batch = src.shape[1]什么意思
时间: 2024-01-11 21:04:32 浏览: 133
这段代码中,src是一个二维数组,shape属性返回该数组的维度信息。由于这里使用了下标1,因此可以得知src数组的第二维中包含了多少个元素,也就是该数组的列数。因此,batch = src.shape[1]的意思是将src数组的列数赋值给batch变量。在机器学习中,通常会使用batch来表示一批数据的大小。
相关问题
解释def train(model, iterator, optimizer, criterion, clip): model.train() epoch_loss = 0 for i, batch in tqdm(enumerate(iterator), total=len(iterator)): src = batch.description trg = batch.diagnosis optimizer.zero_grad() output = model(src, trg) output_dim = output.shape[-1] output = output[1:].view(-1, output_dim) trg = trg[1:].view(-1) loss = criterion(output, trg) loss.backward() torch.nn.utils.clip_grad_norm_(model.parameters(), clip) optimizer.step() epoch_loss += loss.item() return epoch_loss / len(iterator)
这段代码定义了一个训练函数 `train`,用于训练模型。以下是代码的解释:
- `model.train()`:将模型设为训练模式,这会启用模型中的一些特定操作,如 dropout。
- `epoch_loss = 0`:初始化一个变量 `epoch_loss`,用于累积每个 epoch 的损失值。
在接下来的循环中,遍历了数据迭代器 `iterator` 中的每个 batch,并进行以下操作:
- `src = batch.description` 和 `trg = batch.diagnosis`:从当前 batch 中获取描述文本和诊断文本。
- `optimizer.zero_grad()`:将优化器的梯度缓冲区清零,以防止梯度累积。
- `output = model(src, trg)`:使用模型对描述文本进行预测,得到模型的输出。
- `output_dim = output.shape[-1]`:获取模型输出的最后一个维度大小,这对于计算损失函数很重要。
- `output = output[1:].view(-1, output_dim)` 和 `trg = trg[1:].view(-1)`:将模型输出和目标序列都进行裁剪和展平操作,以便计算损失函数。
- `loss = criterion(output, trg)`:计算模型输出和目标序列之间的损失值。
- `loss.backward()`:计算损失关于模型参数的梯度。
- `torch.nn.utils.clip_grad_norm_(model.parameters(), clip)`:对模型的梯度进行裁剪,以防止梯度爆炸问题。
- `optimizer.step()`:更新模型的参数,根据计算得到的梯度和优化器的设置。
- `epoch_loss += loss.item()`:将当前 batch 的损失值添加到 `epoch_loss` 中。
最后,函数返回每个 epoch 的平均损失值,即 `epoch_loss` 除以数据迭代器的长度。
class MemoryEncoding(nn.Module): def __init__(self, in_feats, out_feats, mem_size): #调用 super() 函数来初始化基类 nn.Module,并将输入参数保存为类的属性。 super(MemoryEncoding, self).__init__() self.in_feats = in_feats self.out_feats = out_feats self.mem_size = mem_size self.linear_coef = nn.Linear(in_feats, mem_size, bias=True) self.act = nn.LeakyReLU(0.2, inplace=True) self.linear_w = nn.Linear(mem_size, out_feats * in_feats, bias=False) def get_weight(self, x): coef = self.linear_coef(x) if self.act is not None: coef = self.act(coef) w = self.linear_w(coef) w = w.view(-1, self.out_feats, self.in_feats) return w def forward(self, h_dst, h_src): w = self.get_weight(h_dst) res = torch.einsum('boi, bi -> bo', w, h_src) return res 举个例子
好的,我将给出一个具体的示例,演示如何使用 `MemoryEncoding` 类进行前向传播计算。
```python
import torch
import torch.nn as nn
# 定义输入张量的大小和内存大小
in_feats = 10
out_feats = 5
mem_size = 3
# 创建 MemoryEncoding 实例
memory_encoding = MemoryEncoding(in_feats, out_feats, mem_size)
# 创建示例输入张量
batch_size = 2
dst_feats = in_feats
src_feats = in_feats
h_dst = torch.randn(batch_size, dst_feats)
h_src = torch.randn(batch_size, src_feats)
# 执行前向传播
output = memory_encoding.forward(h_dst, h_src)
print(output.shape) # 输出形状为 (batch_size, out_feats)
```
在这个示例中,我们首先导入了 PyTorch 的 `nn` 模块,并定义了输入张量的大小和内存大小。然后,我们创建了一个 `MemoryEncoding` 类的实例 `memory_encoding`。
接下来,我们使用随机生成的张量 `h_dst` 和 `h_src` 执行前向传播计算,并将结果保存在 `output` 变量中。最后,我们打印出输出张量的形状。
请注意,这只是一个示例,实际使用时需要根据具体问题和数据调整输入张量的大小和值。
阅读全文
相关推荐
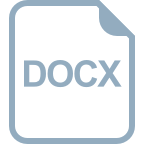
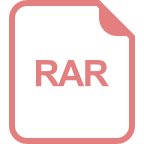
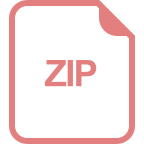






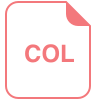
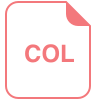
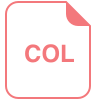




