0, x1, y1, image); // 绘制直线
image.flip_vertically(); // 图片翻转
image.write_tga_file("line.tga"); // 将图片保存到文件
return 0;
}
```
在这个例子中,我们使用了一个名为`TGAImage`的图像库的对象来创建一个800x600大小的图片。然后,我们定义了两个点的坐标(称为(x0, y0)和(x1, y1)),并将这些坐标传递给`drawLineDDA`函数,函数将使用DDA算法在图像上绘制一条直线。最后,我们将生成的图片保存到名为`line.tga`的文件中。
请注意,这个例子中使用的是假设`TGAImage`类已经正确实现的图像库。如果您使用其他图像库,请将代码适当地更改为符合您实际情况的库。
### 回答3:
DDA直线算法(Digital Differential Analyzer)是一种用于在计算机图形学中绘制直线的算法。下面是一个用于实现DDA直线算法的函数,该函数接受参数int x0,int y0,int x1,int y1和TGAImage。
```cpp
void drawDDALine(int x0, int y0, int x1, int y1, TGAImage image) {
int deltaX = x1 - x0;
int deltaY = y1 - y0;
int steps = std::max(abs(deltaX), abs(deltaY)); // 计算直线上的点数量
float stepX = (float)deltaX / steps; // 计算x轴方向每个步骤的增量
float stepY = (float)deltaY / steps; // 计算y轴方向每个步骤的增量
float x = x0;
float y = y0;
for (int i = 0; i <= steps; i++) {
// 使用四舍五入将当前点x,y转换为整数
int currentX = (int)(x + 0.5);
int currentY = (int)(y + 0.5);
// 在图像上设置当前点的颜色
image.set(currentX, currentY, color);
// 更新下一个点的位置
x += stepX;
y += stepY;
}
}
```
在这个函数中,我们首先计算直线的增量 deltaX 和 deltaY,然后取其中绝对值较大的作为步数 `steps`,即直线上的点的数量。我们还计算出 x 和 y 轴方向每个步骤的增量 `stepX` 和 `stepY`。然后我们使用一个循环,通过逐步增加 x 和 y 的值来遍历直线上的每个点。在每个步骤中,我们将当前点的x和y值四舍五入为最接近的整数,并将该点的颜色设置到图像上。最后,我们得到了一条直线在图像上的绘制效果。
请注意,上述代码中的 `color` 是我们自定义的颜色变量,你可以根据实际情况进行适当的更改。此外,`TGAImage` 是一个用于创建和操作图像的库,你需要使用该库提供的相应函数来创建和显示图像。
阅读全文
相关推荐
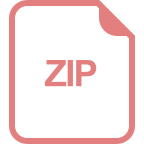
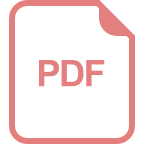
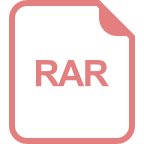















