声明一个基类Animal,有公有整型成员变量age,构成其派生类dog,在其成员函数SetAge(int n)中直接给age赋值
时间: 2024-02-12 18:05:58 浏览: 91
好的,以下是实现代码:
```c++
class Animal {
public:
int age;
};
class Dog : public Animal {
public:
void SetAge(int n) {
age = n;
}
};
```
在这段代码中,我们定义了一个基类Animal,它有一个公共整型成员变量age。然后我们定义了一个派生类Dog,它继承自Animal。在Dog类的成员函数SetAge中,我们直接给age赋值。由于Dog类继承了Animal类的age成员变量,所以可以直接访问和修改它。
相关问题
定义一个基类Animal,有整型成员变量age,构造其派生类dog,在其成员函数SetAge(int n)中直接给age赋值。在主函数中给age赋值后将其输出。编程定义Animal类和派生类dog。
代码如下:
```c++
#include<iostream>
using namespace std;
class Animal{
protected:
int age;
public:
Animal(int age=0):age(age){}
};
class Dog:public Animal{
public:
Dog(int age=0):Animal(age){}
void SetAge(int n){age=n;}
};
int main(){
Dog dog(3);
dog.SetAge(5);
cout<<"Age of the dog is "<<dog.age<<endl;
return 0;
}
```
输出结果为:
```
Age of the dog is 5
```
声明一个基类 Animal,有私有整型成员变量 age,构造其派生类 dog,在其成员函数 SetAge(int n)中直接给 age 赋值,看看会有什么问题,把 age 改为公有成员变量,再在 dog 类用成员函数 ShowAge() 输出变量 age 的值。
首先,我们声明一个基类 `Animal`,其中有一个私有整型成员变量 `age`,表示动物的年龄:
```cpp
class Animal {
private:
int age;
public:
// 构造函数和其他公共方法...
};
```
然后,我们创建一个派生类 `Dog`,试图在 `SetAge` 函数中直接设置 `age` 变量:
```cpp
class Dog : public Animal {
public:
void SetAge(int n) {
age = n; // 直接赋值可能会有问题,因为age是私有的
}
};
```
如果尝试在派生类的这个函数里直接访问 `age`,由于它是私有的,编译器会报错,不允许在派生类的函数内部直接修改基类的私有成员。
接着,我们需要将 `age` 设置为公有,以便于派生类中的其他成员函数可以直接访问:
```cpp
class Animal {
public:
int age;
// 构造函数和其他公共方法...
};
class Dog : public Animal {
public:
void SetAge(int n) {
age = n; // 现在可以正常设置age了,因为它已经是公有成员
}
// 添加ShowAge方法
void ShowAge() {
cout << "Dog's age is: " << age << endl;
}
};
```
现在,在 `Dog` 类中,你可以通过 `ShowAge()` 函数显示 `age` 的值:
```cpp
Dog myDog;
myDog.SetAge(5);
myDog.ShowAge(); // 输出: Dog's age is: 5
```
阅读全文
相关推荐
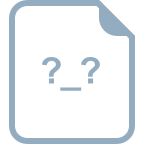
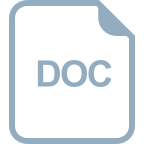
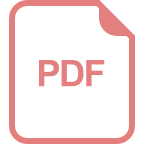













