#include <stdio.h>#include <stdlib.h>#include <string.h>#define MAX_LINE_LEN 1000int main(int argc, char argv[]) { if (argc != 2) { printf("Usage: %s <segment number>\n", argv[0]); exit(1); } int segment_num = atoi(argv[1]); if (segment_num < 1 || segment_num > 5) { printf("Segment number should be between 1 and 5\n"); exit(1); } FILE fp = fopen("dict.dic", "r"); if (fp == NULL) { printf("Failed to open dict.dic\n"); exit(1); } int end_lines[5]; for (int i = 0; i < 5; i++) { fscanf(fp, "%d", &end_lines[i]); } char line[MAX_LINE_LEN]; int cur_line_num = 0; int in_comment = 0; while (fgets(line, MAX_LINE_LEN, fp)) { cur_line_num++; if (cur_line_num > end_lines[segment_num - 1]) { break; } int len = strlen(line); int i = 0; while (i < len) { if (line[i] == '/' && i + 1 < len) { if (line[i + 1] == '/') { if (!in_comment) { printf("\n"); } break; } else if (line[i + 1] == '') { in_comment = 1; i++; } } else if (line[i] == '' && i + 1 < len) { if (line[i + 1] == '/') { in_comment = 0; i++; } } else { if (!in_comment) { printf("%c", line[i]); } } i++; } } fclose(fp); return 0;}运行这段代码,并纠错
时间: 2024-01-14 15:04:26 浏览: 72
这段代码有一些错误,以下是纠错后的代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE_LEN 1000
int main(int argc, char *argv[]) {
if (argc != 2) {
printf("Usage: %s <segment number>\n", argv[0]);
exit(1);
}
int segment_num = atoi(argv[1]);
if (segment_num < 1 || segment_num > 5) {
printf("Segment number should be between 1 and 5\n");
exit(1);
}
FILE *fp = fopen("dict.dic", "r");
if (fp == NULL) {
printf("Failed to open dict.dic\n");
exit(1);
}
int end_lines[5];
for (int i = 0; i < 5; i++) {
fscanf(fp, "%d", &end_lines[i]);
}
char line[MAX_LINE_LEN];
int cur_line_num = 0;
int in_comment = 0;
while (fgets(line, MAX_LINE_LEN, fp)) {
cur_line_num++;
if (cur_line_num > end_lines[segment_num - 1]) {
break;
}
int len = strlen(line);
int i = 0;
while (i < len) {
if (line[i] == '/' && i + 1 < len) {
if (line[i + 1] == '/') {
if (!in_comment) {
printf("\n");
}
break;
} else if (line[i + 1] == '*') {
in_comment = 1;
i++;
}
} else if (line[i] == '*' && i + 1 < len) {
if (line[i + 1] == '/') {
in_comment = 0;
i++;
}
} else {
if (!in_comment) {
printf("%c", line[i]);
}
}
i++;
}
}
fclose(fp);
return 0;
}
```
主要问题如下:
1. `main` 函数声明中的 `char argv[]` 应该改为 `char *argv[]`。
2. 在 `fopen` 函数调用时应该加上 "*",将 `FILE` 类型指针 `fp` 改为 `FILE *fp`。
3. 在处理注释时缺少对 `/*` 和 `*/` 注释的处理,需要增加相关代码。
阅读全文
相关推荐
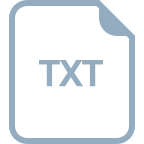
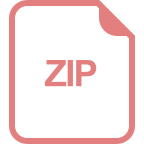

















