easyx柱状图移动排序插入
时间: 2023-11-17 10:06:45 浏览: 101
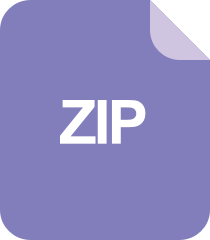
EasyX_Help.zip_EasyX_Help_Help!_easyx图形开发文档_easyx扩展_excited94l
以下是使用easyx库实现柱状图移动排序插入的示例代码:
```c++
#include <graphics.h>
#include <conio.h>
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
const int n = 10; // 柱状图数量
// 柱状图结构体
struct Bar {
int value;
int index;
};
// 比较函数,用于排序
bool cmp(const Bar& a, const Bar& b) {
return a.value < b.value;
}
int main()
{
initgraph(640, 480);
setbkcolor(WHITE);
// 初始化柱状图数组
vector<Bar> bars(n);
for (int i = 0; i < n; i++) {
bars[i].value = rand() % 100;
bars[i].index = i;
}
// 排序柱状图数组
sort(bars.begin(), bars.end(), cmp);
// 绘制柱状图
const int x0 = 100, y0 = 400;
const int width = 40, gap = 20;
for (int i = 0; i < n; i++) {
int x = x0 + i * (width + gap);
int y = y0 - bars[i].value * 2;
setfillcolor(BLUE);
fillrectangle(x, y, x + width, y0);
settextcolor(BLACK);
char str[10];
sprintf_s(str, "%d", bars[i].value);
outtextxy(x, y - 20, str);
}
// 插入新的柱状图
int newValue = rand() % 100;
Bar newBar = { newValue, -1 };
for (int i = 0; i < n; i++) {
if (newValue < bars[i].value) {
bars.insert(bars.begin() + i, newBar);
break;
}
}
if (newBar.index == -1) bars.push_back(newBar);
// 更新柱状图的索引值
for (int i = 0; i < n; i++) {
bars[i].index = i;
}
// 绘制新的柱状图
cleardevice();
for (int i = 0; i < n; i++) {
int x = x0 + i * (width + gap);
int y = y0 - bars[i].value * 2;
setfillcolor(BLUE);
fillrectangle(x, y, x + width, y0);
settextcolor(BLACK);
char str[10];
sprintf_s(str, "%d", bars[i].value);
outtextxy(x, y - 20, str);
}
_getch();
closegraph();
return 0;
}
```
该示例代码使用了结构体来存储每个柱状图的值和索引,然后通过排序函数对柱状图数组进行排序。在插入新的柱状图时,遍历已排序的柱状图数组,找到第一个比新值大的柱状图位置,然后使用 `insert` 函数插入新的柱状图。最后更新所有柱状图的索引值,并重新绘制柱状图。
运行示例代码可以看到,每次运行都会在柱状图中随机插入一个新的柱状图,并且柱状图会按照从小到大的顺序排列。
阅读全文
相关推荐
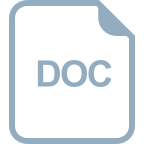
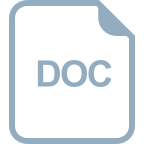















