C语言实现链栈
时间: 2023-08-20 21:13:29 浏览: 101
下是C语言实现链栈的基本代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node *next;
} Node;
typedef struct stack {
Node *top;
} Stack;
Stack *init_stack() {
Stack *s = (Stack *) malloc(sizeof(Stack));
s->top = NULL;
return s;
}
int is_empty(Stack *s) {
return s->top == NULL;
}
void push(Stack *s, int data) {
Node *new_node = (Node *) malloc(sizeof(Node));
new_node->data = data;
new_node->next = s->top;
s->top = new_node;
}
int pop(Stack *s) {
if (is_empty(s)) {
printf("stack is empty\n");
return -1;
}
Node *node = s->top;
int data = node->data;
s->top = node->next;
free(node);
return data;
}
int main() {
Stack *s = init_stack();
push(s, 1);
push(s, 2);
push(s, 3);
while (!is_empty(s)) {
printf("%d ", pop(s));
}
printf("\n");
free(s);
return 0;
}
```
上述代码定义了一个链栈的结构体,包含一个指向栈顶的指针。链栈的基本操作包括初始化栈、判断栈是否为空、入栈、出栈等。其中,入栈和出栈的实现是通过在链表头部插入和删除节点来完成的。
阅读全文
相关推荐
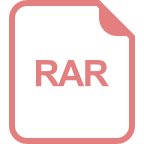














