已知平面上两 点的极坐标值,且其中极角的单位是度,编写程序计算这两点的距离
时间: 2024-09-27 09:08:55 浏览: 91
当需要计算两个在极坐标系下表示的点之间的距离时,可以使用以下公式:
\[ d = r_1 \cdot \sin(\theta_2 - \theta_1) \]
其中:
- \( d \) 表示两点间的距离,
- \( r_1 \) 和 \( r_2 \) 分别是两个点的极径(半径),
- \( \theta_1 \) 和 \( \theta_2 \) 是对应点的极角(角度),注意这里是用弧度制来计算距离,因为数学上的距离运算通常基于弧度。
如果你有两个点 A(r1, θ1) 和 B(r2, θ2),首先你需要将它们从度转换成弧度,然后代入上述公式计算。以下是Python的一个简单示例:
```python
import math
def polar_distance(deg_theta1, deg_r1, deg_theta2, deg_r2):
# 将角度从度转换为弧度
rad_theta1 = math.radians(deg_theta1)
rad_theta2 = math.radians(deg_theta2)
# 计算两点距离
distance = abs(deg_r1 * math.sin(rad_theta2 - rad_theta1))
return distance
# 使用示例
distance_AB = polar_distance(45, 5, 60, 7) # 假设点A(5, 45°) 和点B(7, 60°)
```
相关问题
已知平面上两 点的极坐标值,且其中极角的单位是度,编写C程序计算这两点的距离
在C语言中,我们可以利用两点之间的距离公式来计算给定极坐标的两点间距离。对于极坐标,我们通常有两个值:径向距离(r)和极角(θ,以度为单位)。两点之间的距离(d)可以用下面的公式计算:
\[ d = r_1 \cdot \cos(\frac{\theta_2 - \theta_1}{180} \cdot \pi) + r_2 \cdot \sin(\frac{\theta_2 - \theta_1}{180} \cdot \pi) \]
其中 \( r_1 \) 和 \( r_2 \) 分别是两个点的径向距离,\( \theta_1 \) 和 \( \theta_2 \) 是它们的极角。
以下是一个简单的C程序示例,假设输入已经通过`scanf`函数获取,并存储在变量`r1`, `theta1`, `r2`, `theta2`中:
```c
#include <stdio.h>
#include <math.h>
double calculate_distance(double r1, double theta1, double r2, double theta2) {
// 将角度从度转换为弧度
double angle_diff_radians = ((theta2 - theta1) / 180) * M_PI;
// 使用公式计算距离
return sqrt(r1 * r1 * cos(angle_diff_radians)^2 + r2 * r2 * sin(angle_diff_radians)^2);
}
int main() {
double r1, theta1, r2, theta2;
printf("请输入第一个点的径向距离和极角(单位:度): ");
scanf("%lf %lf", &r1, &theta1);
scanf("%lf %lf", &r2, &theta2);
double distance = calculate_distance(r1, theta1, r2, theta2);
printf("两点间的距离为: %.2f\n", distance);
return 0;
}
```
在这个程序中,首先将极角转换为弧度,然后应用距离公式计算结果并返回。运行这个程序后,按照提示输入两点的极坐标即可得到它们之间的距离。
已知平面上两点的极坐标值,其中极角的单位是度编写程序计算,这两点之间的距离要求所有的实数使用double类型,若需要则圆周率的近似值为3.141592653589793
为了编写计算两点之间极坐标距离的程序,你可以按照以下步骤设计:
1. 首先,你需要从用户输入或其他数据源获取两个点的极坐标,每个点由半径 r 和极角 θ 组成,这里需要注意的是,极角是从0度开始,并且通常以弧度计算。由于题目提到极角单位是度,你需要将其转换为弧度,因为许多数学库(如 C++ 的 std::acos 或者 Python 的 math.acos 等)期望的是弧度作为输入。
```cpp
// 使用 C++ 示例
double convertDegreesToRadians(double degrees) {
return degrees * M_PI / 180.0;
}
double radius1, angle1_degrees, radius2, angle2_degrees; // 用户输入或已知值
angle1_degrees = ...; // 两点的极角(单位:度)
angle2_degrees = ...;
radius1 = ...;
angle1_radians = convertDegreesToRadians(angle1_degrees);
radius2 = ...;
angle2_radians = convertDegreesToRadians(angle2_degrees);
```
2. 接下来,计算两点的欧氏距离。假设 A(x1, y1) 和 B(x2, y2) 分别是两点的直角坐标,则它们的关系为:
- x1 = radius1 * cos(angle1_radians)
- y1 = radius1 * sin(angle1_radians)
- x2 = radius2 * cos(angle2_radians)
- y2 = radius2 * sin(angle2_radians)
然后使用欧氏公式 `d = sqrt((x2-x1)^2 + (y2-y1)^2)` 来计算距离。
3. 最后,将结果存储在一个 `double` 类型变量中并返回给用户。
以下是 C++ 示例代码片段:
```cpp
#include <cmath>
using namespace std;
double distanceInPolar(double radius1, double angle1_rad, double radius2, double angle2_rad) {
double dx = radius2 * cos(angle2_rad) - radius1 * cos(angle1_rad);
double dy = radius2 * sin(angle2_rad) - radius1 * sin(angle1_rad);
return hypot(dx, dy); // 使用hypot函数避免多次平方
}
double result = distanceInPolar(radius1, angle1_radians, radius2, angle2_radians);
cout << "两点之间的距离为:" << result << endl;
```
阅读全文
相关推荐
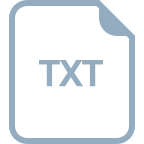
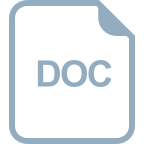

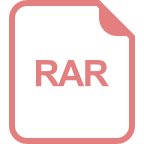
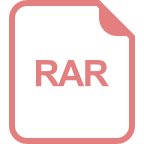
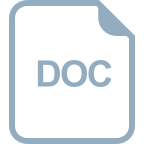

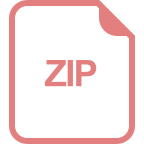