c++ class Circle
时间: 2024-09-21 09:05:39 浏览: 49
在C++中,`Circle`是一个常见的用于表示圆形的对象,它通常会被设计成一个类。下面是一个基础版的`Circle`类的例子,这个类包含了圆的基本属性(如半径`radius`)以及一些基本的操作(如计算周长和面积):
```cpp
class Circle {
public:
// 构造函数,初始化半径
Circle(double r) : radius(r) {}
// 数据成员:圆的半径
double radius;
// 成员函数:获取圆的周长
double getPerimeter() const {
return 2 * M_PI * radius;
}
// 成员函数:获取圆的面积
double getArea() const {
return M_PI * pow(radius, 2);
}
private:
// 私有变量,保护半径不被外部直接修改
double _radius; // 使用下划线前缀防止名称冲突
// 计算圆周率的辅助方法(可以优化为静态全局常量)
static double calculatePi() {
return 3.14159; // 这里仅作为示例,实际应用中可能需要更精确的值
}
};
相关问题
C++抽象类
C++中抽象类是指包含纯虚函数的类,它不能被实例化,只能作为其他类的基类来派生新的类。纯虚函数是在基类中声明的虚函数,但没有给出实现,派生类必须重写这些纯虚函数才能被实例化。
抽象类的声明方式为在类名后面加上 "= 0" 的纯虚函数,例如:
```c++
class Shape {
public:
virtual void draw() = 0; // 纯虚函数
};
```
这里声明了一个名为Shape的抽象类,它包含一个纯虚函数draw(),没有函数体。
派生类可以继承抽象类,但必须实现所有的纯虚函数才能被实例化。例如:
```c++
class Circle : public Shape {
public:
void draw() {
// 实现绘制圆形的代码
}
};
```
这里声明了一个名为Circle的类,它继承自Shape抽象类,并且实现了draw()函数。
抽象类的目的是为了让继承它的类实现某些基本的功能,而不用在每个派生类中都重复实现。
c++ 编程 Define a class Circle and derive the class Cylinder from the class Circle. The specific requirements are as follows: In the main function, input the radius of the circle and output the area of the corresponding circle. Then, input the height of the cylinder, output the surface area and volume of the corresponding cylinder.
下面是一个符合您要求的 C++ 代码实现:
```cpp
#include<iostream>
using namespace std;
class Circle {
protected:
double radius;
public:
Circle(double r) : radius(r) {}
double getArea() const {
return 3.14 * radius * radius;
}
};
class Cylinder : public Circle {
private:
double height;
public:
Cylinder(double r, double h) : Circle(r), height(h) {}
double getSurfaceArea() const {
return 2 * 3.14 * radius * height + 2 * Circle::getArea();
}
double getVolume() const {
return Circle::getArea() * height;
}
};
int main() {
double r, h;
cout << "Please input the radius of the circle: ";
cin >> r;
Circle c(r);
cout << "The area of the corresponding circle is: " << c.getArea() << endl;
cout << "Please input the height of the cylinder: ";
cin >> h;
Cylinder cy(r, h);
cout << "The surface area of the corresponding cylinder is: " << cy.getSurfaceArea() << endl;
cout << "The volume of the corresponding cylinder is: " << cy.getVolume() << endl;
return 0;
}
```
在这个代码实现中,我们定义了一个圆形类 Circle,它有一个受保护的成员变量 radius 和一个公有函数 getArea(),返回圆形的面积。然后我们从 Circle 类派生出一个圆柱体类 Cylinder,它有一个私有成员变量 height 和两个公有函数 getSurfaceArea() 和 getVolume(),分别返回圆柱体的表面积和体积。在 main() 函数中,我们首先输入圆形的半径,创建一个 Circle 对象并输出其面积。然后输入圆柱体的高度,创建一个 Cylinder 对象并输出其表面积和体积。
阅读全文
相关推荐
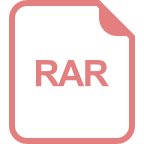
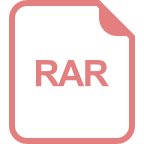
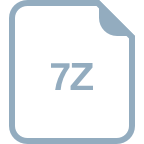













