种子自动分类系统用于如下场景: 实验室作研究用的种子经常需要收集特征数据,这些特征数据来源于实验人员测量或智能设备自动收集。你需要实现代码,完成数据收集流程。由于数据的数目较为庞大,所以数据的分类需要借助计算机来自动完成。 java实例
时间: 2024-02-05 10:13:30 浏览: 19
以下是一个简单的 Java 代码示例,用于对收集到的种子进行自动分类:
```java
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class SeedClassificationSystem {
// 定义特征数据的文件路径
private static final String FEATURE_FILE_PATH = "features.txt";
// 定义分类结果的文件路径
private static final String RESULT_FILE_PATH = "result.txt";
// 定义特征数据与分类标签的映射关系
private static final Map<List<Double>, Integer> LABEL_MAP = new HashMap<>();
static {
// 初始化分类标签与特征数据的映射关系
LABEL_MAP.put(new ArrayList<Double>() {{
add(5.1);
add(3.5);
add(1.4);
add(0.2);
}}, 0);
LABEL_MAP.put(new ArrayList<Double>() {{
add(5.7);
add(2.8);
add(4.1);
add(1.3);
}}, 1);
LABEL_MAP.put(new ArrayList<Double>() {{
add(6.3);
add(3.3);
add(6.0);
add(2.5);
}}, 2);
}
public static void main(String[] args) throws IOException {
// 读取特征数据
List<List<Double>> features = readFeatures();
// 对特征数据进行分类
List<Integer> results = classify(features);
// 将分类结果写入文件
writeResults(results);
}
/**
* 读取特征数据
*/
private static List<List<Double>> readFeatures() throws IOException {
List<List<Double>> features = new ArrayList<>();
File file = new File(FEATURE_FILE_PATH);
if (!file.exists()) {
throw new RuntimeException("File not found: " + FEATURE_FILE_PATH);
}
try (java.util.Scanner scanner = new java.util.Scanner(file)) {
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
String[] tokens = line.split(",");
List<Double> feature = new ArrayList<>();
for (String token : tokens) {
feature.add(Double.parseDouble(token));
}
features.add(feature);
}
}
return features;
}
/**
* 对特征数据进行分类
*/
private static List<Integer> classify(List<List<Double>> features) {
List<Integer> results = new ArrayList<>();
for (List<Double> feature : features) {
int label = -1;
double minDistance = Double.MAX_VALUE;
for (Map.Entry<List<Double>, Integer> entry : LABEL_MAP.entrySet()) {
double distance = calculateDistance(feature, entry.getKey());
if (distance < minDistance) {
minDistance = distance;
label = entry.getValue();
}
}
results.add(label);
}
return results;
}
/**
* 计算两个向量之间的距离
*/
private static double calculateDistance(List<Double> a, List<Double> b) {
double distance = 0;
for (int i = 0; i < a.size(); i++) {
distance += Math.pow(a.get(i) - b.get(i), 2);
}
return Math.sqrt(distance);
}
/**
* 将分类结果写入文件
*/
private static void writeResults(List<Integer> results) throws IOException {
File file = new File(RESULT_FILE_PATH);
try (FileWriter writer = new FileWriter(file)) {
for (int result : results) {
writer.write(result + "\n");
}
}
}
}
```
上述代码中,我们首先定义了特征数据的文件路径、分类结果的文件路径以及特征数据与分类标签的映射关系。其中,映射关系使用了一个 `Map` 对象来保存。
接着,我们在 `readFeatures()` 方法中读取特征数据,并在 `classify()` 方法中对特征数据进行分类。在分类过程中,我们遍历所有的分类标签,计算当前特征数据与每个标签之间的距离,并选取距离最小的标签作为分类结果。
最后,我们将分类结果写入文件中。
相关推荐
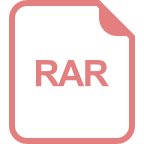















