nn.MaxPool2d()
时间: 2023-12-02 11:42:40 浏览: 75
nn.MaxPool2d() 是 PyTorch 中用于创建最大池化层的函数。最大池化层通常用于减小图像或特征图的空间尺寸,同时保留重要的特征。该函数的常用参数包括 kernel_size(池化窗口大小)、stride(步长)、padding(填充)等。以下是一个示例:
```python
import torch.nn as nn
# 创建一个最大池化层
max_pool_layer = nn.MaxPool2d(kernel_size=2, stride=2)
# 假设有一个输入张量 input_tensor,其形状为 [batch_size, channels, height, width]
# 经过最大池化层后,输出张量的形状为 [batch_size, channels, height/2, width/2]
output_tensor = max_pool_layer(input_tensor)
```
相关问题
nn.MaxPool2d
nn.MaxPool2d is a function in PyTorch's nn module that performs max pooling operation on 2D inputs (images). Max pooling is a type of pooling operation in convolutional neural networks (CNNs) that reduces the spatial dimensions of the input while preserving the most important features.
The function takes several arguments, including the kernel size of the pooling window, stride, padding, and dilation. The input tensor is divided into non-overlapping rectangular blocks of size kernel_size, and the maximum value in each block is taken as the output. The stride parameter controls the spacing between the blocks, while padding adds extra values around the input tensor to ensure that the kernel can be applied to the borders. Dilation controls the spacing between the values within the kernel.
Here's an example of how to use nn.MaxPool2d:
```
import torch.nn as nn
maxpool = nn.MaxPool2d(kernel_size=2, stride=2)
input_tensor = torch.randn(1, 3, 28, 28) # batch size, number of channels, height, width
output_tensor = maxpool(input_tensor)
```
In this example, we create a max pooling layer with a kernel size of 2 and a stride of 2. We then apply this layer to an input tensor of size (1, 3, 28, 28), which represents a batch of 1 RGB image with a height and width of 28 pixels. The output tensor will have a size of (1, 3, 14, 14), with the height and width reduced by half due to the max pooling operation.
nn.maxpool2d
`nn.MaxPool2d` 是 PyTorch 中的一个二维最大池化层,用于在卷积神经网络中减小特征图的空间大小。它将输入张量沿着指定的维度进行滑动窗口最大池化操作,返回池化后的张量。它的输入是一个四维张量,包括:`batch_size`、`channels`、`height` 和 `width`。具体来说,它的参数包括:
- `kernel_size`:池化操作的窗口大小。
- `stride`:池化操作的步幅大小。
- `padding`:在输入边缘添加额外的零值填充。
- `dilation`:输入中元素之间的间距。
- `return_indices`:是否返回池化操作所选取的最大元素的索引。
- `ceil_mode`:当为 True 时,将使用 ceil 而不是 floor 计算输出形状。
示例代码:
```python
import torch.nn as nn
maxpool = nn.MaxPool2d(kernel_size=2, stride=2)
x = torch.randn(1, 16, 28, 28)
output = maxpool(x)
```
在上面的例子中,输入张量 `x` 的大小为 `[1, 16, 28, 28]`,即一个 batch 大小为1,通道数为16,高度和宽度都为28的四维张量。`kernel_size` 和 `stride` 都设置为2,表示在高度和宽度上每隔2个元素进行一次最大池化操作。最终输出的张量大小为 `[1, 16, 14, 14]`,即每个通道的高度和宽度都缩小了一半。
阅读全文
相关推荐
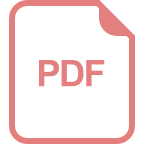
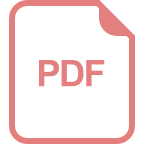
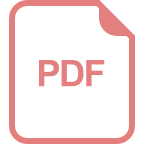













