c++sizeof和strlen区别 并用char[]举例
时间: 2024-12-13 13:18:26 浏览: 9
在C++中,`sizeof` 和 `strlen` 是两个不同的操作符:
1. `sizeof`:这是一个运算符,用于计算某个数据类型或变量在内存中的字节数。它返回的是一个整数,表示指定对象占用的存储空间的大小,以字节为单位。例如,`sizeof(char)` 将返回 1,因为 char 类型通常占一个字节的空间。
```cpp
char str[] = "Hello";
std::cout << "Size of str array: " << sizeof(str); // 输出:Size of str array: 6 (因为字符串常量包括结束符 '\0')
```
这里 `sizeof(str)` 返回整个字符数组(包括空字符'\0')的长度。
2. `strlen`:这是`<cstring>`库中的函数,用于计算给定C风格字符串(以空字符'\0'结尾的字符数组)的实际长度,不包括空字符本身。例如:
```cpp
const char* str = "Hello";
size_t len = strlen(str);
std::cout << "Length of the string: " << len; // 输出:Length of the string: 5
```
`strlen(str)` 计算的是实际有效字符的数量。
相关问题
C++里面文件描述符的使用举例
文件描述符是在Unix、Linux等操作系统中用于表示文件、管道、网络连接等I/O资源的整数。在C++中,可以使用文件描述符进行文件的读写等操作。以下是一个使用文件描述符进行文件读写的示例:
```c++
#include <iostream>
#include <cstdio>
#include <unistd.h>
#include <fcntl.h>
using namespace std;
int main() {
int fd = open("test.txt", O_RDWR | O_CREAT, 0644);
if (fd < 0) {
perror("open");
return -1;
}
char buf[1024] = "Hello, world!";
int len = strlen(buf);
int n = write(fd, buf, len);
if (n != len) {
perror("write");
return -1;
}
lseek(fd, 0, SEEK_SET);
char buf2[1024];
n = read(fd, buf2, sizeof(buf2));
if (n < 0) {
perror("read");
return -1;
}
buf2[n] = '\0';
cout << buf2 << endl;
close(fd);
return 0;
}
```
在上述代码中,我们使用`open`函数打开了一个名为`test.txt`的文件,并指定了`O_RDWR`和`O_CREAT`标志,表示可读可写并且如果文件不存在则创建。`0644`表示文件权限。如果文件打开失败,`open`函数会返回-1。
接着我们使用`write`函数将字符串`"Hello, world!"`写入文件中,并使用`lseek`函数将文件指针移回文件开头。然后我们使用`read`函数读取文件中的内容,并将读取到的内容打印出来。
最后我们使用`close`函数关闭文件描述符。
定时发送心跳包用C++代码举例
以下是一个简单的C++代码示例,用于发送心跳包:
```c++
#include <iostream>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <unistd.h>
#include <string.h>
#include <thread>
#include <chrono>
using namespace std;
void sendHeartbeat(int sockfd, sockaddr_in serverAddr) {
char buffer[1024] = "heartbeat";
while (true) {
int sendResult = sendto(sockfd, buffer, strlen(buffer), 0, (struct sockaddr *) &serverAddr, sizeof(serverAddr));
if (sendResult < 0) {
cout << "Failed to send heartbeat!" << endl;
} else {
cout << "Heartbeat sent successfully!" << endl;
}
std::this_thread::sleep_for(std::chrono::seconds(10));
}
}
int main(int argc, char **argv) {
int sockfd = socket(AF_INET, SOCK_DGRAM, IPPROTO_UDP);
if (sockfd < 0) {
cout << "Failed to create socket!" << endl;
return -1;
}
struct sockaddr_in serverAddr;
memset(&serverAddr, 0, sizeof(serverAddr));
serverAddr.sin_family = AF_INET;
serverAddr.sin_addr.s_addr = inet_addr("127.0.0.1"); // 服务器IP地址
serverAddr.sin_port = htons(8888); // 服务器端口号
std::thread heartBeatThread(sendHeartbeat, sockfd, serverAddr);
heartBeatThread.join();
close(sockfd);
return 0;
}
```
该代码创建了一个UDP socket,然后使用一个独立的线程发送心跳包到指定的服务器IP地址和端口。在发送心跳包之前,线程会暂停10秒钟。
阅读全文
相关推荐
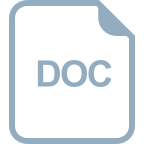
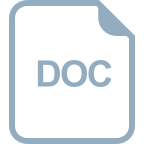
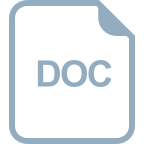
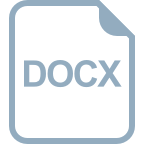
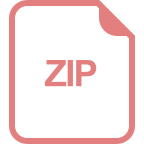
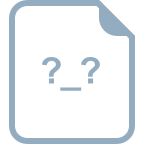
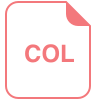
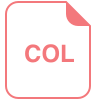






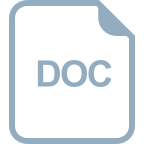
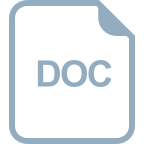
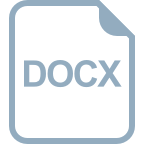