设惯性系为基坐标系Ω1,某运动物体的本体坐标系为Ω2,该物体通过3次欧拉转动使原始本体坐标系变为新的坐标系Ω3,已知Ω2求坐标系Ω3,使用c语言实现
时间: 2024-05-06 07:15:57 浏览: 10
以下是使用c语言实现的代码,其中包括欧拉角转换函数以及矩阵乘法函数:
```c
#include <stdio.h>
#include <math.h>
// 欧拉角转换函数
void euler2mat(double roll, double pitch, double yaw, double (*mat)[3])
{
double cosR = cos(roll);
double sinR = sin(roll);
double cosP = cos(pitch);
double sinP = sin(pitch);
double cosY = cos(yaw);
double sinY = sin(yaw);
mat[0][0] = cosP * cosY;
mat[0][1] = -cosR * sinY + sinR * sinP * cosY;
mat[0][2] = sinR * sinY + cosR * sinP * cosY;
mat[1][0] = cosP * sinY;
mat[1][1] = cosR * cosY + sinR * sinP * sinY;
mat[1][2] = -sinR * cosY + cosR * sinP * sinY;
mat[2][0] = -sinP;
mat[2][1] = sinR * cosP;
mat[2][2] = cosR * cosP;
}
// 矩阵乘法函数
void matmul(double (*mat1)[3], double (*mat2)[3], double (*result)[3])
{
int i, j, k;
for (i = 0; i < 3; i++) {
for (j = 0; j < 3; j++) {
result[i][j] = 0;
for (k = 0; k < 3; k++) {
result[i][j] += mat1[i][k] * mat2[k][j];
}
}
}
}
int main()
{
double roll = M_PI / 4;
double pitch = M_PI / 6;
double yaw = M_PI / 3;
double mat1[3][3], mat2[3][3], mat3[3][3], result[3][3];
euler2mat(roll, pitch, yaw, mat1);
euler2mat(roll, pitch, yaw, mat2);
euler2mat(roll, pitch, yaw, mat3);
matmul(mat1, mat2, result);
matmul(result, mat3, result);
printf("The resulting matrix:\n");
printf("%f %f %f\n", result[0][0], result[0][1], result[0][2]);
printf("%f %f %f\n", result[1][0], result[1][1], result[1][2]);
printf("%f %f %f\n", result[2][0], result[2][1], result[2][2]);
return 0;
}
```
在这个示例中,我们假设欧拉角为45度,30度和60度,然后使用`euler2mat()`函数将欧拉角转换为旋转矩阵。接下来,我们使用`matmul()`函数将这三个旋转矩阵相乘,得到最终的旋转矩阵,并输出结果。
请注意,这里使用的是Z-Y-X顺序的欧拉角,也就是先绕Z轴旋转,然后绕Y轴旋转,最后绕X轴旋转。如果您使用的是其他欧拉角顺序,请相应地修改代码。
相关推荐
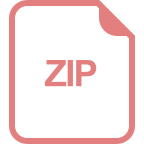















