用c++实现给定一个无向连通图G,采用深度优先搜索方式访问图中节点,并输出。
时间: 2024-06-13 19:08:32 浏览: 15
以下是C++实现给定一个无向连通图G,采用深度优先搜索方式访问图中节点,并输出的代码:
```c++
#include <iostream>
#include <vector>
#include <stack>
using namespace std;
// 定义图的结构体
struct Graph {
int V; // 顶点数
vector<int> *adj; // 邻接表
};
// 初始化图
Graph* initGraph(int V) {
Graph* graph = new Graph;
graph->V = V;
graph->adj = new vector<int>[V];
return graph;
}
// 添加边
void addEdge(Graph* graph, int v, int w) {
graph->adj[v].push_back(w);
graph->adj[w].push_back(v);
}
// 深度优先搜索
void DFS(Graph* graph, int s) {
bool* visited = new bool[graph->V];
for (int i = 0; i < graph->V; i++) {
visited[i] = false;
}
stack<int> stack;
stack.push(s);
while (!stack.empty()) {
s = stack.top();
stack.pop();
if (!visited[s]) {
visited[s] = true;
cout << s << " ";
}
for (auto i = graph->adj[s].begin(); i != graph->adj[s].end(); ++i) {
if (!visited[*i]) {
stack.push(*i);
}
}
}
}
int main() {
Graph* graph = initGraph(5); // 创建一个有5个顶点的图
addEdge(graph, 0, 1);
addEdge(graph, 0, 2);
addEdge(graph, 1, 2);
addEdge(graph, 2, 3);
addEdge(graph, 3, 4);
cout << "深度优先搜索结果:";
DFS(graph, 0); // 从0号顶点开始搜索
cout << endl;
return 0;
}
```
相关推荐
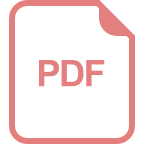
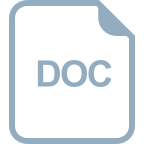
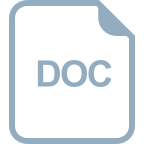














