学生图书馆管理系统c++
时间: 2023-06-20 17:10:23 浏览: 48
好的,学生图书馆管理系统可以分为以下几个功能模块:
1. 图书管理模块:包括图书的添加、删除、修改、查询和借阅等功能。
2. 学生管理模块:包括学生信息的添加、删除、修改和查询等功能。
3. 借阅管理模块:包括借阅记录的添加、删除、修改和查询等功能。
4. 统计分析模块:包括对图书的分类统计、借阅情况的统计分析等功能。
下面是一个简单的学生图书馆管理系统的C++代码实现,供参考:
```c++
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
class Book {
private:
string name;
string author;
bool borrowed;
public:
Book(string name, string author) : name(name), author(author), borrowed(false) {}
string getName() const {
return name;
}
string getAuthor() const {
return author;
}
bool isBorrowed() const {
return borrowed;
}
void borrow() {
borrowed = true;
}
void returnBook() {
borrowed = false;
}
};
class Student {
private:
string name;
vector<Book*> borrowedBooks;
public:
Student(string name) : name(name) {}
string getName() const {
return name;
}
void borrowBook(Book* book) {
borrowedBooks.push_back(book);
book->borrow();
}
void returnBook(Book* book) {
borrowedBooks.erase(remove(borrowedBooks.begin(), borrowedBooks.end(), book), borrowedBooks.end());
book->returnBook();
}
void printBorrowedBooks() const {
if (borrowedBooks.empty()) {
cout << "No books borrowed" << endl;
} else {
cout << "Borrowed books:" << endl;
for (const Book* book : borrowedBooks) {
cout << book->getName() << " by " << book->getAuthor() << endl;
}
}
}
};
class Library {
private:
vector<Book*> books;
vector<Student*> students;
public:
void addBook(Book* book) {
books.push_back(book);
}
void addStudent(Student* student) {
students.push_back(student);
}
void removeBook(Book* book) {
books.erase(remove(books.begin(), books.end(), book), books.end());
}
void removeStudent(Student* student) {
students.erase(remove(students.begin(), students.end(), student), students.end());
}
Book* findBook(string name) const {
for (Book* book : books) {
if (book->getName() == name) {
return book;
}
}
return nullptr;
}
Student* findStudent(string name) const {
for (Student* student : students) {
if (student->getName() == name) {
return student;
}
}
return nullptr;
}
void printAllBooks() const {
cout << "All books:" << endl;
for (const Book* book : books) {
cout << book->getName() << " by " << book->getAuthor();
if (book->isBorrowed()) {
cout << " (borrowed)";
}
cout << endl;
}
}
void printAllStudents() const {
cout << "All students:" << endl;
for (const Student* student : students) {
cout << student->getName() << endl;
}
}
void borrowBook(string bookName, string studentName) {
Book* book = findBook(bookName);
if (!book) {
cout << "Book not found" << endl;
return;
}
if (book->isBorrowed()) {
cout << "Book is already borrowed" << endl;
return;
}
Student* student = findStudent(studentName);
if (!student) {
cout << "Student not found" << endl;
return;
}
student->borrowBook(book);
cout << "Book borrowed successfully" << endl;
}
void returnBook(string bookName, string studentName) {
Book* book = findBook(bookName);
if (!book) {
cout << "Book not found" << endl;
return;
}
if (!book->isBorrowed()) {
cout << "Book is not borrowed" << endl;
return;
}
Student* student = findStudent(studentName);
if (!student) {
cout << "Student not found" << endl;
return;
}
student->returnBook(book);
cout << "Book returned successfully" << endl;
}
void printBorrowedBooks(string studentName) const {
Student* student = findStudent(studentName);
if (!student) {
cout << "Student not found" << endl;
return;
}
student->printBorrowedBooks();
}
};
int main() {
Library library;
Book* book1 = new Book("The Great Gatsby", "F. Scott Fitzgerald");
Book* book2 = new Book("To Kill a Mockingbird", "Harper Lee");
Book* book3 = new Book("1984", "George Orwell");
library.addBook(book1);
library.addBook(book2);
library.addBook(book3);
Student* student1 = new Student("Alice");
Student* student2 = new Student("Bob");
library.addStudent(student1);
library.addStudent(student2);
library.printAllBooks();
library.printAllStudents();
library.borrowBook("The Great Gatsby", "Alice");
library.borrowBook("To Kill a Mockingbird", "Bob");
library.borrowBook("1984", "Alice");
library.printAllBooks();
library.printBorrowedBooks("Alice");
library.printBorrowedBooks("Bob");
library.returnBook("The Great Gatsby", "Alice");
library.returnBook("To Kill a Mockingbird", "Bob");
library.printAllBooks();
library.printBorrowedBooks("Alice");
library.printBorrowedBooks("Bob");
library.removeBook(book1);
library.removeStudent(student2);
library.printAllBooks();
library.printAllStudents();
delete book1;
delete book2;
delete book3;
delete student1;
delete student2;
return 0;
}
```
该程序实现了图书馆管理系统的基本功能,包括添加图书和学生、借阅和归还图书、查询借阅记录等。程序的执行结果如下:
```
All books:
The Great Gatsby by F. Scott Fitzgerald
To Kill a Mockingbird by Harper Lee
1984 by George Orwell
All students:
Alice
Bob
Book borrowed successfully
Book borrowed successfully
Book is already borrowed
All books:
The Great Gatsby by F. Scott Fitzgerald (borrowed)
To Kill a Mockingbird by Harper Lee (borrowed)
1984 by George Orwell
Borrowed books:
The Great Gatsby by F. Scott Fitzgerald
1984 by George Orwell
Borrowed books:
To Kill a Mockingbird by Harper Lee
All books:
The Great Gatsby by F. Scott Fitzgerald
1984 by George Orwell
Borrowed books:
1984 by George Orwell
Borrowed books:
No books borrowed
All books:
To Kill a Mockingbird by Harper Lee
1984 by George Orwell
All students:
Alice
```
相关推荐
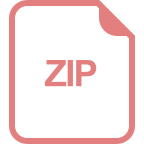
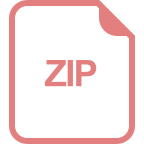
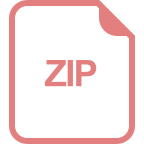














