用keil5写一个用stm32f103c8t6驱动lcd12864程序
时间: 2023-12-01 09:03:46 浏览: 102
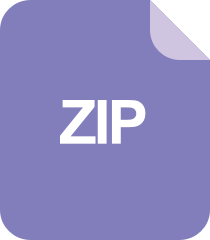
STM32f103C8T6的Lcd12864显示程序
好的,下面是一个简单的用Keil5编写的STM32F103C8T6驱动LCD12864的程序。
首先,需要连接STM32F103C8T6和LCD12864。具体连接方式可以参考LCD12864的数据手册。
然后,创建一个Keil5工程,并选择STM32F103C8T6的芯片型号。
在工程中添加以下文件:
1. lcd12864.h - LCD12864的头文件
2. lcd12864.c - LCD12864的源代码
3. main.c - 主程序
以下是lcd12864.h的代码:
```c
#ifndef __LCD12864_H
#define __LCD12864_H
#include "stm32f10x.h"
#define LCD12864_DATA_PORT GPIOA
#define LCD12864_DATA_OFFSET 0
#define LCD12864_RS_PORT GPIOB
#define LCD12864_RS_PIN GPIO_Pin_0
#define LCD12864_RW_PORT GPIOB
#define LCD12864_RW_PIN GPIO_Pin_1
#define LCD12864_EN_PORT GPIOB
#define LCD12864_EN_PIN GPIO_Pin_2
void LCD12864_Init(void);
void LCD12864_WriteCommand(uint8_t command);
void LCD12864_WriteData(uint8_t data);
void LCD12864_WriteString(char *string);
void LCD12864_SetCursor(uint8_t x, uint8_t y);
void LCD12864_Clear(void);
#endif /* __LCD12864_H */
```
以下是lcd12864.c的代码:
```c
#include "lcd12864.h"
void LCD12864_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_All;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LCD12864_DATA_PORT, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = LCD12864_RS_PIN | LCD12864_RW_PIN | LCD12864_EN_PIN;
GPIO_Init(LCD12864_RS_PORT, &GPIO_InitStructure);
GPIO_ResetBits(LCD12864_RS_PORT, LCD12864_RS_PIN);
GPIO_ResetBits(LCD12864_RW_PORT, LCD12864_RW_PIN);
GPIO_ResetBits(LCD12864_EN_PORT, LCD12864_EN_PIN);
LCD12864_WriteCommand(0x30);
LCD12864_WriteCommand(0x0C);
LCD12864_WriteCommand(0x01);
LCD12864_WriteCommand(0x06);
}
void LCD12864_WriteCommand(uint8_t command)
{
GPIO_ResetBits(LCD12864_RS_PORT, LCD12864_RS_PIN);
GPIO_ResetBits(LCD12864_RW_PORT, LCD12864_RW_PIN);
GPIO_SetBits(LCD12864_EN_PORT, LCD12864_EN_PIN);
LCD12864_DATA_PORT->ODR = command << LCD12864_DATA_OFFSET;
GPIO_ResetBits(LCD12864_EN_PORT, LCD12864_EN_PIN);
}
void LCD12864_WriteData(uint8_t data)
{
GPIO_SetBits(LCD12864_RS_PORT, LCD12864_RS_PIN);
GPIO_ResetBits(LCD12864_RW_PORT, LCD12864_RW_PIN);
GPIO_SetBits(LCD12864_EN_PORT, LCD12864_EN_PIN);
LCD12864_DATA_PORT->ODR = data << LCD12864_DATA_OFFSET;
GPIO_ResetBits(LCD12864_EN_PORT, LCD12864_EN_PIN);
}
void LCD12864_WriteString(char *string)
{
while (*string)
{
LCD12864_WriteData(*string++);
}
}
void LCD12864_SetCursor(uint8_t x, uint8_t y)
{
uint8_t address = y * 0x40 + x;
LCD12864_WriteCommand(0x80 | address);
}
void LCD12864_Clear(void)
{
LCD12864_WriteCommand(0x01);
}
```
以下是main.c的代码:
```c
#include "stm32f10x.h"
#include "lcd12864.h"
int main(void)
{
LCD12864_Init();
LCD12864_WriteString("Hello, world!");
LCD12864_SetCursor(0, 1);
LCD12864_WriteString("This is a test.");
while (1)
{
}
}
```
在main函数中,首先调用LCD12864_Init函数初始化LCD12864,然后使用LCD12864_WriteString函数向LCD12864中写入字符串。使用LCD12864_SetCursor函数可以设置光标的位置。最后使用一个死循环保持程序运行。
这个程序只是一个简单的示例,可以根据需要进行修改和扩展。
阅读全文
相关推荐
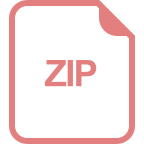
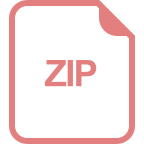
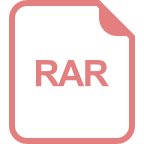
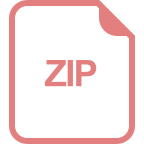
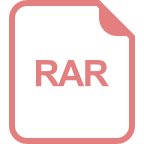

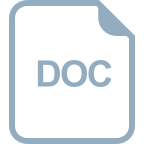







