单个结构体成员为1个字符串和1个无符号整数 输入字符串后需要和数组里面每个结构体成员的字符串进行匹配,匹配到后返回对应的无符号整数,如何做查找效率最高。要求,结构体成员个数不确定,可以增加或删除。举例用C语言,不要用C++
时间: 2024-11-05 10:27:36 浏览: 21
在C语言中,由于结构体大小和布局不是固定的,且成员数量可变,直接遍历整个结构体数组并逐个比较可能效率不高。为了提高查找效率,你可以考虑使用哈希表(如`unordered_map`或`hash_table`)来存储字符串及其对应的无符号整数值。这样,通过字符串作为键,可以直接定位到相应的整数值。
下面是实现的一个简单示例,假设我们有一个动态结构体数组`structs`,其中第一个成员是字符串`str`,第二个成员是无符号整数`int_value`:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h> // 使用strlen()获取字符串长度
// 定义结构体
typedef struct {
char *str;
uint32_t int_value;
} MyStruct;
// 哈希表的简化版本,实际应用中可以使用更高效的库
typedef struct HashTableEntry {
char *key; // 字符串
uint32_t value;
struct HashTableEntry* next;
} HashTableEntry;
HashTableEntry* hash_table_entry_new(char* key, uint32_t value) {
HashTableEntry* entry = (HashTableEntry*)malloc(sizeof(HashTableEntry));
entry->key = key;
entry->value = value;
entry->next = NULL;
return entry;
}
// 添加到哈希表
void add_to_hash_table(HashTableEntry** table, char* key, uint32_t value) {
HashTableEntry* entry = *table;
while (entry != NULL) {
if (strcmp(entry->key, key) == 0) {
// 如果已存在,则更新值
entry->value = value;
break;
}
entry = entry->next;
}
if (entry == NULL) {
// 如果不存在,添加新条目
entry = hash_table_entry_new(key, value);
if (*table == NULL) {
*table = entry;
} else {
HashTableEntry* last = *table;
while (last->next != NULL) {
last = last->next;
}
last->next = entry;
}
}
}
// 查找哈希表
uint32_t find_in_hash_table(HashTableEntry* table, const char* key) {
HashTableEntry* entry = table;
while (entry != NULL) {
if (strcmp(entry->key, key) == 0) {
return entry->value;
}
entry = entry->next;
}
return 0; // 如果未找到,返回默认值或抛异常
}
// 示例:输入字符串,查找对应的无符号整数
int main() {
HashTableEntry* hash_table = NULL;
MyStruct structs[] = {{"test", 123}, {"example", 456}};
int num_structs = sizeof(structs) / sizeof(structs[0]);
for (int i = 0; i < num_structs; ++i) {
add_to_hash_table(&hash_table, structs[i].str, structs[i].int_value);
}
const char* input_str = "test";
uint32_t result = find_in_hash_table(hash_table, input_str);
printf("For input '%s', the corresponding integer is %u\n", input_str, result);
return 0;
}
```
阅读全文
相关推荐
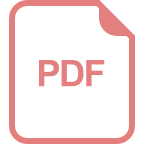
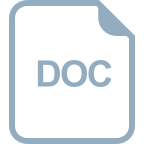
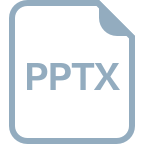


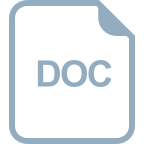
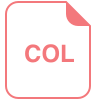
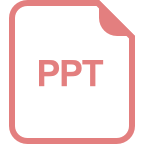
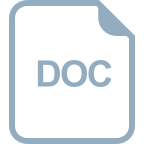
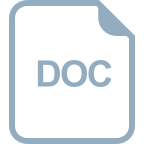
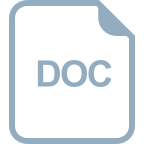
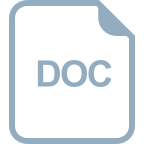
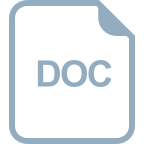
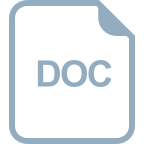
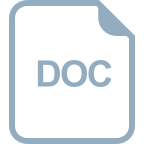
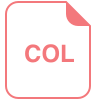
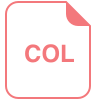
