unity 按角度向其他物体旋转
时间: 2023-06-07 09:08:08 浏览: 92
可以使用Transform类中的Rotate方法来实现。具体实现代码如下:
Transform targetTransform = targetObject.transform;
targetTransform.Rotate(0, angle, 0);
其中,targetObject为需要旋转的物体,而angle为旋转的角度。
相关问题
Unity 点击按钮摄像机围绕物体旋转指定角度
可以使用以下代码实现:
```csharp
public class RotateCamera : MonoBehaviour
{
public Transform target;
public float rotationSpeed = 5.0f;
public float maxRotationAngle = 90.0f;
private Vector3 offset;
void Start()
{
offset = transform.position - target.position;
}
void LateUpdate()
{
float horizontal = Input.GetAxis("Horizontal") * rotationSpeed;
float vertical = Input.GetAxis("Vertical") * rotationSpeed;
transform.RotateAround(target.position, Vector3.up, horizontal);
Vector3 cameraPosition = transform.position;
Vector3 targetPosition = target.position + offset;
Vector3 direction = cameraPosition - targetPosition;
Quaternion rotation = Quaternion.LookRotation(direction, Vector3.up);
float angle = Quaternion.Angle(transform.rotation, rotation);
if (angle < maxRotationAngle)
{
transform.rotation = rotation;
}
}
}
```
这段代码实现了一个摄像机围绕物体旋转的效果,可以通过水平和垂直输入控制旋转方向和速度,同时限制了最大旋转角度。
unity中按钮实现物体旋转
要实现在Unity中通过按钮来控制物体的旋转,可以按照以下步骤进行:
1. 创建一个空物体,将要旋转的物体作为其子物体,以便于后续的旋转操作。
2. 在空物体上添加一个脚本,用于控制旋转。例如:
```csharp
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class RotationController : MonoBehaviour
{
public float rotationSpeed = 10f;
// Update is called once per frame
void Update()
{
transform.Rotate(Vector3.up * rotationSpeed * Time.deltaTime);
}
}
```
这个脚本将使空物体在每一帧旋转一定的角度,即每秒旋转 `rotationSpeed` 度。
3. 在场景中添加一个UI按钮,用于控制旋转。
4. 在按钮上添加一个脚本,用于处理按钮点击事件。例如:
```csharp
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class ButtonController : MonoBehaviour
{
public RotationController rotationController;
private Button button;
// Start is called before the first frame update
void Start()
{
button = GetComponent<Button>();
button.onClick.AddListener(RotateObject);
}
// Update is called once per frame
void Update()
{
}
void RotateObject()
{
rotationController.rotationSpeed *= -1;
}
}
```
这个脚本将使按钮在被点击时,将旋转速度取反,即实现了控制物体旋转的效果。
注意:在这个示例中,我们是通过控制空物体的旋转来实现控制物体旋转的,因此需要将要旋转的物体作为空物体的子物体。如果你想直接控制要旋转的物体,可以将脚本中的 `transform` 改为要旋转的物体的 `transform`。
阅读全文
相关推荐
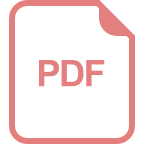
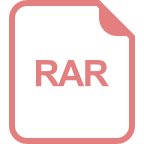
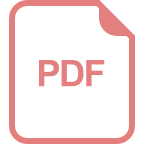













