用python设计一个贪吃蛇游戏,贪吃蛇可以掉头,也可以斜着走
时间: 2024-05-08 13:16:54 浏览: 10
以下是一个基本的贪吃蛇游戏,可以掉头和斜着走。使用Python的turtle模块进行绘图。
```python
import turtle
import random
# 设置窗口大小
screen = turtle.Screen()
screen.setup(600, 600)
screen.bgcolor('black')
screen.title('贪吃蛇')
# 创建蛇头及身体列表
head = turtle.Turtle()
head.shape('square')
head.color('green')
head.penup()
head.speed(0)
head.goto(0, 0)
head.direction = 'stop'
body = []
# 创建食物
food = turtle.Turtle()
food.shape('circle')
food.color('red')
food.penup()
food.speed(0)
food.goto(random.randint(-280, 280), random.randint(-280, 280))
# 创建分数
score = 0
score_pen = turtle.Turtle()
score_pen.speed(0)
score_pen.color('white')
score_pen.penup()
score_pen.hideturtle()
score_pen.goto(0, 260)
score_pen.write('Score: {}'.format(score), align='center', font=('Courier', 24, 'normal'))
# 定义移动函数
def move():
if head.direction == 'up':
y = head.ycor()
head.sety(y + 20)
elif head.direction == 'down':
y = head.ycor()
head.sety(y - 20)
elif head.direction == 'right':
x = head.xcor()
head.setx(x + 20)
elif head.direction == 'left':
x = head.xcor()
head.setx(x - 20)
elif head.direction == 'upright':
x = head.xcor()
y = head.ycor()
head.goto(x + 20, y + 20)
elif head.direction == 'upleft':
x = head.xcor()
y = head.ycor()
head.goto(x - 20, y + 20)
elif head.direction == 'downright':
x = head.xcor()
y = head.ycor()
head.goto(x + 20, y - 20)
elif head.direction == 'downleft':
x = head.xcor()
y = head.ycor()
head.goto(x - 20, y - 20)
# 定义移动方向函数
def go_up():
if head.direction != 'down':
head.direction = 'up'
def go_down():
if head.direction != 'up':
head.direction = 'down'
def go_right():
if head.direction != 'left':
head.direction = 'right'
def go_left():
if head.direction != 'right':
head.direction = 'left'
def go_upright():
if head.direction != 'downleft':
head.direction = 'upright'
def go_upleft():
if head.direction != 'downright':
head.direction = 'upleft'
def go_downright():
if head.direction != 'upleft':
head.direction = 'downright'
def go_downleft():
if head.direction != 'upright':
head.direction = 'downleft'
# 监听键盘事件
screen.listen()
screen.onkeypress(go_up, 'Up')
screen.onkeypress(go_down, 'Down')
screen.onkeypress(go_right, 'Right')
screen.onkeypress(go_left, 'Left')
screen.onkeypress(go_upright, 'w')
screen.onkeypress(go_upleft, 'q')
screen.onkeypress(go_downright, 'x')
screen.onkeypress(go_downleft, 'z')
# 游戏循环
while True:
screen.update()
# 判断是否吃到食物
if head.distance(food) < 20:
food.goto(random.randint(-280, 280), random.randint(-280, 280))
# 添加新的身体
new_body = turtle.Turtle()
new_body.shape('square')
new_body.color('light green')
new_body.penup()
new_body.speed(0)
body.append(new_body)
# 增加分数
score += 10
score_pen.clear()
score_pen.write('Score: {}'.format(score), align='center', font=('Courier', 24, 'normal'))
# 移动身体
for i in range(len(body) - 1, 0, -1):
x = body[i - 1].xcor()
y = body[i - 1].ycor()
body[i].goto(x, y)
if len(body) > 0:
x = head.xcor()
y = head.ycor()
body[0].goto(x, y)
# 判断是否碰到墙壁或者身体
if head.xcor() > 290 or head.xcor() < -290 or head.ycor() > 290 or head.ycor() < -290:
score_pen.clear()
score_pen.write('Game Over', align='center', font=('Courier', 24, 'normal'))
break
for b in body:
if b.distance(head) < 20:
score_pen.clear()
score_pen.write('Game Over', align='center', font=('Courier', 24, 'normal'))
break
# 移动蛇头
move()
turtle.done()
```
运行代码,即可开始游戏。按上下左右和斜向的w、q、x、z键控制蛇的移动方向。吃到食物会增加分数,碰到墙壁或蛇身会游戏结束。
相关推荐
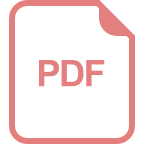
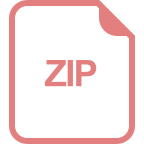
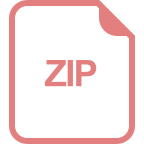












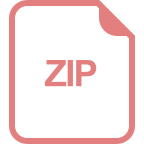
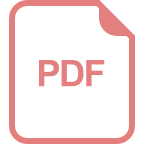