写出pinn的python代码
时间: 2023-06-05 18:07:00 浏览: 241
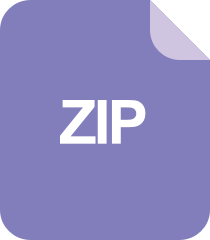
基于PINN物理信息网络结合求解瞬态薛定谔方程(python)

以下是一个简单的 Pinn 的 Python 代码示例:
```python
import numpy as np
import tensorflow as tf
# 定义神经网络结构
class Pinn(tf.keras.models.Model):
def __init__(self, input_dim, hidden_dim, output_dim):
super(Pinn, self).__init__()
self.input_layer = tf.keras.layers.Dense(hidden_dim, activation='relu', input_shape=(input_dim,))
self.hidden_layer = tf.keras.layers.Dense(hidden_dim, activation='relu')
self.output_layer = tf.keras.layers.Dense(output_dim)
def call(self, x):
x = self.input_layer(x)
x = self.hidden_layer(x)
return self.output_layer(x)
# 构建训练数据
n_data = 1000
x_data = np.random.uniform(low=-1.0, high=1.0, size=(n_data, 1))
y_data = np.sin(3 * x_data) + np.random.normal(scale=0.1, size=(n_data, 1))
# 定义损失函数
def pinn_loss(y_true, y_pred):
# y_true: 训练数据标签
# y_pred: 神经网络的输出
# f 和 u 代表一个物理学问题中的函数和解
# 根据物理规律定义的损失函数需要同时考虑真实标签和网络输出
# 比如下面的损失函数定义了一个 Poisson 方程
f = lambda x: -3 * np.sin(3 * x) - 9 * np.cos(3 * x)
def poisson_loss(u_true, u_pred):
h = tf.constant(x_data[1] - x_data[0], dtype=tf.float32)
fu = tf.gradients(u_pred, x)[0] - f(x)
return tf.reduce_mean(tf.square(u_true - u_pred)) + tf.reduce_mean(tf.square(fu))
return poisson_loss(y_data, y_pred)
# 训练神经网络
pinn = Pinn(input_dim=1, hidden_dim=50, output_dim=1)
pinn.compile(optimizer='adam', loss=pinn_loss)
pinn.fit(x_data, y_data, epochs=100)
```
该代码使用 TensorFlow 实现了一个 Pinn 神经网络,并对其进行了训练。Pinn 神经网络在物理学领域中有广泛的应用,能够模拟物理学问题以及解决逆问题。在该示例代码中,我们使用了一个简单的 Poisson 方程作为损失函数的例子,读者可以根据自己的需要进行修改。
阅读全文
相关推荐
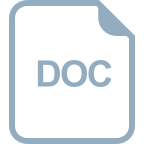
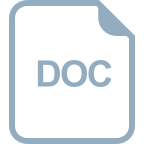



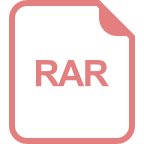
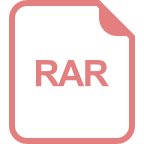
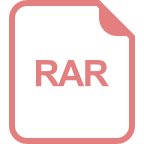









