用cpp语言设计一个多项式相加的程序,要求写出完整的代码,该程序对多项式进行的第一个操作是对数据项中的变量排序,输入多项式之后,每个数据项都分别用排序向量进行排序,链表中的节点可以对该向量进行访问.第二个操作是多项式相加.这个过程是由创建一个链表开始的,这个链表是由待相加的多项式的节点副本组成的,并且最后能够输出多项式相加的值.
时间: 2023-02-07 10:07:12 浏览: 87
下面是使用 C++ 语言设计一个多项式相加的程序的完整代码:
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
struct Node {
// 每个节点存储系数和指数
int coefficient;
int exponent;
// 对指数进行排序
bool operator<(const Node &other) const {
return exponent < other.exponent;
}
};
struct LinkedList {
Node data;
LinkedList *next;
// 创建一个链表节点的构造函数
LinkedList(Node data, LinkedList *next = nullptr) : data(data), next(next) {}
};
// 链表的头指针
LinkedList *head = nullptr;
// 将一个节点插入到链表中
void insert(Node data) {
// 创建一个新的节点
LinkedList *node = new LinkedList(data);
// 将新节点插入到链表的开头
node->next = head;
head = node;
}
// 输出链表中的所有节点
void print() {
// 遍历链表
LinkedList *curr = head;
while (curr) {
// 输出节点的系数和指数
cout << curr->data.coefficient << "x^" << curr->data.exponent << " ";
// 移动到下一个节点
curr = curr->next;
}
cout << endl;
}
// 多项式相加
void add() {
// 遍历链表,将多项式的节点放入一个向量中
vector<Node> nodes;
LinkedList *curr = head;
while (curr) {
nodes.push_back(curr->data);
curr = curr->next;
}
// 对向量中的节点进行排序
sort(nodes.begin(), nodes.end());
// 将排序后的节点放回到链表中
head = nullptr;
for (const Node
阅读全文
相关推荐





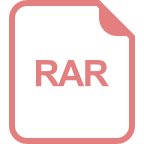
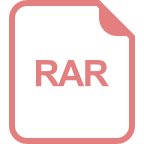


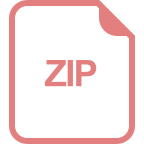
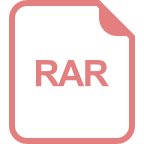
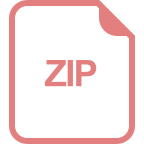
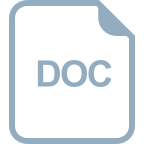
