SpringBoot 整合 elasticsearch 实现增删改查代码怎么写
时间: 2024-04-22 12:26:06 浏览: 26
你可以按照以下步骤使用 Spring Boot 和 Elasticsearch 实现增删改查的功能:
1. 添加依赖:在你的 Spring Boot 项目的 pom.xml 文件中,添加 Elasticsearch 相关的依赖。例如:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-elasticsearch</artifactId>
</dependency>
```
2. 配置 Elasticsearch:在你的 application.properties 或 application.yml 文件中,配置 Elasticsearch 的连接信息。例如:
```yaml
spring.data.elasticsearch.cluster-nodes=localhost:9200
```
3. 创建实体类:创建一个代表你要操作的数据的实体类,并使用注解来映射到 Elasticsearch 中的索引和类型。例如:
```java
import org.springframework.data.annotation.Id;
import org.springframework.data.elasticsearch.annotations.Document;
@Document(indexName = "your_index_name", type = "your_type_name")
public class YourEntity {
@Id
private String id;
private String name;
// 其他属性和方法
}
```
4. 创建数据访问接口:创建一个继承自 ElasticsearchRepository 的数据访问接口,该接口提供了一些基本的 CRUD 方法。例如:
```java
import org.springframework.data.elasticsearch.repository.ElasticsearchRepository;
public interface YourEntityRepository extends ElasticsearchRepository<YourEntity, String> {
// 可以根据需要添加自定义的查询方法
}
```
5. 编写业务逻辑:在你的服务类中使用 YourEntityRepository 来进行相关的数据操作。例如:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class YourService {
@Autowired
private YourEntityRepository repository;
public YourEntity save(YourEntity entity) {
return repository.save(entity);
}
public void delete(String id) {
repository.deleteById(id);
}
public Iterable<YourEntity> findAll() {
return repository.findAll();
}
// 可以根据需要添加其他的查询方法和业务逻辑
}
```
这样,你就可以通过调用 YourService 中的方法来实现对 Elasticsearch 的增删改查操作了。希望对你有帮助!
相关推荐
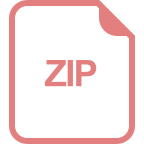
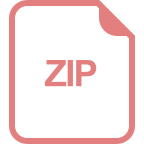
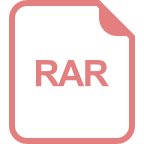
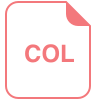
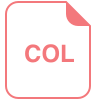
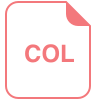
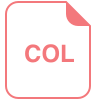
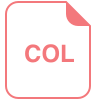









