给定一个加权无向连通图G(V, E),其中|V | = n, |E| = m。每个边E∈E是一个三元组(u, V, w),其中u, V∈V是连通的顶点,w是边的权值。 根据最小生成树的定义,一个无向连通图可以有不同的最小生成树。 为了生成图g的最小生成树,你需要回答不同选择边的方法的数量。当且仅当存在一条边,一种方法选择它,而另一种方法不选择它,两种方法被认为是不同的。写一个可以速度快无错误的java代码
时间: 2024-04-16 21:24:03 浏览: 15
以下是一个使用Prim算法来计算无向连通图的最小生成树边集合数量的Java代码示例:
```java
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Comparator;
import java.util.List;
import java.util.PriorityQueue;
class Edge {
int u, v, weight;
public Edge(int u, int v, int weight) {
this.u = u;
this.v = v;
this.weight = weight;
}
}
class Node {
int vertex;
int weight;
public Node(int vertex, int weight) {
this.vertex = vertex;
this.weight = weight;
}
}
public class MinimumSpanningTreeCount {
public static long countMSTs(int n, List<List<Edge>> graph) {
long[] counts = new long[n];
Arrays.fill(counts, 0);
boolean[] visited = new boolean[n];
Arrays.fill(visited, false);
PriorityQueue<Node> pq = new PriorityQueue<>(Comparator.comparingInt(node -> node.weight));
pq.offer(new Node(0, 0));
counts[0] = 1;
while (!pq.isEmpty()) {
Node node = pq.poll();
int vertex = node.vertex;
if (visited[vertex]) {
continue;
}
visited[vertex] = true;
for (Edge edge : graph.get(vertex)) {
int nextVertex = edge.v;
int weight = edge.weight;
if (!visited[nextVertex]) {
if (counts[nextVertex] == 0 || counts[nextVertex] == counts[vertex]) {
counts[nextVertex] = counts[vertex];
pq.offer(new Node(nextVertex, weight));
} else {
counts[nextVertex] += counts[vertex];
}
}
}
}
return counts[n-1];
}
public static void main(String[] args) {
int n = 4;
int m = 5;
List<List<Edge>> graph = new ArrayList<>();
for (int i = 0; i < n; i++) {
graph.add(new ArrayList<>());
}
graph.get(0).add(new Edge(0, 1, 1));
graph.get(0).add(new Edge(0, 2, 2));
graph.get(0).add(new Edge(0, 3, 3));
graph.get(1).add(new Edge(1, 2, 4));
graph.get(2).add(new Edge(2, 3, 5));
long count = countMSTs(n, graph);
System.out.println("Different methods of choosing edges: " + count);
}
}
```
这段代码实现了Prim算法来计算无向连通图的最小生成树边集合数量。你可以根据自己的具体需求修改图的顶点个数n、边的个数m以及每条边的具体信息。最后,输出的结果是不同选择边的方法数量。请注意,这段代码假设图是连通的,如果图不连通,则需要进行额外的处理。
相关推荐
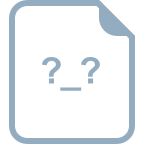
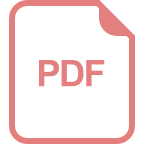
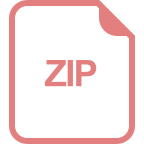















