使用java编写一个breakout游戏
时间: 2023-07-20 15:32:47 浏览: 69
好的,我可以给你提供一些关于使用Java编写breakout游戏的指导。
首先,你需要了解breakout游戏的规则和基本要素。breakout游戏是一种非常简单的街机游戏,玩家需要通过控制一个挡板来反弹球,打掉上方的砖块,最终消除所有砖块才能过关。
下面是一个基本的breakout游戏的代码实现:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Breakout extends JFrame implements ActionListener {
private static final int WIDTH = 800;
private static final int HEIGHT = 600;
private static final int BRICK_WIDTH = 60;
private static final int BRICK_HEIGHT = 20;
private static final int PADDLE_WIDTH = 100;
private static final int PADDLE_HEIGHT = 10;
private static final int BALL_DIAMETER = 20;
private static final int BALL_SPEED = 5;
private static final int DELAY = 10;
private Timer timer;
private Ball ball;
private Paddle paddle;
private Brick[] bricks;
private int score;
private JLabel scoreLabel;
public Breakout() {
setTitle("Breakout");
setSize(WIDTH, HEIGHT);
setDefaultCloseOperation(EXIT_ON_CLOSE);
Container contentPane = getContentPane();
contentPane.setLayout(new BorderLayout());
JPanel gamePanel = new JPanel();
gamePanel.setLayout(null);
contentPane.add(gamePanel, BorderLayout.CENTER);
scoreLabel = new JLabel("Score: " + score);
contentPane.add(scoreLabel, BorderLayout.NORTH);
ball = new Ball((WIDTH - BALL_DIAMETER) / 2, HEIGHT / 2, BALL_DIAMETER, BALL_DIAMETER);
gamePanel.add(ball);
paddle = new Paddle((WIDTH - PADDLE_WIDTH) / 2, HEIGHT - PADDLE_HEIGHT - 20, PADDLE_WIDTH, PADDLE_HEIGHT);
gamePanel.add(paddle);
bricks = new Brick[10];
for (int i = 0; i < bricks.length; i++) {
bricks[i] = new Brick(i * BRICK_WIDTH + 30, 60, BRICK_WIDTH, BRICK_HEIGHT);
gamePanel.add(bricks[i]);
}
timer = new Timer(DELAY, this);
timer.start();
addKeyListener(new KeyAdapter() {
public void keyPressed(KeyEvent e) {
if (e.getKeyCode() == KeyEvent.VK_LEFT) {
paddle.moveLeft();
}
if (e.getKeyCode() == KeyEvent.VK_RIGHT) {
paddle.moveRight();
}
}
});
setFocusable(true);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
ball.move();
if (ball.intersects(paddle)) {
ball.reverseY();
}
for (int i = 0; i < bricks.length; i++) {
if (ball.intersects(bricks[i])) {
bricks[i].setVisible(false);
ball.reverseY();
score += 10;
scoreLabel.setText("Score: " + score);
}
}
if (ball.getY() > HEIGHT) {
timer.stop();
JOptionPane.showMessageDialog(this, "Game over!");
System.exit(0);
}
repaint();
}
public static void main(String[] args) {
new Breakout();
}
}
class Ball extends JComponent {
private int x;
private int y;
private int width;
private int height;
private int dx = BALL_SPEED;
private int dy = BALL_SPEED;
public Ball(int x, int y, int width, int height) {
this.x = x;
this.y = y;
this.width = width;
this.height = height;
}
public void move() {
x += dx;
y += dy;
if (x < 0 || x > Breakout.WIDTH - width) {
dx = -dx;
}
if (y < 0) {
dy = -dy;
}
}
public void reverseY() {
dy = -dy;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public int getWidth() {
return width;
}
public int getHeight() {
return height;
}
public Rectangle getBounds() {
return new Rectangle(x, y, width, height);
}
public boolean intersects(Rectangle r) {
return getBounds().intersects(r);
}
public void paint(Graphics g) {
g.setColor(Color.RED);
g.fillOval(x, y, width, height);
}
}
class Paddle extends JComponent {
private int x;
private int y;
private int width;
private int height;
public Paddle(int x, int y, int width, int height) {
this.x = x;
this.y = y;
this.width = width;
this.height = height;
}
public void moveLeft() {
x -= 5;
if (x < 0) {
x = 0;
}
}
public void moveRight() {
x += 5;
if (x > Breakout.WIDTH - width) {
x = Breakout.WIDTH - width;
}
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public int getWidth() {
return width;
}
public int getHeight() {
return height;
}
public Rectangle getBounds() {
return new Rectangle(x, y, width, height);
}
public void paint(Graphics g) {
g.setColor(Color.BLUE);
g.fillRect(x, y, width, height);
}
}
class Brick extends JComponent {
private int x;
private int y;
private int width;
private int height;
public Brick(int x, int y, int width, int height) {
this.x = x;
this.y = y;
this.width = width;
this.height = height;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public int getWidth() {
return width;
}
public int getHeight() {
return height;
}
public Rectangle getBounds() {
return new Rectangle(x, y, width, height);
}
public void paint(Graphics g) {
g.setColor(Color.GREEN);
g.fillRect(x, y, width, height);
}
}
```
这个代码实现是一个基本的breakout游戏,包含了球、挡板和砖块等基本要素,可以移动挡板和反弹球,打掉砖块并计分。你可以根据需要修改代码来实现更加完整的游戏功能。
希望这些指导对你有帮助,祝你编写成功breakout游戏!
相关推荐
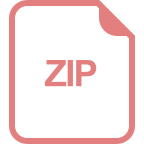
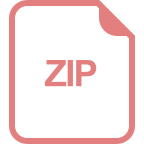














